Transforming Collections into Queries: Laravel's toQuery Method
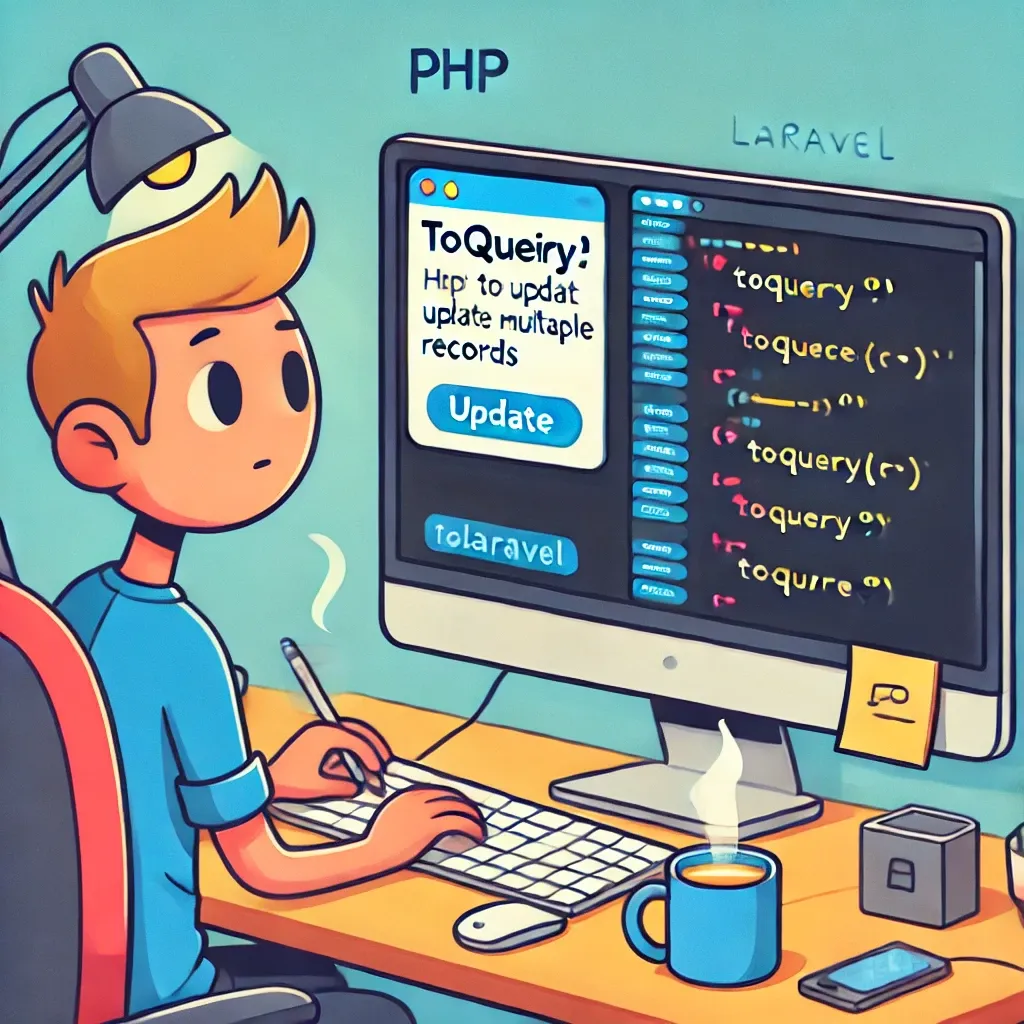
Laravel's Eloquent ORM is known for its powerful and expressive API. One of its lesser-known but incredibly useful features is the toQuery
method available on collections. This method allows you to convert a collection of models back into a query builder instance, opening up a world of possibilities for efficient data manipulation.
Understanding toQuery
The toQuery
method takes a collection of Eloquent models and returns an Eloquent query builder instance. This query builder includes a whereIn
constraint on the primary keys of the models in the collection.
Basic Usage
Here's a simple example of how to use toQuery
:
$users = User::where('status', 'active')->get();
$userQuery = $users->toQuery();
Now, $userQuery
is a query builder instance that you can further modify or execute.
Practical Applications
1. Bulk Updates
One of the most common use cases for toQuery
is performing bulk updates on a subset of models:
$vipUsers = User::where('status', 'VIP')->get();
$vipUsers->toQuery()->update(['discount' => 20]);
This operation is more efficient than looping through the collection and updating each model individually, especially for large datasets.
2. Further Filtering
You can use toQuery
to apply additional filters to a collection you've already retrieved:
$activeUsers = User::where('status', 'active')->get();
$recentActiveUsers = $activeUsers->toQuery()
->where('last_login', '>=', now()->subDays(7))
->get();
3. Aggregations
toQuery
allows you to perform database-level aggregations on a collection:
$orders = Order::where('status', 'completed')->get();
$totalRevenue = $orders->toQuery()->sum('total_amount');
4. Joining with Other Tables
You can use toQuery
to join your collection with other tables:
$products = Product::where('category', 'electronics')->get();
$productsWithSuppliers = $products->toQuery()
->join('suppliers', 'products.supplier_id', '=', 'suppliers.id')
->select('products.*', 'suppliers.name as supplier_name')
->get();
Real-World Scenario: Order Processing System
Imagine you're building an order processing system. You've retrieved a collection of orders that need to be marked as shipped:
$pendingOrders = Order::where('status', 'pending')
->where('shipping_date', '<=', now())
->get();
// Mark orders as shipped and update shipping information
$pendingOrders->toQuery()->update([
'status' => 'shipped',
'shipped_at' => now(),
]);
// Now, let's notify customers about their shipped orders
$shippedOrderIds = $pendingOrders->pluck('id');
Notification::send(
User::whereHas('orders', function ($query) use ($shippedOrderIds) {
$query->whereIn('id', $shippedOrderIds);
})->get(),
new OrderShippedNotification()
);
In this example, we use toQuery
to efficiently update all pending orders in a single query. Then, we use the original collection to notify customers about their shipped orders.
Laravel's toQuery
method bridges the gap between collections and query builders, allowing for flexible and efficient data operations. By converting collections back to query builders, you can leverage the full power of Eloquent's querying capabilities, even after you've already retrieved data from the database. This can lead to more efficient and expressive code in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!