Transform HTTP Response Data with Laravel's Fluent Method
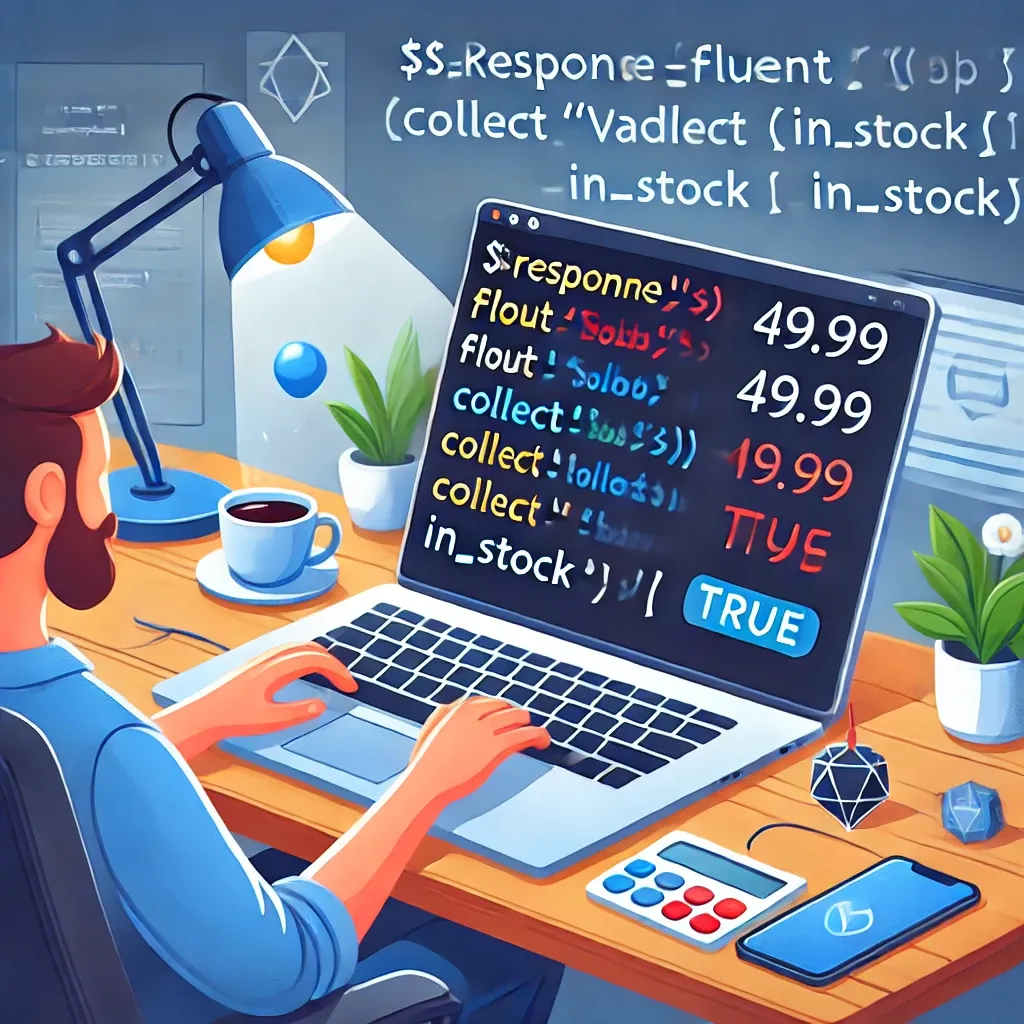
Transform HTTP response data effortlessly with Laravel's new fluent() method. This addition to the HTTP Client brings type-safe access to your API responses.
Basic Usage
Convert your HTTP responses to fluent instances:
$response = Http::get('https://api.example.com/products/1')->fluent();
$price = $response->float('price'); // 420.69
$colors = $response->collect('colors'); // Collection(['red', 'blue'])
$onSale = $response->boolean('on_sale'); // true
$stock = $response->integer('current_stock'); // 69
Real-World Example
Here's how you might use it in a product service:
class ExternalProductService
{
public function fetchProductDetails(string $productId)
{
$response = Http::get("https://api.example.com/products/{$productId}")
->throw()
->fluent();
return new ProductDetails([
'price' => $response->float('price'),
'stock_level' => $response->integer('current_stock'),
'is_available' => $response->boolean('in_stock'),
'variants' => $response->collect('variants')->map(function ($variant) {
return [
'sku' => $variant['sku'],
'quantity' => (int) $variant['quantity'],
'price' => (float) $variant['price']
];
}),
'metadata' => $response->collect('metadata')->toArray(),
'last_updated' => $response->date('updated_at')
]);
}
public function updateInventory(string $productId, int $quantity)
{
$response = Http::put("https://api.example.com/inventory/{$productId}", [
'quantity' => $quantity
])->fluent();
return [
'success' => $response->boolean('success'),
'new_quantity' => $response->integer('current_stock'),
'timestamp' => $response->date('processed_at')
];
}
}
The fluent() method provides a clean, type-safe way to work with API response data.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!