The Last Line of Defense: Mastering Laravel's Fallback Routes
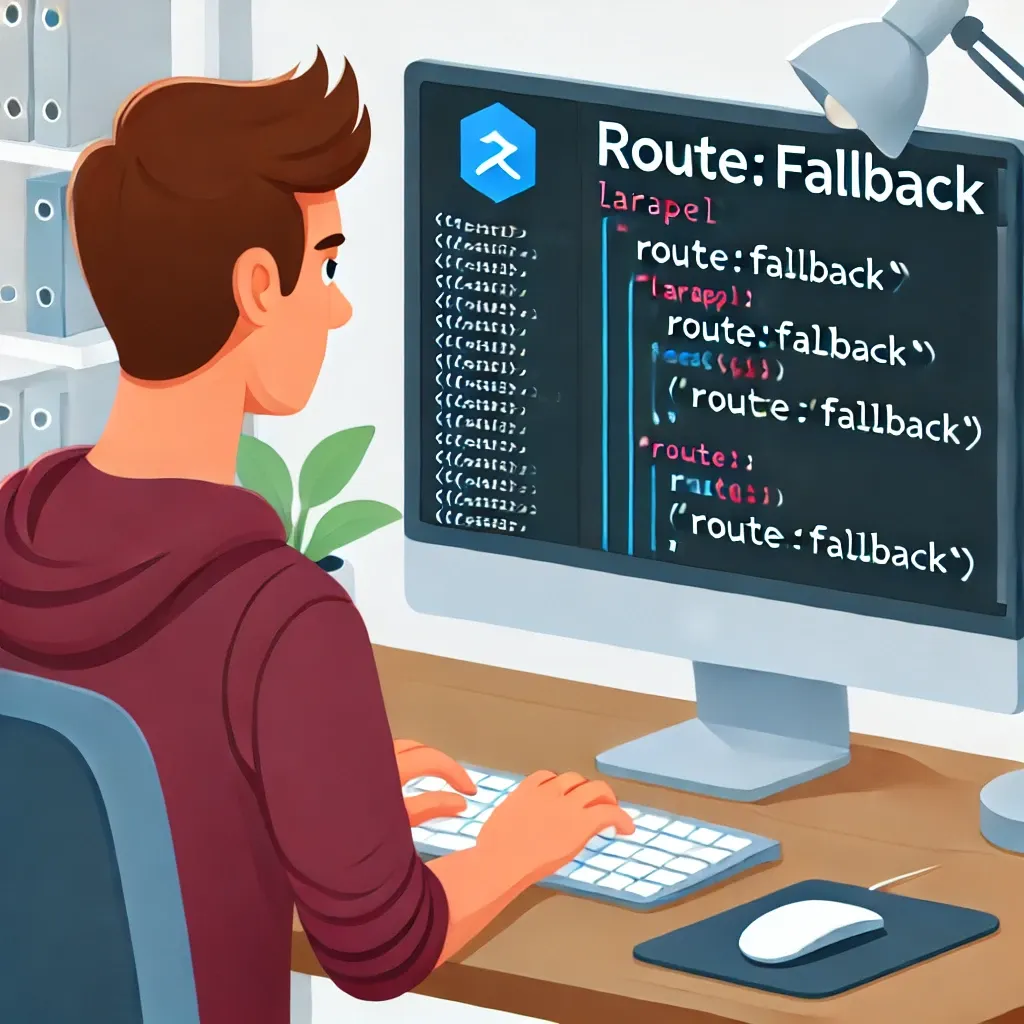
Ever wondered what happens when a user hits a URL that doesn't exist in your Laravel app? Enter the Route::fallback
method - your secret weapon for handling those pesky 404s with style. Let's dive in!
What's Route::fallback?
Route::fallback
is Laravel's way of saying, "If all else fails, do this." It's the route of last resort, catching any requests that don't match your defined routes.
Basic Usage
Here's how you might use it:
Route::fallback(function () {
return view('errors.404');
});
Real-World Example: Smart 404 Handler
Let's create a fallback route that does more than just show an error:
use Illuminate\Support\Facades\Route;
use App\Models\Page;
use Illuminate\Support\Str;
Route::fallback(function () {
$path = request()->path();
// Try to find a similar page
$similarPage = Page::where('slug', 'like', '%' . Str::slug(Str::words($path, 1, '')) . '%')
->first();
if ($similarPage) {
return redirect()->to($similarPage->slug)
->with('message', 'The page you looked for was not found, but you might be interested in this.');
}
// Log the miss
\Log::channel('404s')->info('Missing page: ' . $path);
// Return custom 404 view with search functionality
return response()
->view('errors.404', ['searched' => $path], 404)
->header('Cache-Control', 'no-cache, must-revalidate');
});
In this example:
- We try to find a similar page based on the URL
- If found, we redirect with a helpful message
- We log all 404s for later analysis
- We return a custom 404 page with the path they were looking for
Pro Tip: Fallback API Routes
Don't forget about API routes! You can define a separate fallback for your API:
Route::fallback(function(){
return response()->json([
'message' => 'Page Not Found. If error persists, contact info@website.com'
], 404);
})->name('api.fallback.404');
By leveraging Route::fallback
, you're not just handling errors – you're creating opportunities to guide users, gather insights, and polish your app's user experience. It's the safety net that keeps your users from falling through the cracks!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!