URL Validation in Laravel with isUrl Method
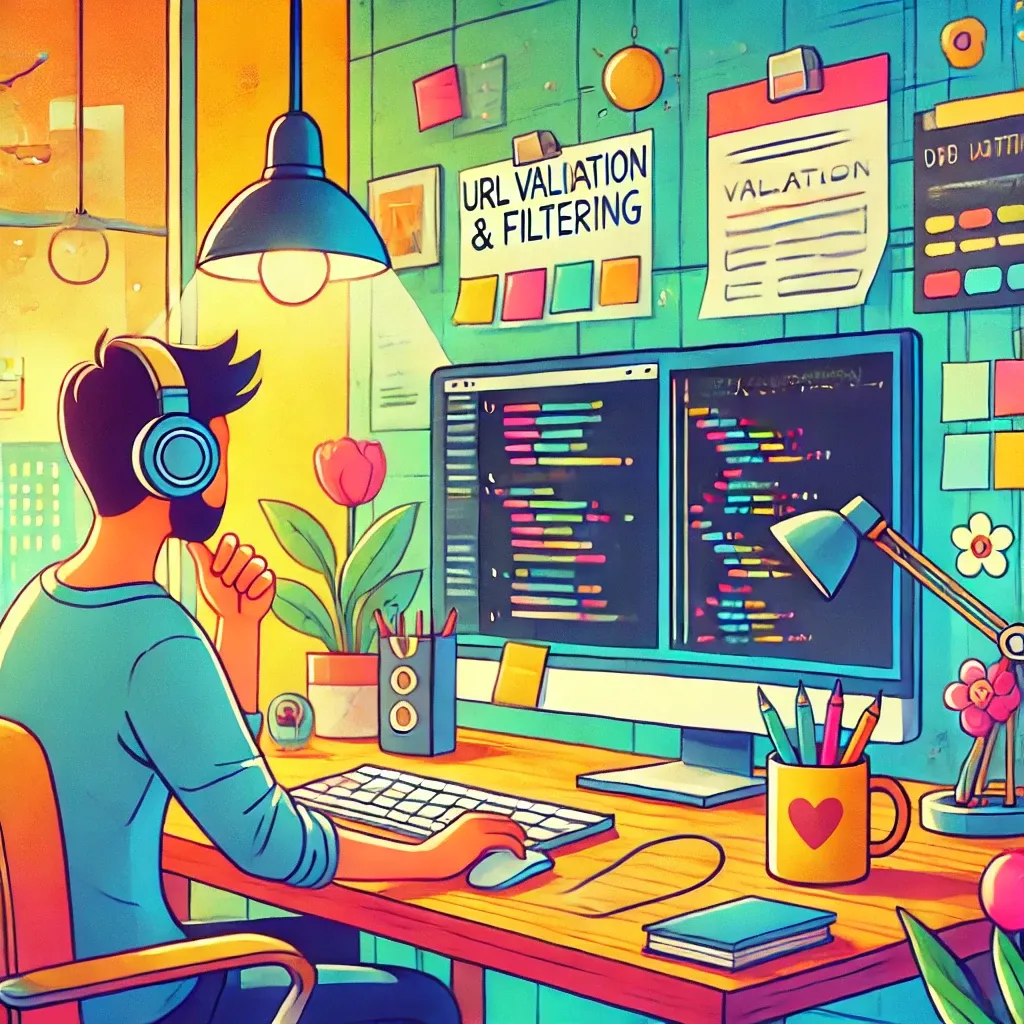
Need to validate URLs in your Laravel application? The Str::isUrl method provides a simple and flexible way to check if strings are valid URLs.
Basic Usage
Validate URLs with or without protocol restrictions:
use Illuminate\Support\Str;
// Basic URL validation
$isUrl = Str::isUrl('http://example.com'); // true
$isUrl = Str::isUrl('laravel'); // false
// With protocol restrictions
$isUrl = Str::isUrl('http://example.com', ['http', 'https']);
Real-World Example
Here's how you might use it in a link management system:
class LinkValidator
{
public function validateSocialLinks(array $links)
{
return collect($links)->filter(function ($url, $platform) {
return match ($platform) {
'twitter' => Str::isUrl($url, ['https']),
'github' => Str::isUrl($url, ['https']),
'website' => Str::isUrl($url, ['http', 'https']),
default => Str::isUrl($url)
};
});
}
public function validateSecureUrls(array $urls)
{
return collect($urls)
->filter(fn($url) => Str::isUrl($url, ['https']))
->values();
}
public function sanitizeUserSubmittedLinks(array $links)
{
return array_filter($links, function ($link) {
// Only allow http/https URLs
if (!Str::isUrl($link, ['http', 'https'])) {
return false;
}
// Additional validation logic here
return true;
});
}
}
// Usage
$validator = new LinkValidator();
$socialLinks = [
'twitter' => 'https://twitter.com/user',
'website' => 'http://example.com',
'invalid' => 'not-a-url'
];
$validLinks = $validator->validateSocialLinks($socialLinks);
The isUrl method makes it easy to validate URLs while maintaining control over accepted protocols.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!