Taming Your Routes: Mastering Parameter Constraints in Laravel
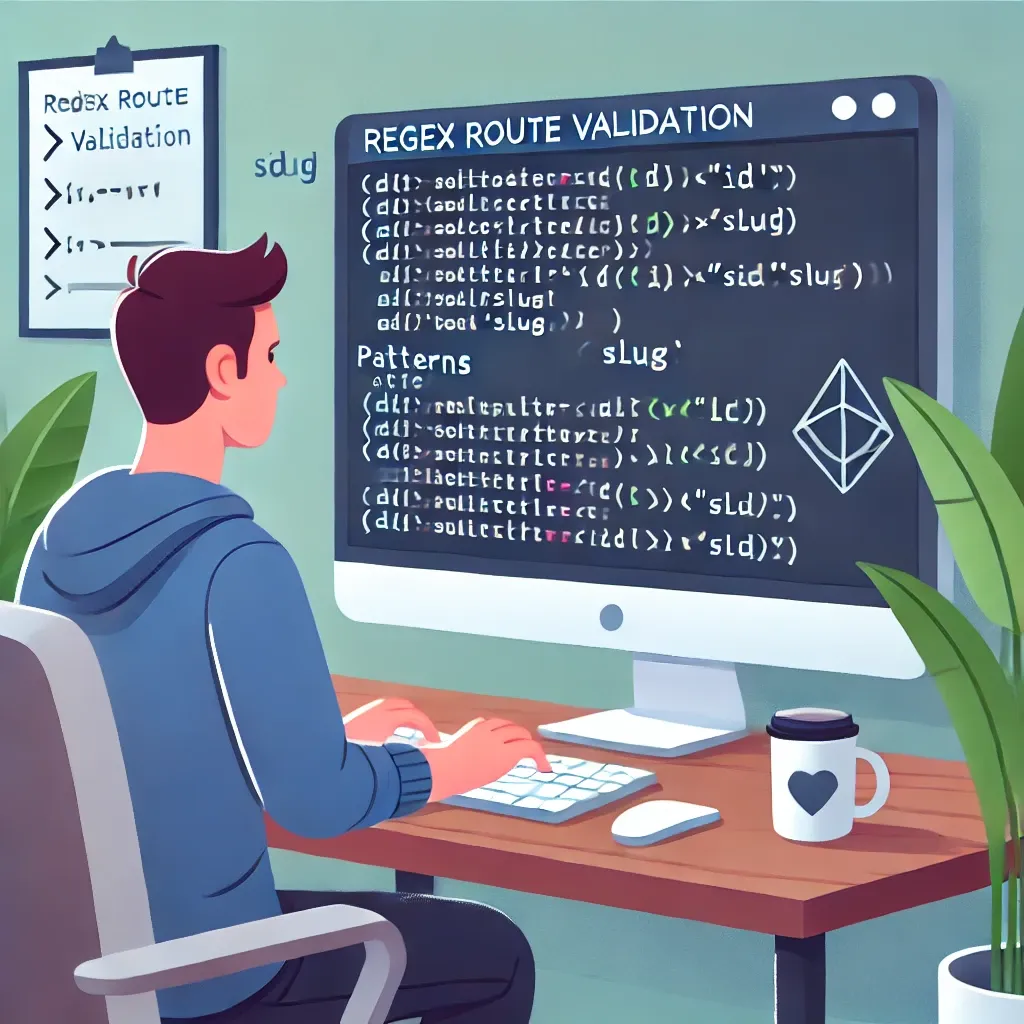
Ever worried about users entering weird stuff in your URL parameters? Laravel's where
method is here to save the day! Let's dive into how you can keep your routes in check with some simple constraints.
The Power of where
The where
method lets you set rules for your route parameters using regular expressions. It's like having a bouncer for your URLs!
Basic Usage
Here's how you can use where
:
Route::get('/user/{name}', function (string $name) {
// ...
})->where('name', '[A-Za-z]+');
Getting Specific with Numbers
Need to make sure an ID is actually a number? No problem:
Route::get('/user/{id}', function (string $id) {
// ...
})->where('id', '[0-9]+');
This route will only match if {id}
is made up of digits.
Handling Multiple Parameters
Got more than one parameter to wrangle? You can chain them together:
Route::get('/user/{id}/{name}', function (string $id, string $name) {
// ...
})->where(['id' => '[0-9]+', 'name' => '[a-z]+']);
Here, we're saying:
id
must be numbersname
must be lowercase letters
Real-World Example: Blog Post URLs
Let's say you're building a blog with pretty URLs. Here's how you might set it up:
use App\Http\Controllers\BlogController;
Route::get('/blog/{year}/{month}/{slug}', [BlogController::class, 'show'])
->where([
'year' => '\d{4}',
'month' => '0[1-9]|1[0-2]',
'slug' => '[a-z0-9\-]+'
]);
In this example:
year
must be exactly 4 digitsmonth
must be 01-12slug
can contain lowercase letters, numbers, and hyphens
This ensures URLs like /blog/2023/05/my-awesome-post
work, but /blog/not-a-year/13/oops!
won't match.
By using the where
method, you're not just writing routes – you're crafting a robust, self-validating URL structure for your app. It's a simple technique that can save you headaches down the road!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!