String Pattern Replacement in Laravel: Using replaceMatches
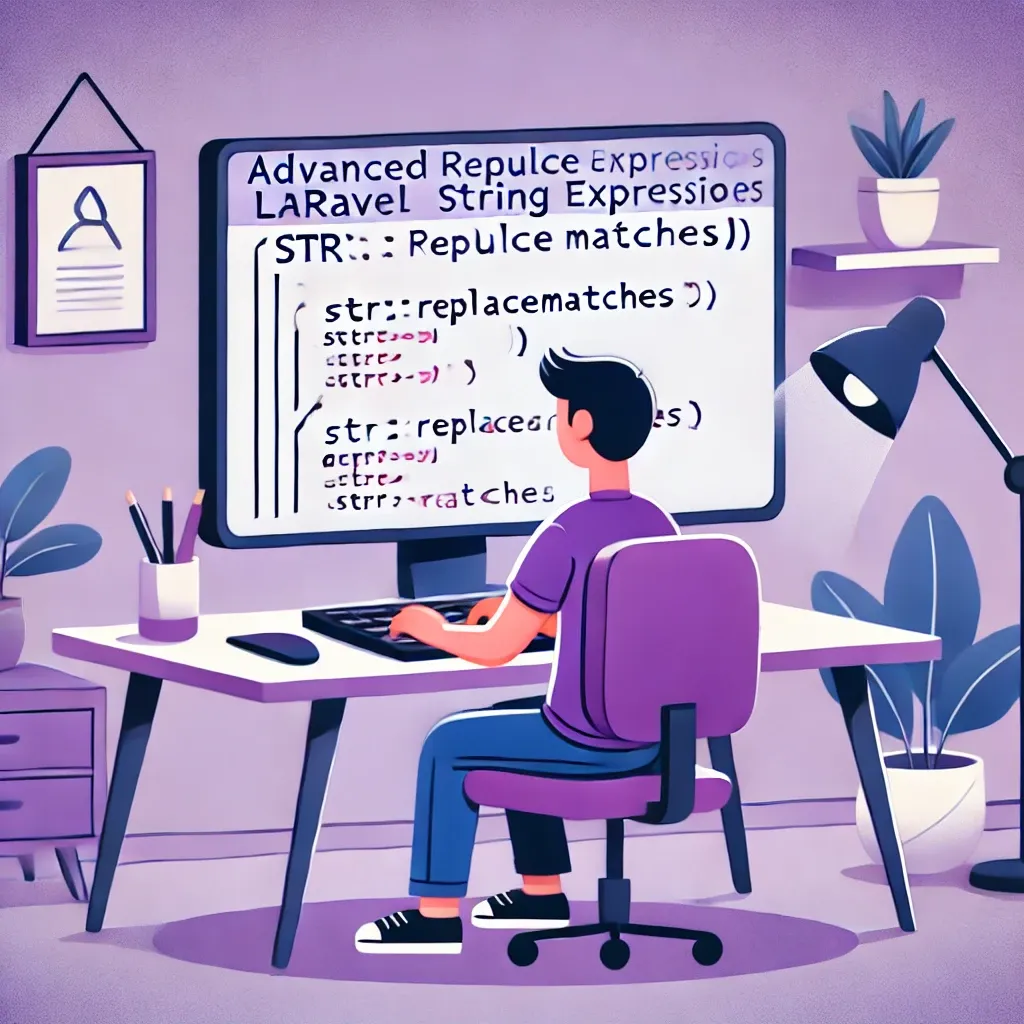
Learn how to perform advanced string pattern replacement in Laravel using the Str::replaceMatches method. Let's explore both simple replacements and complex patterns with closure-based replacements.
Basic Usage
Here's a simple pattern replacement:
use Illuminate\Support\Str;
$replaced = Str::replaceMatches(
pattern: '/[^A-Za-z0-9]++/',
replace: '',
subject: '(+1) 501-555-1000'
);
// Result: '15015551000'
Using Closures for Complex Replacements
$replaced = Str::replaceMatches('/\d/', function (array $matches) {
return '[' . $matches[0] . ']';
}, '123');
// Result: '[1][2][3]'
Real-World Example
Here's how you might use it in a text formatting service:
class TextFormatter
{
public function formatPhoneNumbers(string $text): string
{
return Str::replaceMatches(
'/(\d{3})[\s-]?(\d{3})[\s-]?(\d{4})/',
fn($matches) => "({$matches[1]}) {$matches[2]}-{$matches[3]}",
$text
);
}
public function highlightKeywords(string $text, array $keywords): string
{
$pattern = '/(' . implode('|', array_map('preg_quote', $keywords)) . ')/i';
return Str::replaceMatches(
$pattern,
fn($matches) => '<mark>' . $matches[0] . '</mark>',
$text
);
}
public function cleanupMarkdown(string $markdown): string
{
// Remove extra spaces around bold/italic markers
return Str::replaceMatches(
'/\s*([*_]{1,2})\s*([^*_]+)\s*([*_]{1,2})\s*/',
fn($matches) => $matches[1] . $matches[2] . $matches[3],
$markdown
);
}
}
// Usage
$formatter = new TextFormatter();
$text = "Call me at 1234567890 or email at john@example.com";
echo $formatter->formatPhoneNumbers($text);
// "Call me at (123) 456-7890 or email at john@example.com"
$content = "Laravel is a web application framework.";
echo $formatter->highlightKeywords($content, ['Laravel', 'framework']);
// "<mark>Laravel</mark> is a web application <mark>framework</mark>."
$markdown = "This is **bold** and *italic* text";
echo $formatter->cleanupMarkdown($markdown);
// "This is **bold** and *italic* text"
The replaceMatches
method provides a powerful way to perform pattern-based string replacements, whether you need simple substitutions or complex transformations using closure callbacks.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!