Unraveling Laravel's Request Host Methods: A Comprehensive Guide
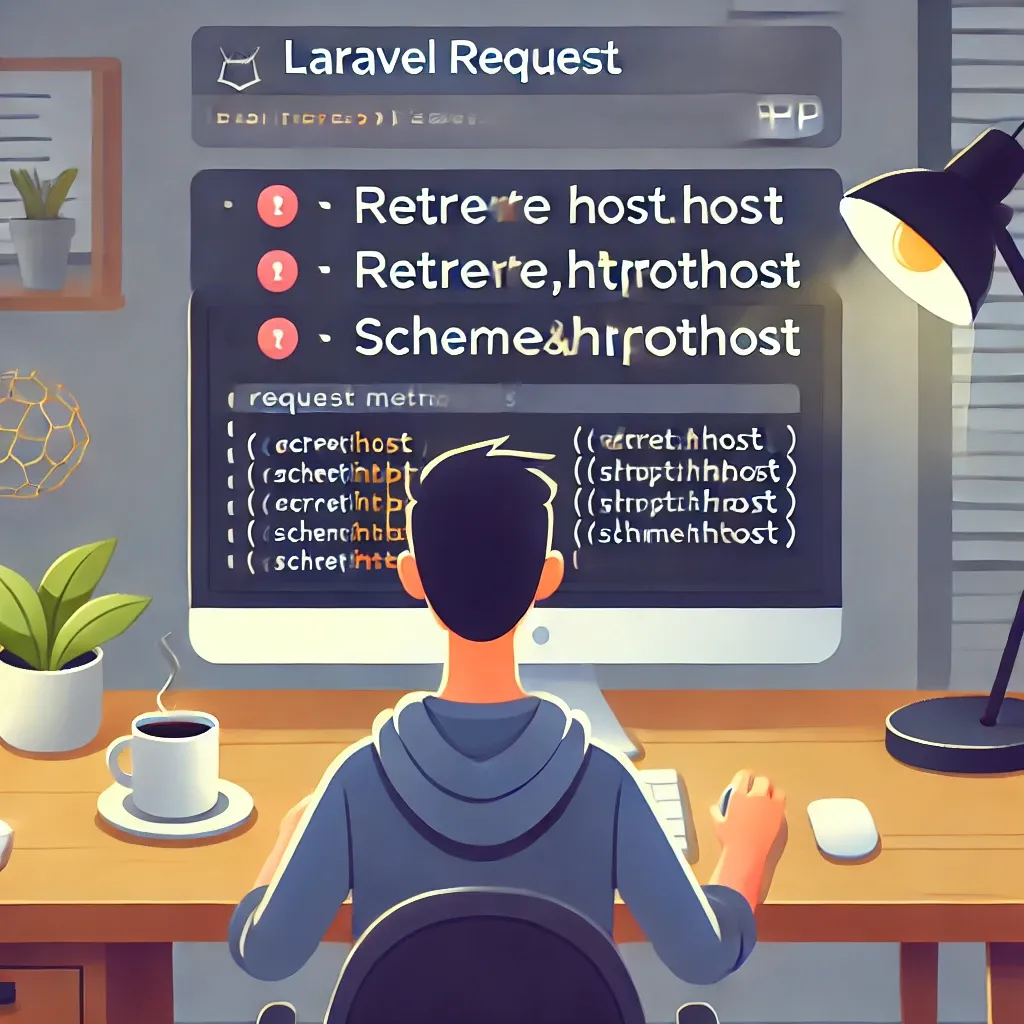
When building web applications, understanding and manipulating request information is crucial. Laravel provides several convenient methods to retrieve the host of incoming requests. In this post, we'll explore the host()
, httpHost()
, and schemeAndHttpHost()
methods, their differences, and when to use each one.
Understanding Request Host Methods
Laravel's Request object provides three methods to retrieve the host of an incoming request:
host()
: Returns the host name.httpHost()
: Returns the HTTP host name and port number.schemeAndHttpHost()
: Returns the full URL scheme and HTTP host.
Let's dive deeper into each method:
1. The host() Method
The host()
method returns the host name of the request:
$host = $request->host();
2. The httpHost() Method
The httpHost()
method returns the HTTP host name and port number:
$httpHost = $request->httpHost();
3. The schemeAndHttpHost() Method
The schemeAndHttpHost()
method returns the full URL scheme and HTTP host:
$fullHost = $request->schemeAndHttpHost();
Real-Life Example
Let's consider a scenario where we're building a multi-tenant application that needs to handle requests from different subdomains. We'll use these methods to determine which tenant is being accessed and to generate full URLs for each tenant.
Here's how we might use these methods in a middleware:
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class DetermineTenant
{
public function handle(Request $request, Closure $next)
{
$host = $request->host();
$tenant = Tenant::where('subdomain', $host)->firstOrFail();
// Store the tenant in the request for later use
$request->merge(['tenant' => $tenant]);
// Generate URLs for the tenant
$tenantUrls = [
'dashboard' => $request->schemeAndHttpHost() . '/dashboard',
'api' => $request->schemeAndHttpHost() . '/api/v1',
];
$request->merge(['tenantUrls' => $tenantUrls]);
return $next($request);
}
}
Now, let's see how this might work in practice:
// Assuming the request is coming from 'client1.ourapp.com'
// In a controller action
public function dashboard(Request $request)
{
$tenant = $request->tenant;
$dashboardUrl = $request->tenantUrls['dashboard'];
return view('dashboard', compact('tenant', 'dashboardUrl'));
}
The output might look something like this:
// GET http://client1.ourapp.com/dashboard
{
"view": "dashboard",
"data": {
"tenant": {
"id": 1,
"name": "Client 1",
"subdomain": "client1"
},
"dashboardUrl": "http://client1.ourapp.com/dashboard"
}
}
In this example:
- We use
host()
to determine the tenant based on the subdomain. - We use
schemeAndHttpHost()
to generate full URLs for the tenant's dashboard and API.
This approach allows us to handle multiple tenants on different subdomains while generating the correct URLs for each tenant.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!