Using Backed Enums with Laravel Authorization
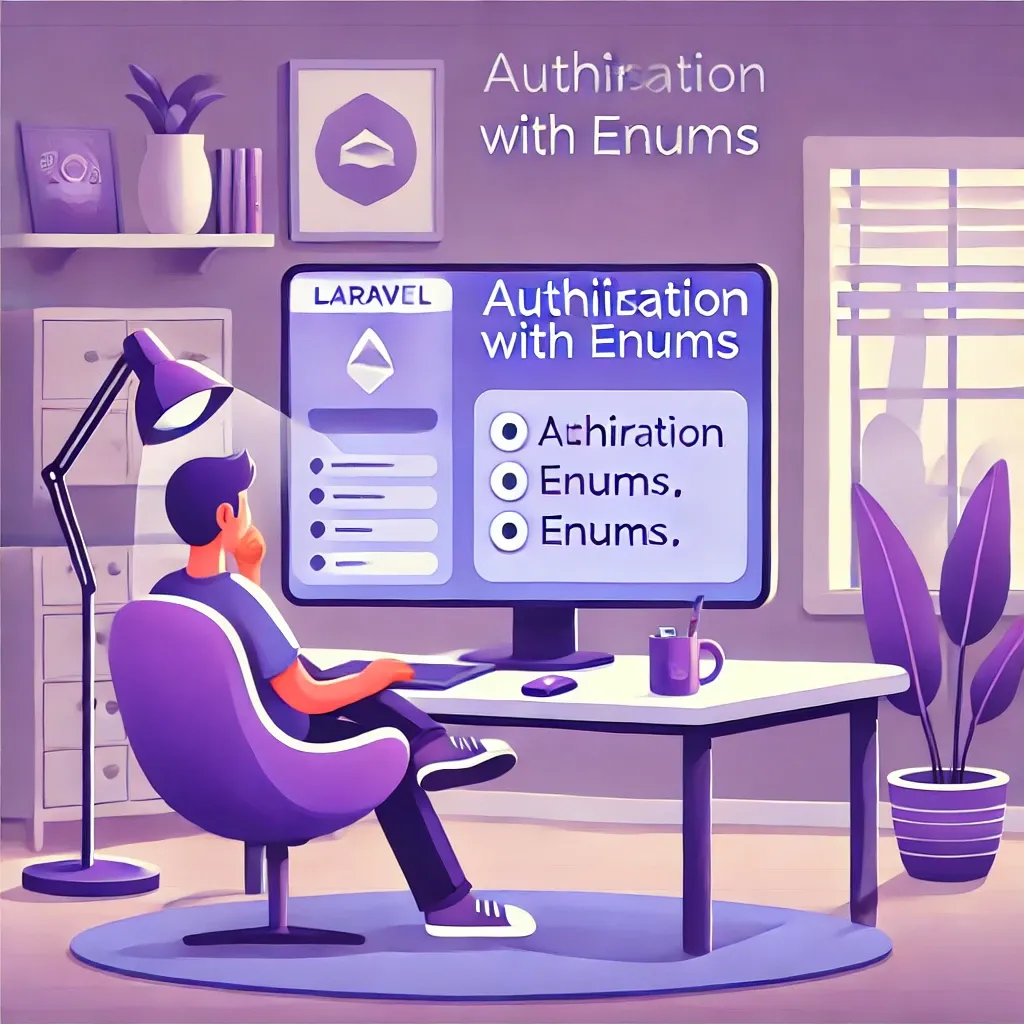
Simplify authorization in Laravel with direct enum support. The updated AuthorizesRequests trait now accepts backed enums without requiring the ->value accessor.
Basic Usage
Define and use your permission enums directly:
enum DashboardPermission: string
{
case VIEW = 'dashboard.view';
case EDIT = 'dashboard.edit';
case MANAGE = 'dashboard.manage';
}
public function index(): Response
{
$this->authorize(DashboardPermission::VIEW);
return view('dashboard.index');
}
Real-World Example
Here's how to implement a comprehensive permission system using enums:
enum UserPermission: string
{
case VIEW_USERS = 'users.view';
case CREATE_USERS = 'users.create';
case EDIT_USERS = 'users.edit';
case DELETE_USERS = 'users.delete';
}
class UserController extends Controller
{
public function index()
{
$this->authorize(UserPermission::VIEW_USERS);
return User::paginate();
}
public function store(UserRequest $request)
{
$this->authorize(UserPermission::CREATE_USERS);
$user = User::create($request->validated());
return redirect()
->route('users.show', $user)
->with('success', 'User created successfully');
}
public function update(UserRequest $request, User $user)
{
$this->authorize(UserPermission::EDIT_USERS);
$user->update($request->validated());
return response()->json([
'message' => 'User updated successfully',
'user' => $user
]);
}
public function destroy(User $user)
{
$this->authorize(UserPermission::DELETE_USERS);
$user->delete();
return redirect()
->route('users.index')
->with('success', 'User deleted successfully');
}
}
The direct enum support makes your authorization code cleaner and more maintainable.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!