Supercharge your app with session caching using Cache::put
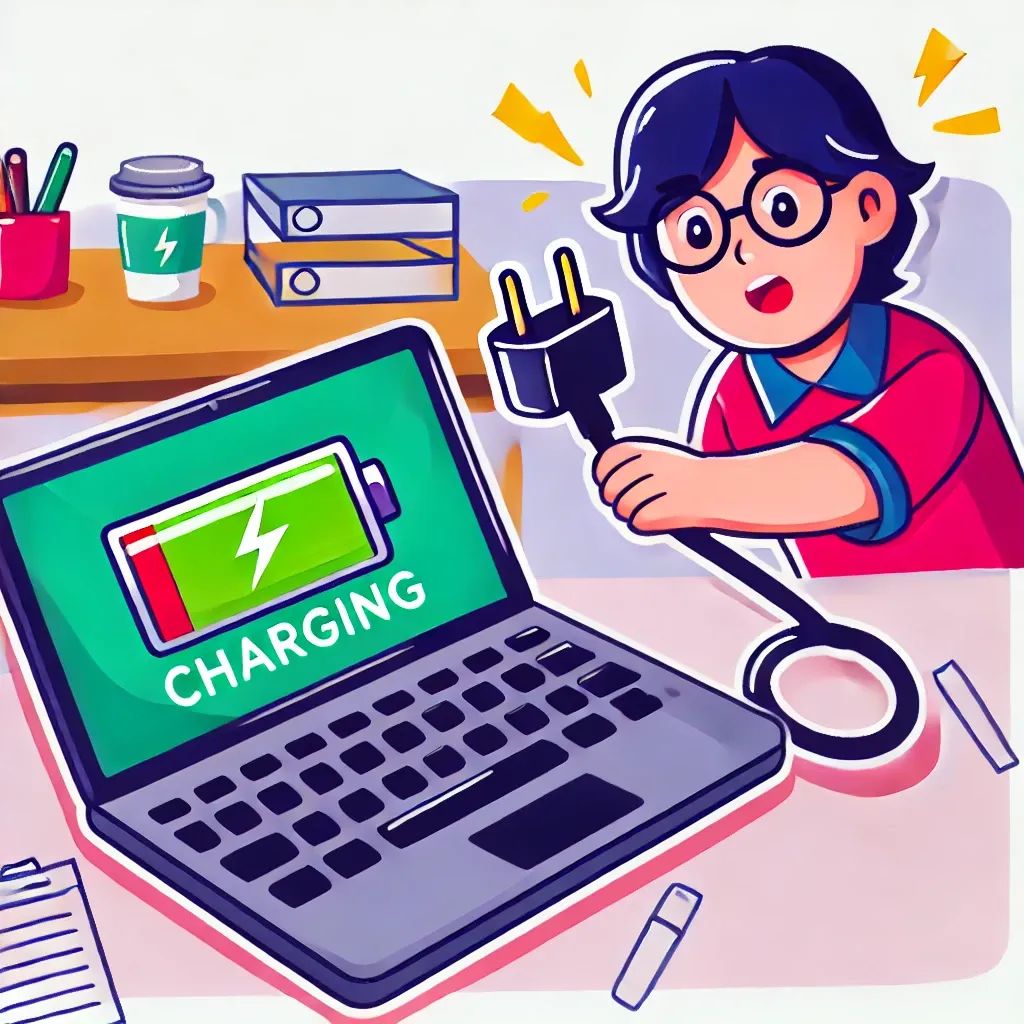
Hey Laravel devs, here is a gem for you! 💎
Efficiently managing session data is crucial for maintaining a fast and responsive web application. Laravel provides a simple yet powerful method, Cache::put()
, to handle session caching. In this blog post, we’ll explore how to use Cache::put()
to store session data and enhance your application's performance.
Why Use Session Caching?
Session caching allows you to store user session data temporarily, reducing the need to repeatedly fetch this data from the database. This can significantly improve your application's response time and reduce database load.
Benefits of Session Caching
Using session caching in your Laravel application offers several advantages:
- Improved Performance: By caching session data, you reduce the time it takes to retrieve user-specific information.
- Reduced Database Load: Decrease the number of queries hitting your database, which can improve its overall performance and stability.
- Persistent Sessions: Ensure that session data is available quickly and reliably.
How to Use Cache::put() in Laravel
Laravel makes it easy to implement session caching using the Cache::put()
method. Here’s how you can store and retrieve session data efficiently:
Storing Session Data
The Cache::put()
method allows you to store data in the cache for a specified period. Here’s an example of how to store user session data:
Cache::put('user_session', $sessionData, 60);
In this example, the user_session
key is used to store the session data in the cache for 60 minutes.
Retrieving Session Data
To retrieve the cached session data, use the Cache::get()
method:
$sessionData = Cache::get('user_session');
If the user_session
key exists in the cache, the data is returned. Otherwise, null
is returned.
Real-Life Example
Imagine you have an application where users can customize their dashboard settings. Each user's settings are stored in the database and need to be fetched every time they log in. By caching these settings, you can reduce the number of database queries and speed up the login process.
Here’s how you might implement session caching for user settings:
Example:
// Storing user settings in the cache
$userSettings = [
'theme' => 'dark',
'notifications' => true,
'language' => 'en'
];
Cache::put('user_settings_' . auth()->id(), $userSettings, 60);
// Retrieving user settings from the cache
$userSettings = Cache::get('user_settings_' . auth()->id(), function () {
return DB::table('settings')->where('user_id', auth()->id())->first();
});
In this example, user settings are cached for 60 minutes. If the settings are not found in the cache, they are fetched from the database and stored in the cache for future requests.
Pro Tip
When using session caching, choose an appropriate expiration time for your cached data. This ensures that the data remains fresh and relevant. Also, consider clearing the cache when the user updates their settings to ensure that the cached data is always up-to-date.
Using Laravel's Cache::put()
method for session caching is a powerful way to enhance the performance of your application. By reducing the need to repeatedly fetch session data from the database, you can improve response times and provide a better user experience. Start leveraging session caching in your Laravel projects today!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!