Using Backed Enums with Bus Chain's onQueue Method
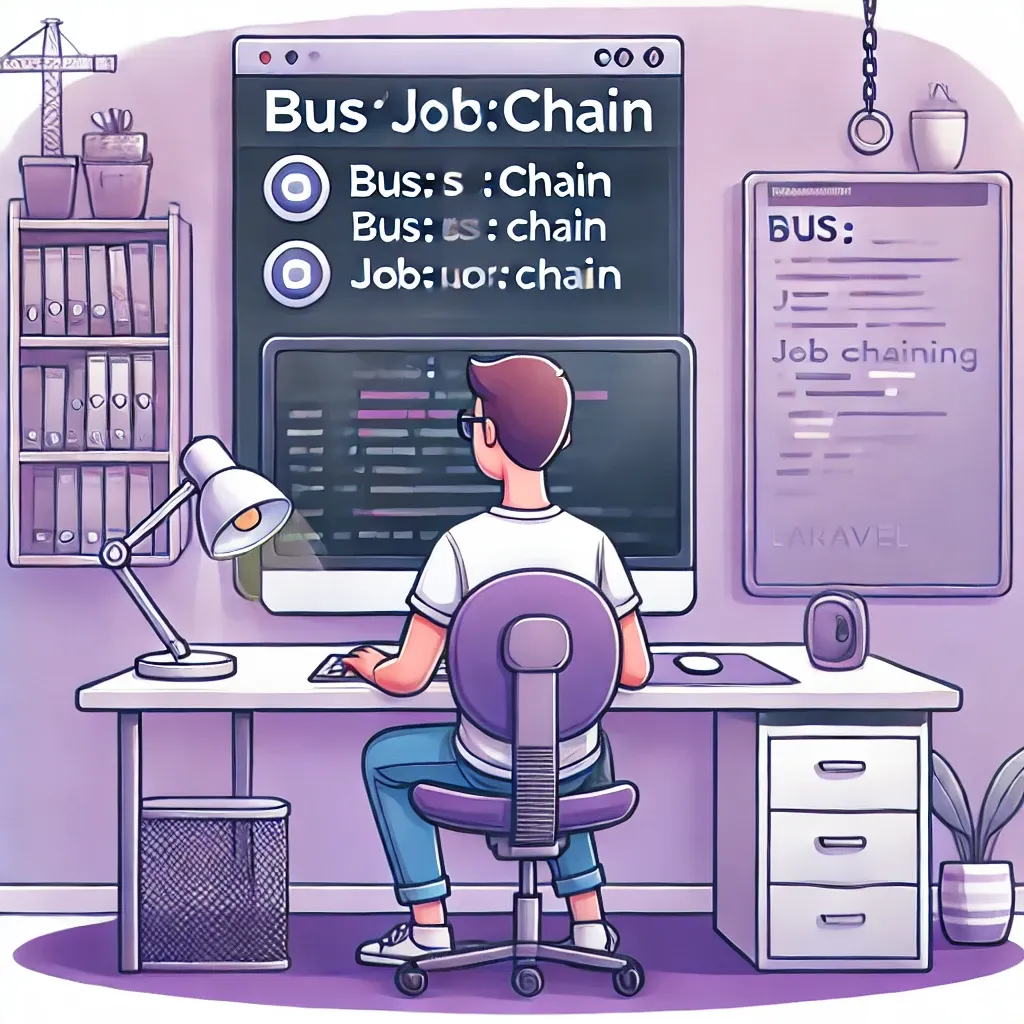
Learn how to streamline your queue handling by directly using backed enums with Laravel's Bus chain onQueue method, removing the need for explicit value access.
Basic Usage
Here's the clean, new way to use enums with queue chains:
// The new, cleaner way
Bus::chain($jobs)
->onQueue(QueueName::long)
->dispatch();
Real-World Example
Here's a practical implementation using queue chains with enums:
enum QueueName: string
{
case High = 'high-priority';
case Normal = 'default';
case Long = 'long-running';
case Background = 'background-tasks';
}
class OrderProcessor
{
public function processLargeOrder(Order $order)
{
Bus::chain([
new ValidateOrderJob($order),
new ProcessPaymentJob($order),
new UpdateInventoryJob($order),
new SendNotificationsJob($order)
])
->onQueue(QueueName::Long)
->dispatch();
}
public function processUrgentOrder(Order $order)
{
Bus::chain([
new ValidateOrderJob($order),
new ExpressProcessingJob($order),
new PriorityNotificationJob($order)
])
->onQueue(QueueName::High)
->catch(function (Throwable $e) use ($order) {
report("Failed processing urgent order {$order->id}: {$e->getMessage()}");
})
->dispatch();
}
}
// Usage in controller
class OrderController extends Controller
{
public function store(OrderRequest $request, OrderProcessor $processor)
{
$order = Order::create($request->validated());
if ($request->is_urgent) {
$processor->processUrgentOrder($order);
} else {
$processor->processLargeOrder($order);
}
return response()->json([
'message' => 'Order processing started',
'order_id' => $order->id
]);
}
}
This new support for backed enums makes your code cleaner and more type-safe when working with queue chains.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!