Transform Array Values into Enum Instances with Laravel's mapInto Method
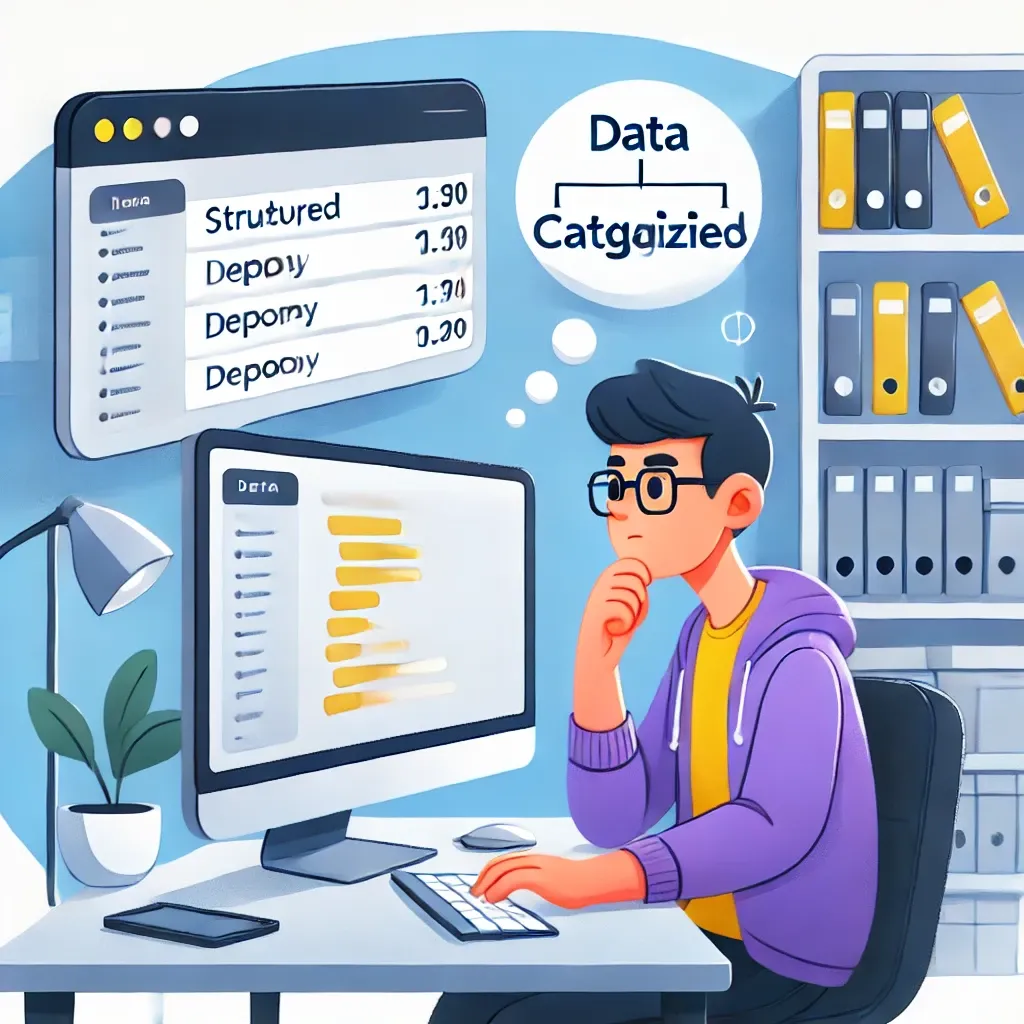
Ever struggled with transforming array values into enum instances in Laravel? The enhanced Collection::mapInto method now offers first-class support for PHP 8.1 Enums, making enum mapping elegant and straightforward.
When PHP 8.1 introduced Enums as a first-class feature, it gave developers a powerful way to define a set of possible values for a type. Laravel has fully embraced this feature, and the Collection::mapInto method now provides a clean solution for transforming raw values into proper enum instances - especially useful when handling user input that needs conversion to type-safe enum cases.
Let's see how it works:
public function store(Request $request)
{
$request->validate([
'features' => ['array'],
'features.*' => [new Enum(Feature::class)],
]);
$features = $request
->collect('features')
->mapInto(Feature::class);
if ($features->contains(Feature::DarkMode)) {
// Enable dark mode functionality
}
}
Real-World Example
Here's a more comprehensive example showing how you might use this feature in a user preferences system:
<?php
namespace App\Enums;
enum Feature: string
{
case DarkMode = 'dark_mode';
case BetaFeatures = 'beta_features';
case AdvancedAnalytics = 'advanced_analytics';
case CustomThemes = 'custom_themes';
public function isEnterprise(): bool
{
return in_array($this, [
self::AdvancedAnalytics,
self::CustomThemes
]);
}
}
class UserPreferencesController extends Controller
{
public function update(Request $request)
{
$request->validate([
'features' => ['array'],
'features.*' => [new Enum(Feature::class)],
]);
// Transform string values into Feature enum instances
$features = $request
->collect('features')
->mapInto(Feature::class);
// Check for enterprise features
$hasEnterpriseFeatures = $features
->filter(fn (Feature $feature) => $feature->isEnterprise())
->isNotEmpty();
if ($hasEnterpriseFeatures && !$request->user()->hasEnterpriseAccess()) {
return back()->withErrors([
'features' => 'Some selected features require an enterprise subscription'
]);
}
// Store the selected features
$request->user()->preferences()->update([
'features' => $features->all()
]);
return redirect()->route('user.preferences.show')
->with('status', 'Preferences updated successfully');
}
}
Behind the scenes, the mapInto
method creates each enum instance by passing the original value to the enum class. For string-backed enums, this process converts string values like 'dark_mode'
to the corresponding enum case Feature::DarkMode
.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!