Transform URL Handling with Laravel's Uri Class
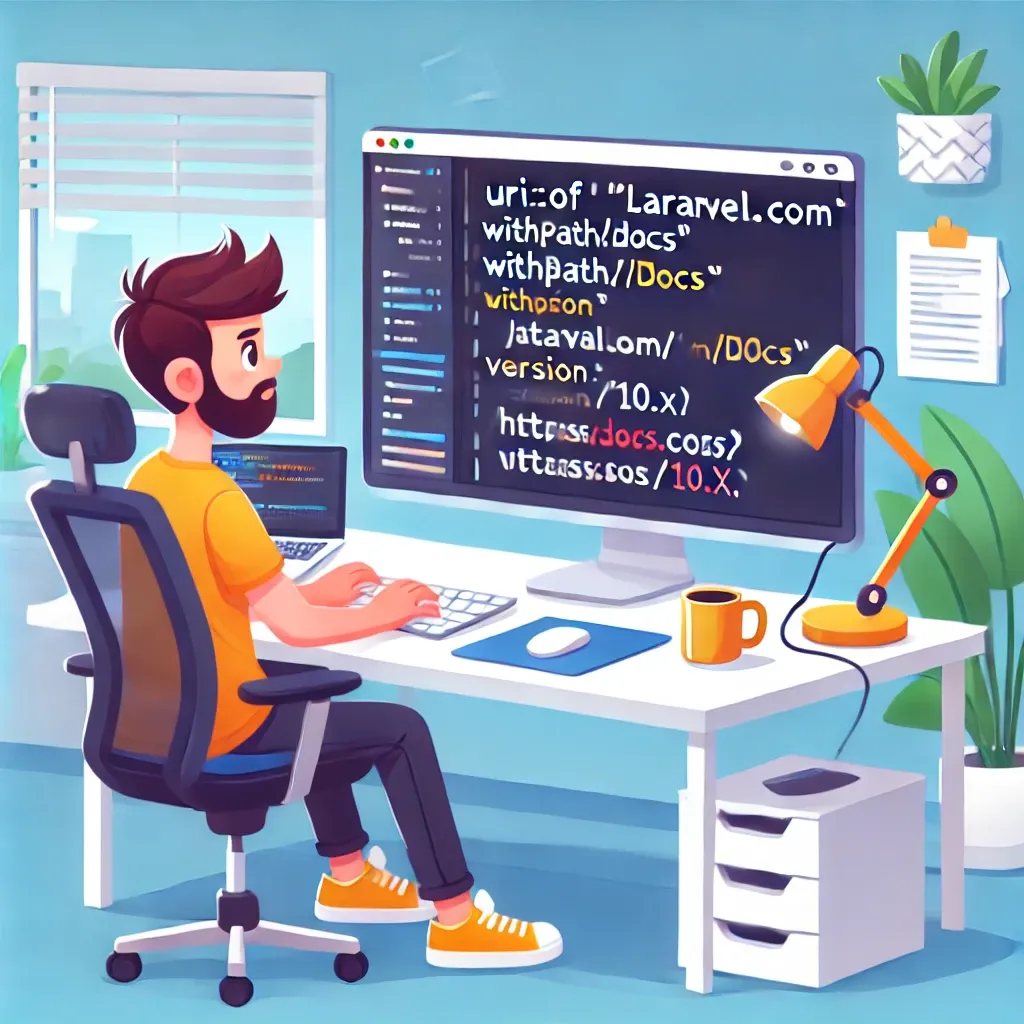
Transform the way you work with URLs in Laravel using the new Uri class. This powerful abstraction makes URI manipulation more intuitive and maintainable than ever.
Basic Usage
Create and manipulate URIs with a fluent interface:
$uri = Uri::of('https://laravel.com')
->withQuery(['name' => 'Taylor'])
->withPath('/docs/installation')
->withFragment('hello-world');
// https://laravel.com/docs/installation?name=Taylor#hello-world
Real-World Example
Here's how you might use it in a link management system:
class LinkManager
{
public function createTrackableLink(string $destination, array $params = [])
{
$uri = Uri::of($destination)
->withQuery(array_merge(
$params,
['utm_source' => 'newsletter', 'ref' => uniqid()]
));
return Link::create([
'original_url' => $destination,
'tracked_url' => (string) $uri,
'params' => $uri->query()->decode()
]);
}
public function generateSocialShareLinks(Post $post)
{
$url = Uri::route('posts.show', $post);
return [
'twitter' => Uri::of('https://twitter.com/intent/tweet')
->withQuery([
'url' => (string) $url,
'text' => $post->title
]),
'facebook' => Uri::of('https://www.facebook.com/sharer/sharer.php')
->withQuery(['u' => (string) $url]),
'linkedin' => Uri::of('https://www.linkedin.com/shareArticle')
->withQuery([
'url' => (string) $url,
'title' => $post->title,
'mini' => true
])
];
}
}
The Uri class provides a clean, fluent interface for all your URL manipulation needs.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!