Supercharging Request Input Handling in Laravel with Collections
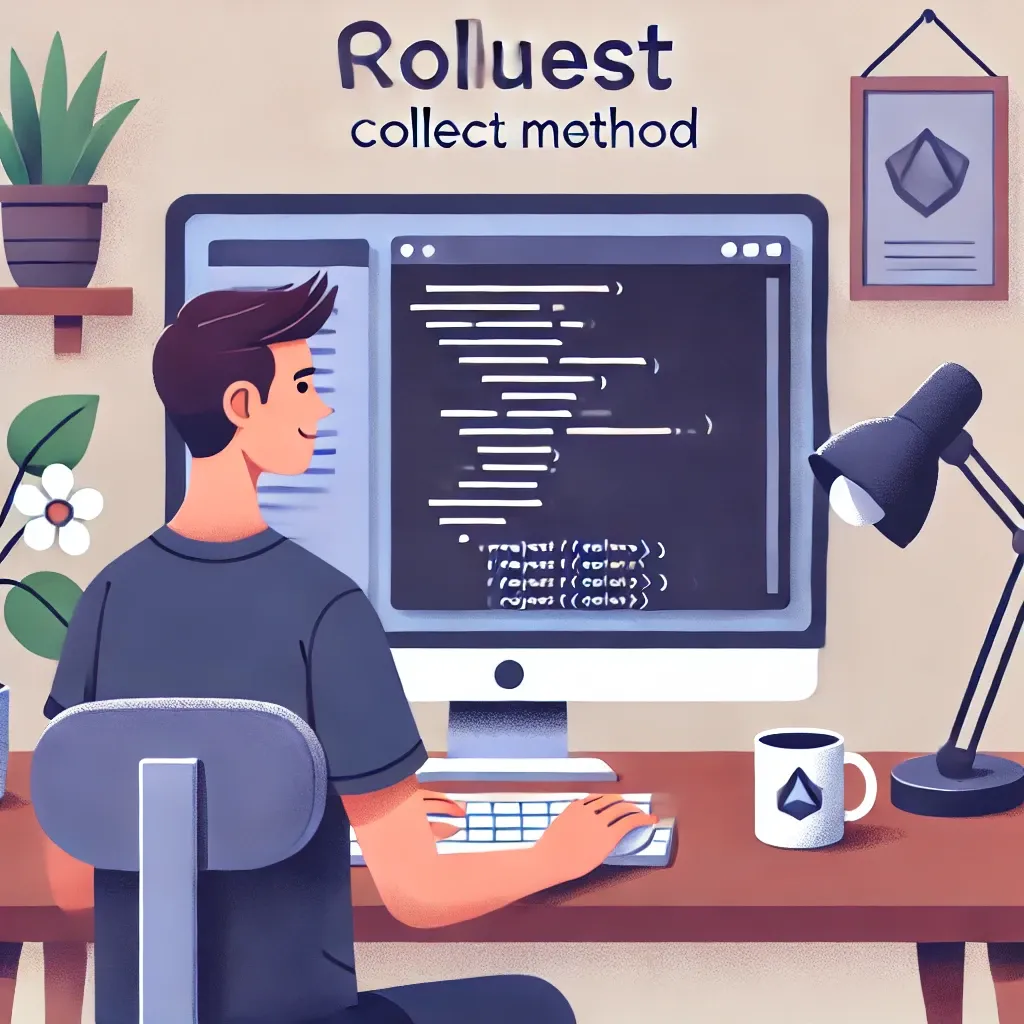
When building web applications, handling request input efficiently is crucial. Laravel offers a powerful yet often overlooked feature: the ability to transform all incoming request data into a collection using the collect
method. This simple trick can significantly enhance your input processing capabilities. Let's dive in and see how you can leverage this feature in your Laravel applications.
Understanding $request->collect()
The collect
method on the request object allows you to retrieve all incoming request input data as a Laravel Collection. Here's how you use it:
$input = $request->collect();
This single line transforms your request input into a collection, giving you access to all of Laravel's powerful collection methods.
Real-Life Example
Let's consider a scenario where we're building a user registration form with multiple fields, some of which are optional. We want to filter out any empty fields and transform the data before saving it to the database.
Here's how we might handle this using $request->collect()
:
public function register(Request $request)
{
$input = $request->collect();
$userData = $input
->filter() // Remove any empty fields
->map(function ($value, $key) {
// Transform specific fields
if ($key === 'name') {
return ucwords($value);
}
if ($key === 'email') {
return strtolower($value);
}
return $value;
})
->toArray();
$user = User::create($userData);
return response()->json($user, 201);
}
In this example, we're using collection methods to:
- Filter out any empty fields with
filter()
- Transform the name to title case and email to lowercase with
map()
- Convert the collection back to an array for creating the user
Here's what the input and output might look like:
// Input
{
"name": "john doe",
"email": "JOHN@EXAMPLE.COM",
"age": "30",
"bio": ""
}
// Output
{
"id": 1,
"name": "John Doe",
"email": "john@example.com",
"age": "30",
"created_at": "2023-06-15T14:30:00.000000Z",
"updated_at": "2023-06-15T14:30:00.000000Z"
}
Note how the empty 'bio' field was removed, the name was capitalized, and the email was converted to lowercase.
By leveraging $request->collect()
, you can significantly simplify your input handling logic, making your code more readable and maintainable. Whether you're filtering, transforming, or validating input data, collections provide a powerful toolset to handle these operations efficiently.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!