Supercharge Your Laravel Queries with Relation Aggregates
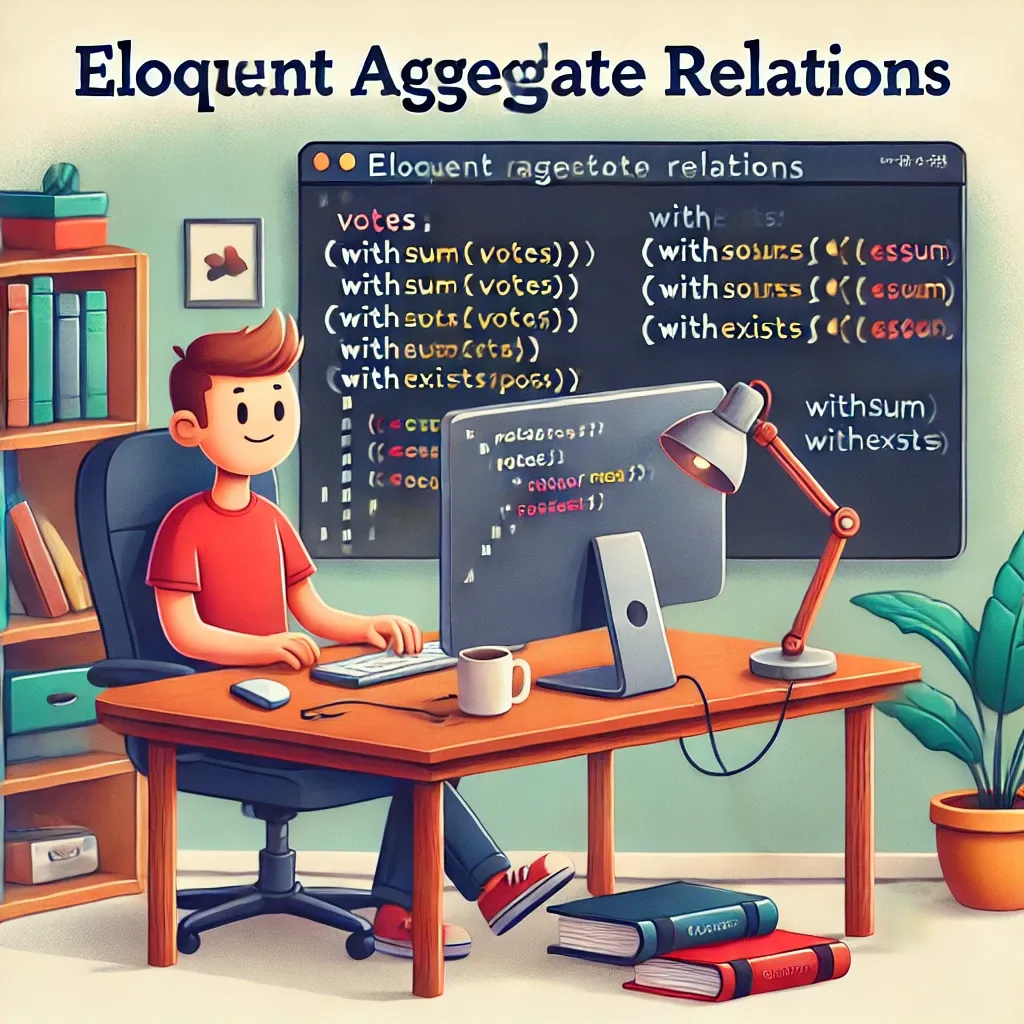
When building complex applications with Laravel, you often need to retrieve aggregated data from related models. Eloquent provides a set of powerful methods to accomplish this efficiently: withSum
, withAvg
, withMax
, withMin
, and withExists
. These methods allow you to include aggregated data from relationships without writing complex, nested queries.
Understanding Relation Aggregates
Relation aggregates in Laravel allow you to add computed values to your Eloquent models based on related data. These methods add a {relation}_{function}_{column}
attribute to your resulting models, making it easy to access the aggregated data.
Basic Usage
Let's look at a basic example using the withSum
method:
$posts = Post::withSum('comments', 'votes')->get();
foreach ($posts as $post) {
echo $post->comments_sum_votes;
}
In this example, each Post
model will have a comments_sum_votes
attribute containing the sum of all votes from related comments.
Available Aggregate Methods
Laravel provides several aggregate methods:
withSum
: Sums a column from the related modelswithAvg
: Calculates the average of a columnwithMax
: Finds the maximum value of a columnwithMin
: Finds the minimum value of a columnwithExists
: Checks if related models exist
Custom Aliases
If you prefer a different name for the aggregated attribute, you can specify a custom alias:
$posts = Post::withSum('comments as total_votes', 'votes')->get();
foreach ($posts as $post) {
echo $post->total_votes;
}
Combining with Eager Loading
These methods work seamlessly with Laravel's eager loading:
$posts = Post::with('user')
->withSum('comments', 'votes')
->withExists('comments')
->get();
Deferred Execution
Laravel also provides deferred versions of these methods, allowing you to perform aggregations on already retrieved models:
$post = Post::first();
$post->loadSum('comments', 'votes');
Use with Select: When combining these methods with select
, ensure you call the aggregate methods after the select
method:
$posts = Post::select(['title', 'body'])
->withExists('comments')
->get();
By leveraging these powerful aggregate methods, you can significantly simplify your Laravel code and improve its efficiency. They allow you to retrieve complex, aggregated data with clean, expressive syntax, keeping your application's logic tidy and performant.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!