Testing Number Ranges in Laravel with countBetween
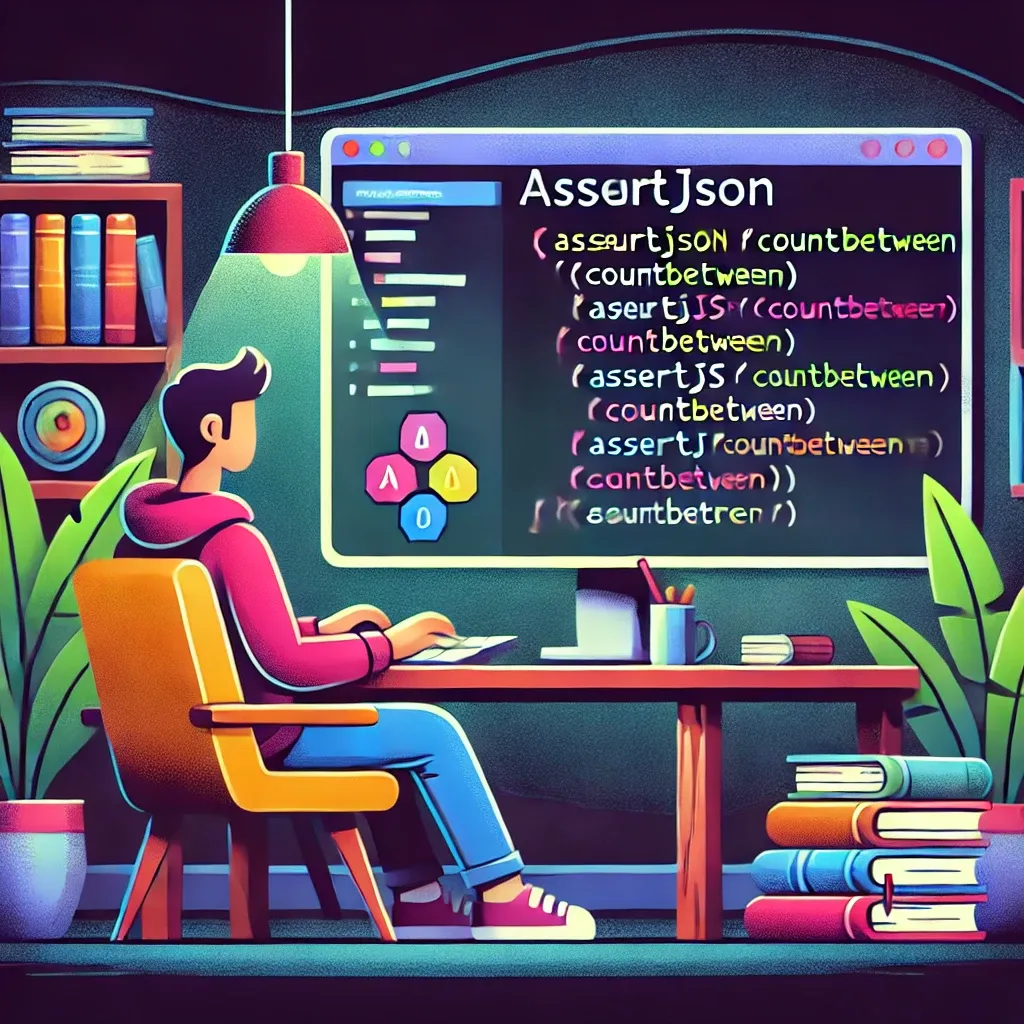
Need to test if a count falls within a specific range in your JSON responses? Laravel's new countBetween
method for AssertableJson
makes this validation a breeze! Let's see how this handy addition simplifies your tests.
Using countBetween
The new method lets you assert that a count falls within a specific range:
$response->assertJson(fn (AssertableJson $json) => $json
->countBetween(10, 30)
->etc()
);
Real-World Example
Here's how you might use it in a test for a paginated API:
class PostApiTest extends TestCase
{
public function test_search_returns_reasonable_results()
{
// Create some test posts
Post::factory()->count(20)->create([
'title' => 'Contains searchword'
]);
$response = $this->getJson('/api/posts?search=searchword');
$response->assertJson(fn (AssertableJson $json) => $json
->has('data')
->countBetween(5, 25)
->where('meta.search', 'searchword')
->etc()
);
}
public function test_related_posts_suggestions()
{
$post = Post::factory()->create();
$response = $this->getJson("/api/posts/{$post->id}/related");
$response->assertJson(fn (AssertableJson $json) => $json
->countBetween(3, 10) // Expect 3-10 related posts
->each(fn ($json) => $json
->has('id')
->has('title')
->etc()
)
);
}
}
This new assertion method makes it easy to test flexible count requirements in your JSON responses, perfect for scenarios where exact counts aren't necessary.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!