Supercharge your Laravel API with HTTP Response Caching
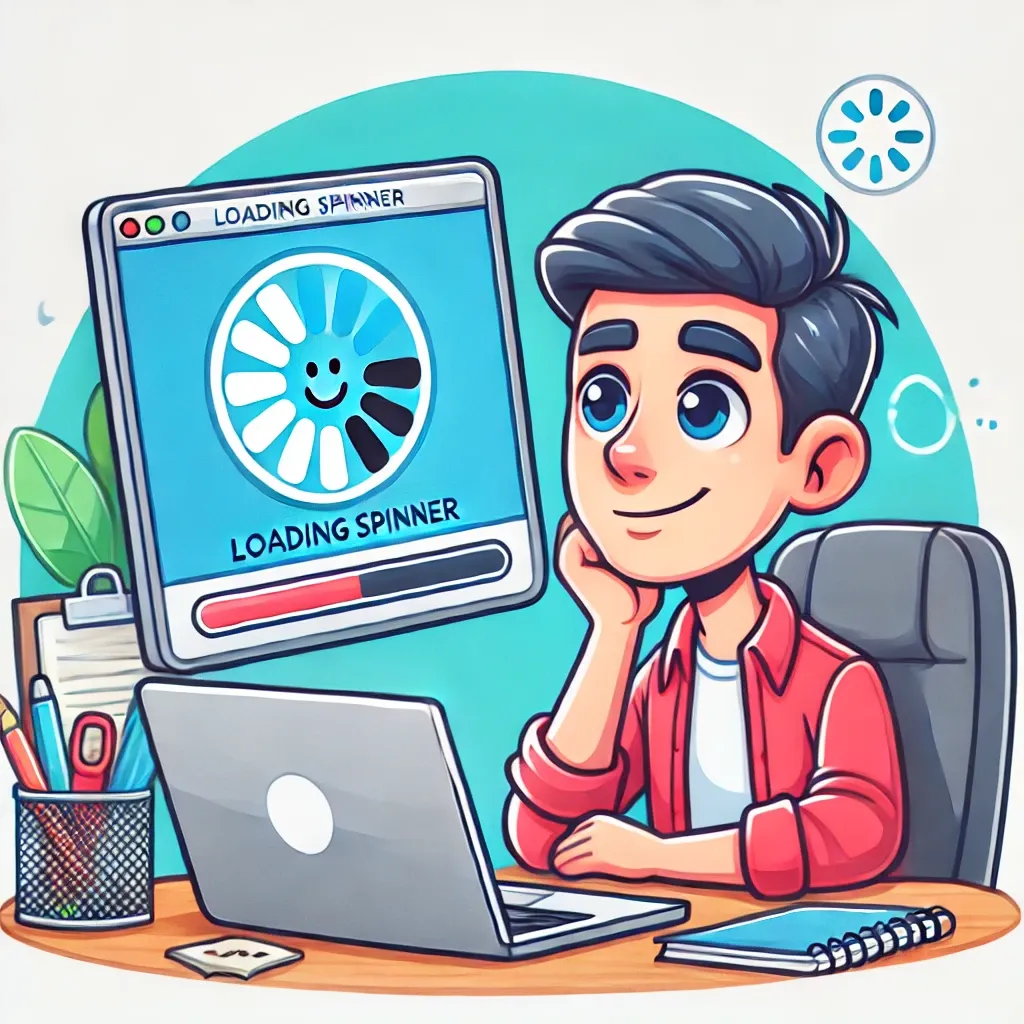
As developers, we're always looking for ways to optimize our applications and improve performance. One powerful technique that often goes underutilized is HTTP response caching. In this post, we'll explore how to implement HTTP response caching in Laravel to boost your API performance significantly.
What is HTTP Response Caching?
HTTP response caching is a technique where we instruct clients (browsers or API consumers) to store a copy of the response locally for a specified period. This reduces the number of requests to the server, lowering server load and improving response times for subsequent requests.
Implementing HTTP Response Caching in Laravel
Laravel makes it easy to add caching headers to your API responses. Here's how you can do it:
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Cache;
class ProductController extends Controller
{
public function show($id, Request $request)
{
$product = Cache::remember("product:{$id}", 3600, function () use ($id) {
return Product::findOrFail($id);
});
return response()->json($product)
->header('Cache-Control', 'public, max-age=3600')
->setEtag(md5($product->updated_at));
}
}
Let's break down what's happening here:
- We use Laravel's
Cache::remember()
method to cache the product data for an hour (3600 seconds). - We set the
Cache-Control
header to instruct clients to cache the response for an hour. - We add an ETag header, which allows clients to check if their cached version is still valid without downloading the entire response again.
Benefits of HTTP Response Caching
- Reduced Server Load: Clients can serve cached responses without hitting your server.
- Improved Response Times: Cached responses are served instantly from the client's local storage.
- Bandwidth Savings: Less data transferred means lower bandwidth costs.
- Better User Experience: Faster responses lead to a smoother user experience.
Real-Life Example: E-commerce Product Catalog
Let's consider a real-life scenario where HTTP response caching can make a significant impact: an e-commerce product catalog.
Imagine you're building an API for a large e-commerce platform with millions of products. The product details don't change frequently, but the API receives a high volume of requests. Here's how you might implement caching:
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Cache;
class ProductCatalogController extends Controller
{
public function index(Request $request)
{
$page = $request->get('page', 1);
$perPage = 20;
$cacheKey = "product_catalog:page:{$page}";
$products = Cache::remember($cacheKey, 3600, function () use ($page, $perPage) {
return Product::with('category')
->where('status', 'active')
->orderBy('name')
->paginate($perPage);
});
return response()->json($products)
->header('Cache-Control', 'public, max-age=3600')
->setEtag(md5($products->lastPage() . $products->total() . $products->currentPage()));
}
public function updateProduct($id, Request $request)
{
$product = Product::findOrFail($id);
$product->update($request->all());
// Invalidate cache for all pages
Cache::flush();
return response()->json(['message' => 'Product updated successfully']);
}
}
In this example:
- We cache each page of the product catalog for an hour.
- We use a combination of the last page, total products, and current page as the ETag to ensure cache invalidation when the product list changes.
- When a product is updated, we flush the entire cache to ensure all pages reflect the latest data.
This approach can dramatically reduce the load on your database and API servers. For instance, if you have 1,000 requests per second to your product catalog, with caching in place, you might only need to generate the response from the database once per hour per page, instead of 3,600,000 times!
Conclusion
HTTP response caching is a powerful tool in your Laravel performance optimization toolkit. By implementing it in your APIs, you can significantly reduce server load and improve response times, leading to a better experience for your users and lower costs for your infrastructure.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!