Supercharging String Input Handling in Laravel with Stringable
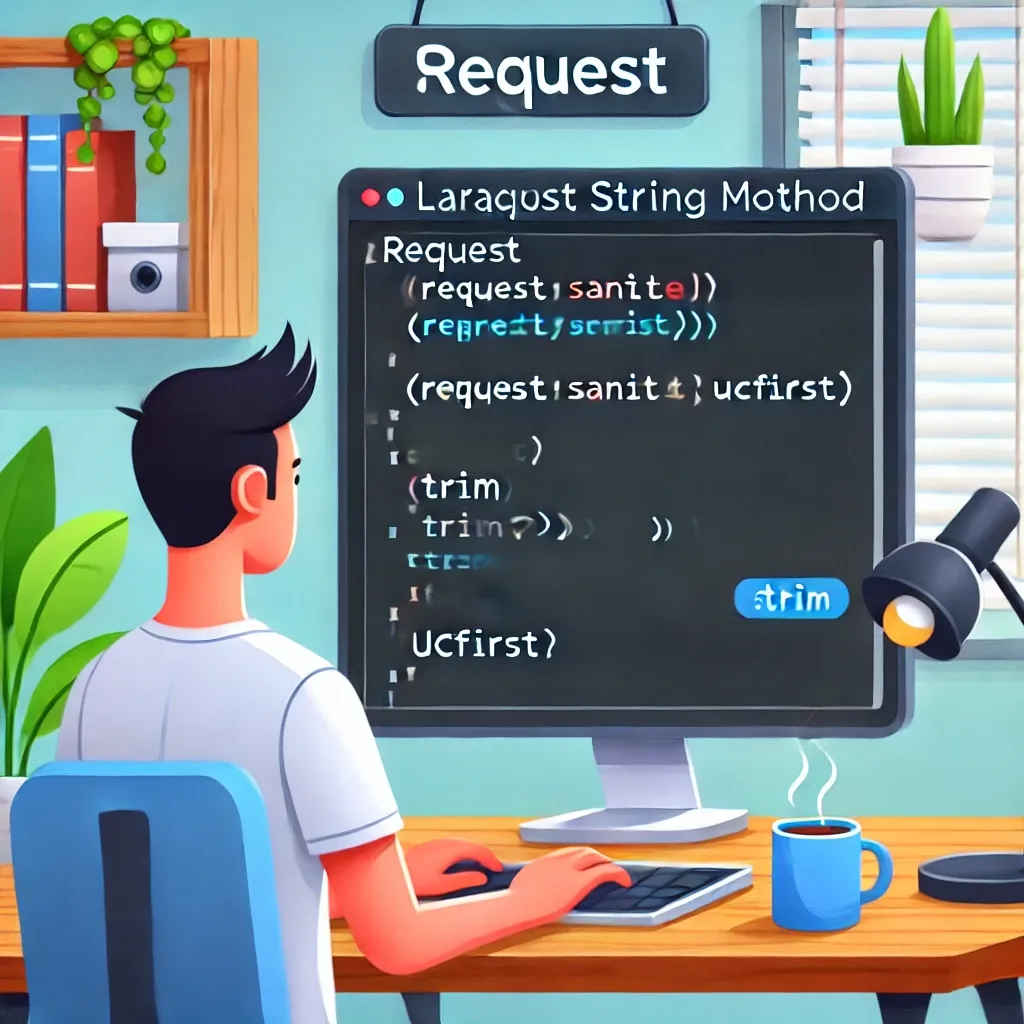
When building web applications, handling user input efficiently is crucial. Laravel offers a powerful yet often overlooked feature: the ability to retrieve request input as Stringable
instances using the string
method. This simple trick can significantly enhance your string manipulation capabilities. Let's dive in and see how you can leverage this feature in your Laravel applications.
Understanding $request->string()
The string
method on the request object allows you to retrieve input data as an instance of Illuminate\Support\Stringable
. Here's how you use it:
$name = $request->string('name');
This single line transforms your input into a Stringable
instance, giving you access to a wide array of string manipulation methods.
Real-Life Example
Let's consider a scenario where we're building a user registration form. We want to ensure that the user's name is properly formatted before saving it to the database. Here's how we might handle this using $request->string()
:
public function register(Request $request)
{
$name = $request->string('name')
->trim()
->ucwords()
->limit(50);
$email = $request->string('email')
->trim()
->lower();
$user = User::create([
'name' => $name->toString(),
'email' => $email->toString(),
'password' => Hash::make($request->input('password')),
]);
return response()->json($user, 201);
}
In this example, we're using Stringable
methods to:
- Trim whitespace from the name and email
- Convert the name to title case with
ucwords()
- Limit the name to 50 characters
- Convert the email to lowercase
Here's what the input and output might look like:
// Input
{
"name": " jOHN doe ",
"email": " JOHN@EXAMPLE.COM ",
"password": "secret123"
}
// Output
{
"id": 1,
"name": "John Doe",
"email": "john@example.com",
"created_at": "2023-06-15T14:30:00.000000Z",
"updated_at": "2023-06-15T14:30:00.000000Z"
}
By leveraging $request->string()
, you can significantly simplify your input handling logic, making your code more readable and maintainable. Whether you're trimming, transforming, or validating string input, Stringable
instances provide a powerful toolset to handle these operations efficiently.
Here's a quick reference of some useful Stringable
methods you can chain:
trim()
: Remove whitespace from both endsltrim()
/rtrim()
: Remove whitespace from left or right sideupper()
/lower()
: Convert to uppercase or lowercasetitle()
: Convert to title caseslug()
: Generate a URL-friendly "slug"limit()
: Limit the number of charactersreplaceFirst()
/replaceLast()
: Replace occurrences of a substringbefore()
/after()
: Get part of the string before or after a given substring
Remember to call toString()
when you need to convert the Stringable
instance back to a primitive string, such as when saving to the database or returning in a response.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!