Testing Exception Reporting in Laravel with assertReported
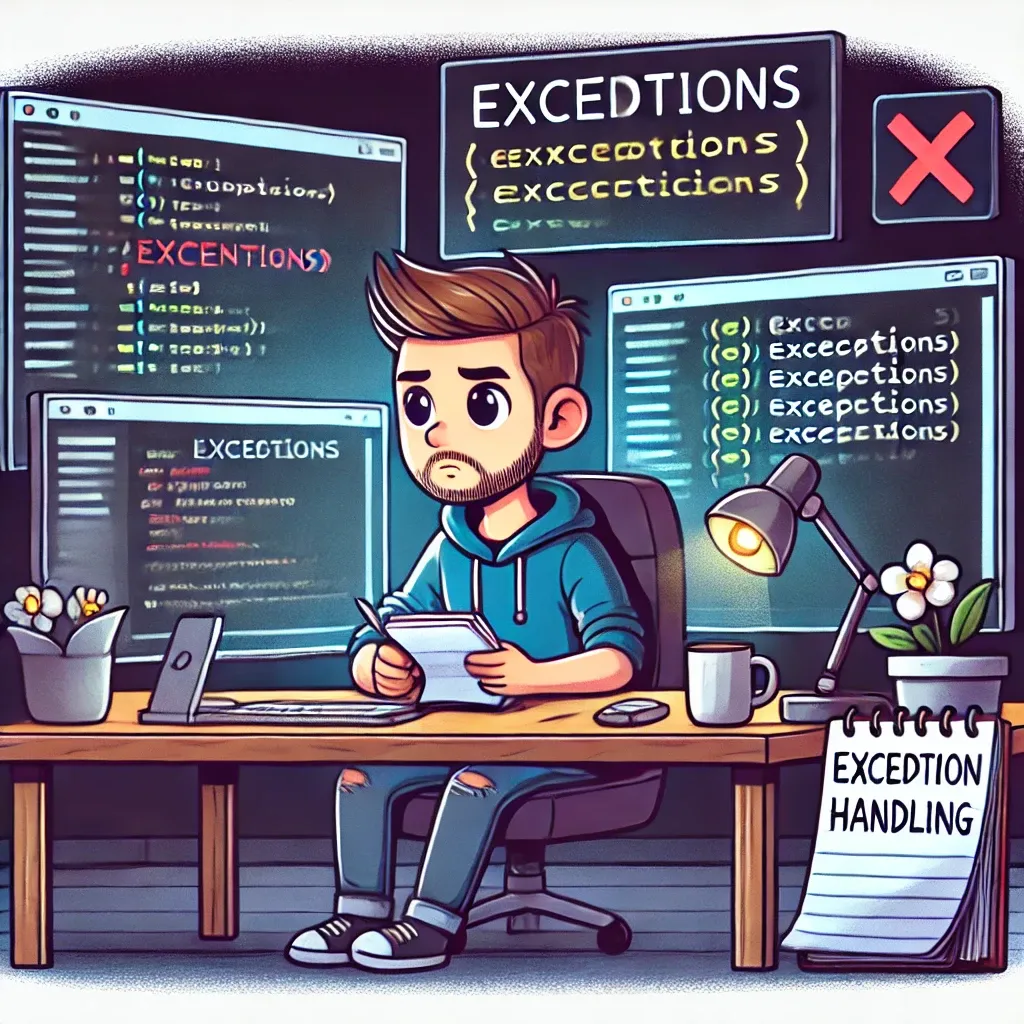
Testing exception flows in Laravel applications can be challenging. Discover how the Exceptions facade's assertReported method makes verifying exception reporting straightforward and reliable.
When developing robust applications, properly handling and reporting exceptions is crucial. However, testing that your exceptions are being correctly reported can be difficult without the right tools. Laravel's Exceptions facade offers an elegant solution with its assertReported
method, allowing you to verify that specific exceptions are properly captured during testing.
Let's see how it works:
use Illuminate\Support\Facades\Exceptions;
test('exception reporting works correctly', function () {
Exceptions::fake();
$this->post('/generate-report', [
'throw' => 'true',
])->assertStatus(503); // Service Unavailable
Exceptions::assertReported(ServiceUnavailableException::class);
});
Real-World Example
Here's how you might use the assertReported
method in a more detailed test case for an API endpoint:
use Illuminate\Support\Facades\Exceptions;
use App\Exceptions\InvalidDataException;
use App\Exceptions\DatabaseConnectionException;
test('order creation reports appropriate exceptions', function () {
Exceptions::fake();
// Test invalid data exception
$this->postJson('/api/orders', [
'items' => [],
])->assertStatus(422);
Exceptions::assertReported(InvalidDataException::class);
// Test database exception
$this->withDatabaseFailure()
->postJson('/api/orders', [
'items' => [['product_id' => 1, 'quantity' => 5]],
])->assertStatus(500);
Exceptions::assertReported(DatabaseConnectionException::class);
// You can also make more specific assertions about the exception
Exceptions::assertReported(function (DatabaseConnectionException $exception) {
return $exception->getMessage() === 'Failed to connect to database' &&
$exception->getCode() === 1045;
});
});
The assertReported
method offers two powerful approaches for verification. First, you can use class-based assertion to verify that an exception of a specific class was reported:
Exceptions::assertReported(ServiceUnavailableException::class);
Alternatively, you can use callback-based assertion to verify details about the reported exception:
Exceptions::assertReported(function (ServiceUnavailableException $exception) {
return $exception->getMessage() === 'Service is currently unavailable';
});
This flexibility allows you to not only test that the correct type of exception was reported but also verify specific properties of the exception such as its message, code, or custom attributes.
The assertReported
method is particularly valuable when testing complex workflows where exception handling is critical, such as payment processing, API integrations, or scheduled tasks. It ensures that your application is correctly identifying and reporting errors, which is essential for proper monitoring and debugging in production environments.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter, Bluesky, and YouTube. It helps a lot!