Swift Soft Delete Management with Laravel's forceDestroy
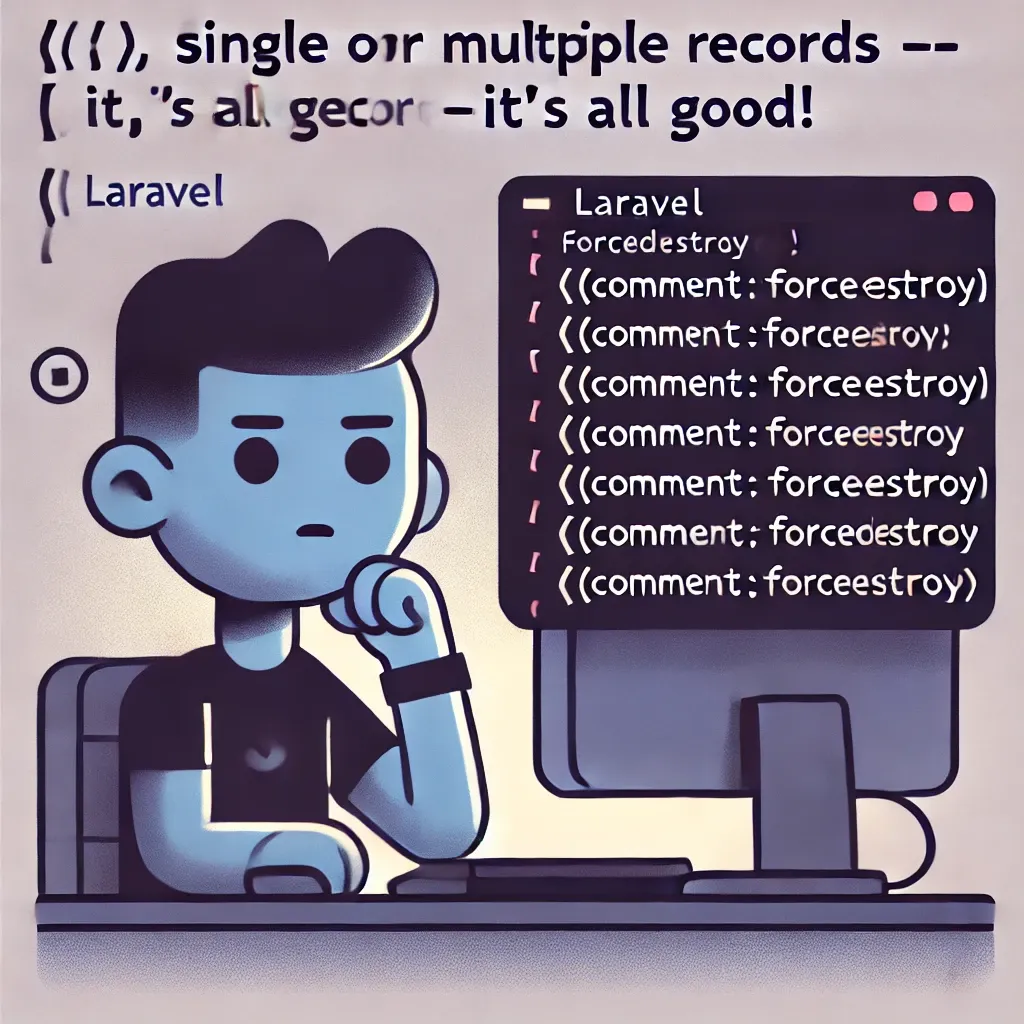
Need to permanently delete soft-deleted records? Laravel's new forceDestroy
method streamlines the process! Let's explore this convenient addition to the SoftDeletes
toolkit.
The Old vs New Way
The traditional approach required finding the model first:
// Old way
$comment = Comment::find(1);
$comment->forceDelete();
// New way
Comment::forceDestroy(1);
Working with Multiple Records
You can also destroy multiple records at once and get the count:
// Delete multiple records and get count
$count = Comment::forceDestroy([1, 2, 3]);
Real-World Example
Here's how you might use it in a comment moderation system:
class CommentModerator
{
public function purgeSpam(array $spamCommentIds)
{
$purgedCount = Comment::forceDestroy($spamCommentIds);
Log::info("Permanently removed {$purgedCount} spam comments");
event(new SpamCommentsPurged($spamCommentIds));
return [
'status' => 'success',
'purged_count' => $purgedCount,
'message' => "{$purgedCount} spam comments were permanently removed"
];
}
}
The new forceDestroy
method makes permanent deletion simpler and more efficient, especially when dealing with multiple records.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!