Testing Failed Jobs in Laravel with assertFailedWith
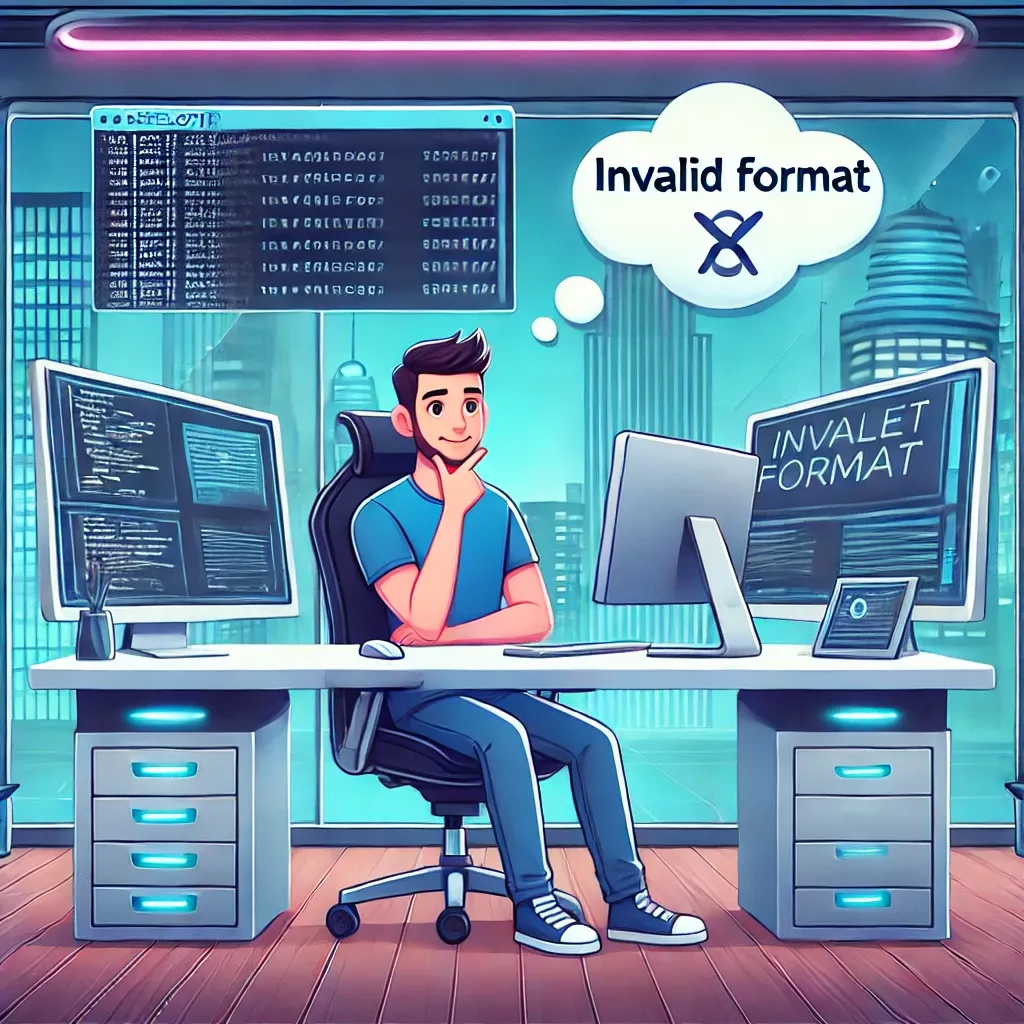
Need to test job failures more precisely? Laravel's new assertFailedWith method lets you verify exactly how your queued jobs fail, making your tests more specific and reliable.
Basic Usage
Test job failures with different assertions:
use App\Jobs\ProcessPodcast;
use App\Exceptions\MyException;
$job = (new ProcessPodcast)->withFakeQueueInteractions();
// Test with message
$job->assertFailedWith('whoops');
// Test with exception class
$job->assertFailedWith(MyException::class);
// Test with exception instance
$job->assertFailedWith(new MyException);
// Test with specific message
$job->assertFailedWith(new MyException(message: 'whoops'));
// Test with message and code
$job->assertFailedWith(new MyException(message: 'whoops', code: 123));
Real-World Example
Here's how you might use it in your test suite:
class JobFailureTest extends TestCase
{
public function test_podcast_processing_fails_with_invalid_format()
{
$job = new ProcessPodcastJob($invalidPodcast)
->withFakeQueueInteractions();
$job->handle();
$job->assertFailedWith(
new InvalidFormatException('Unsupported audio format')
);
}
public function test_email_job_handles_server_timeout()
{
$job = new SendBulkEmailJob($recipients)
->withFakeQueueInteractions();
$job->handle();
$job->assertFailedWith(
new ConnectionException('SMTP timeout', 504)
);
}
public function test_image_processing_handles_missing_file()
{
$job = (new ProcessImageJob('non-existent.jpg'))
->withFakeQueueInteractions();
$job->handle();
$job->assertFailedWith('File not found: non-existent.jpg');
}
}
The assertFailedWith method makes it easy to ensure your jobs fail appropriately under different conditions.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!