String Repetition in Laravel with repeat Method
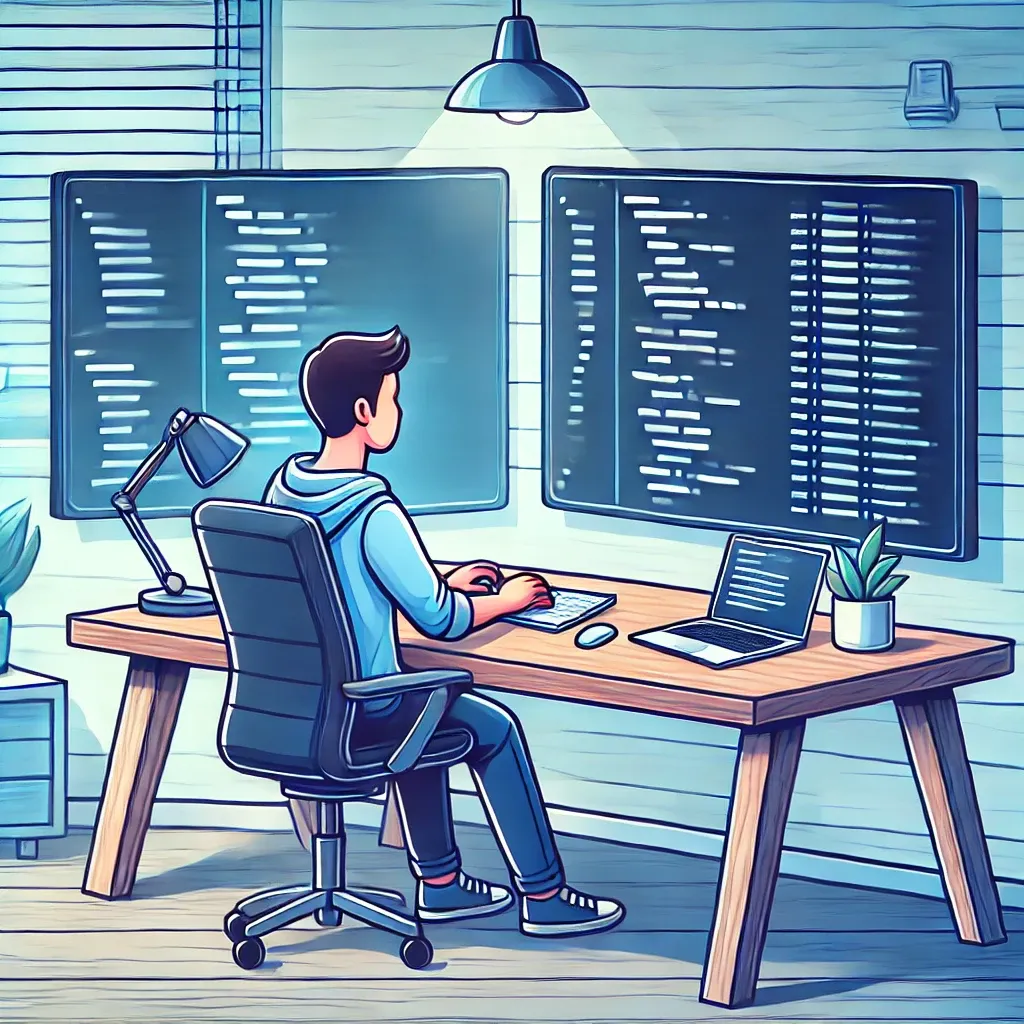
Need to repeat a string multiple times? Laravel's Str::repeat method offers a simple solution for creating repeated string patterns.
Basic Usage
Repeat a string a specific number of times:
use Illuminate\Support\Str;
$string = 'a';
$repeat = Str::repeat($string, 5);
// Result: 'aaaaa'
Real-World Example
Here's how you might use it in an HTML generator:
class HtmlGenerator
{
public function createSpacerElement(int $size = 1)
{
// Creates margin using em units
return sprintf(
'<div style="margin-bottom: %s"></div>',
Str::repeat('1em ', $size)
);
}
public function generateStarRating(int $rating, int $maxStars = 5)
{
$fullStars = Str::repeat('★', $rating);
$emptyStars = Str::repeat('☆', $maxStars - $rating);
return sprintf(
'<div class="rating">%s%s</div>',
$fullStars,
$emptyStars
);
}
public function createLoadingIndicator(int $dots = 3)
{
return sprintf(
'Loading%s',
Str::repeat('.', $dots)
);
}
}
// Usage
$html = new HtmlGenerator();
echo $html->createSpacerElement(2);
// Output: <div style="margin-bottom: 1em 1em "></div>
echo $html->generateStarRating(3);
// Output: <div class="rating">★★★☆☆</div>
echo $html->createLoadingIndicator();
// Output: Loading...
The repeat method provides a clean way to handle string repetition tasks in your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!