Updating JSON Columns in Laravel: A Quick Guide
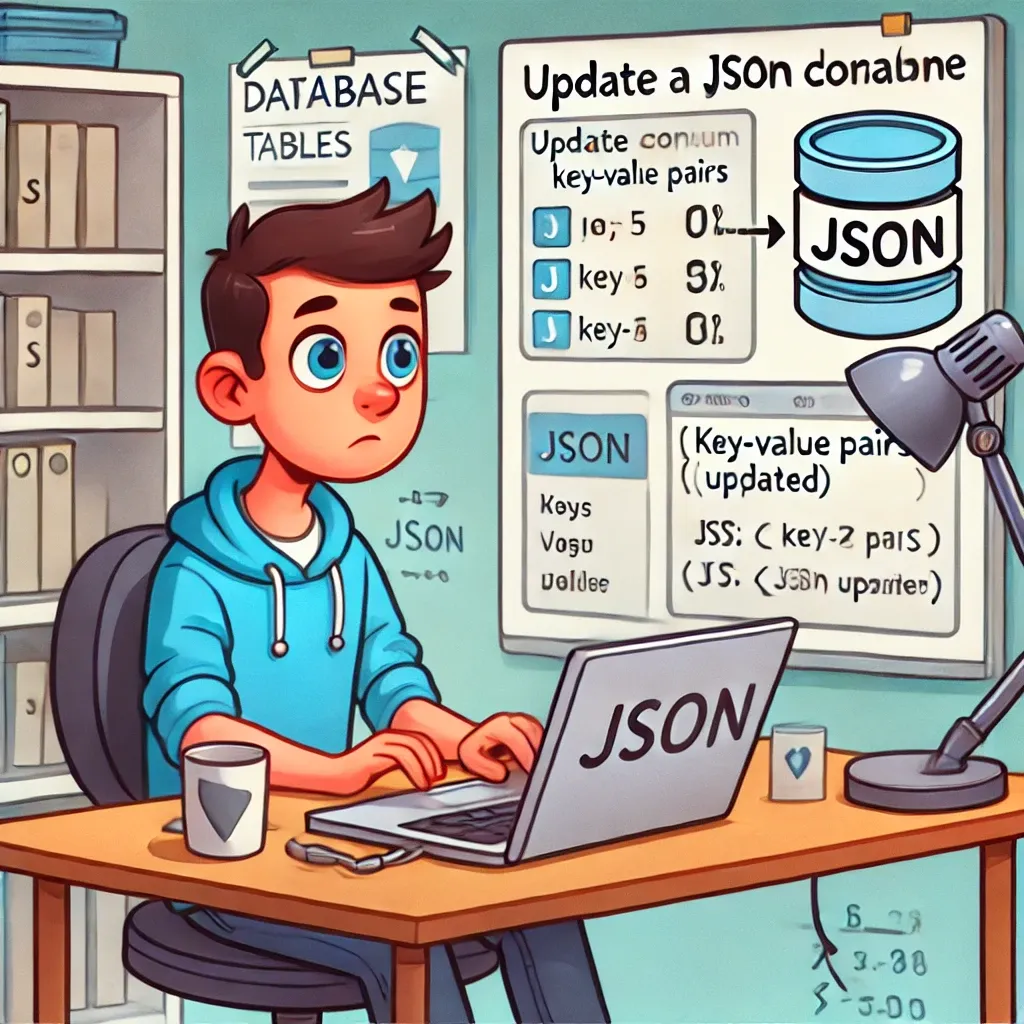
Ever needed to update just a part of a JSON column in your database? Laravel makes this a breeze with its intuitive syntax. Let's dive into how you can easily modify JSON data in your database queries.
The JSON Arrow Syntax
Laravel uses the ->
syntax to update specific keys in a JSON object. It's like saying, "Hey database, go into this JSON column and change this particular value."
Basic Usage
Here's a simple example:
$affected = DB::table('users')
->where('id', 1)
->update(['options->enabled' => true]);
What's happening here?
- We're targeting the 'users' table
- We're looking for a user with id 1
- We're updating the 'enabled' key in the 'options' JSON column
- We're setting its value to true
Database Support
This nifty feature works on:
- MariaDB 10.3+
- MySQL 5.7+
- PostgreSQL 9.5+
Make sure your database version supports this before you start coding away!
Real-World Example: User Preferences
Let's say you have a user preferences system where users can toggle various settings. Here's how you might update a specific preference:
use Illuminate\Support\Facades\DB;
class UserPreferencesController extends Controller
{
public function toggleNotifications($userId, $notificationType, $enabled)
{
$affected = DB::table('users')
->where('id', $userId)
->update(["preferences->notifications->$notificationType" => $enabled]);
return $affected ? 'Preference updated' : 'Update failed';
}
}
In this example:
- We're updating a nested JSON structure (preferences -> notifications -> specific type)
- We're toggling a boolean value for a specific notification type
- We're returning a message based on whether the update was successful
This approach lets you handle complex user preferences without needing separate tables for each setting.
Using Laravel's JSON column update feature, you can easily manage complex data structures in your database. It's perfect for user settings, configuration options, or any scenario where you need flexible, schemaless data storage.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!