Streamlining View Logic with Laravel View Composers
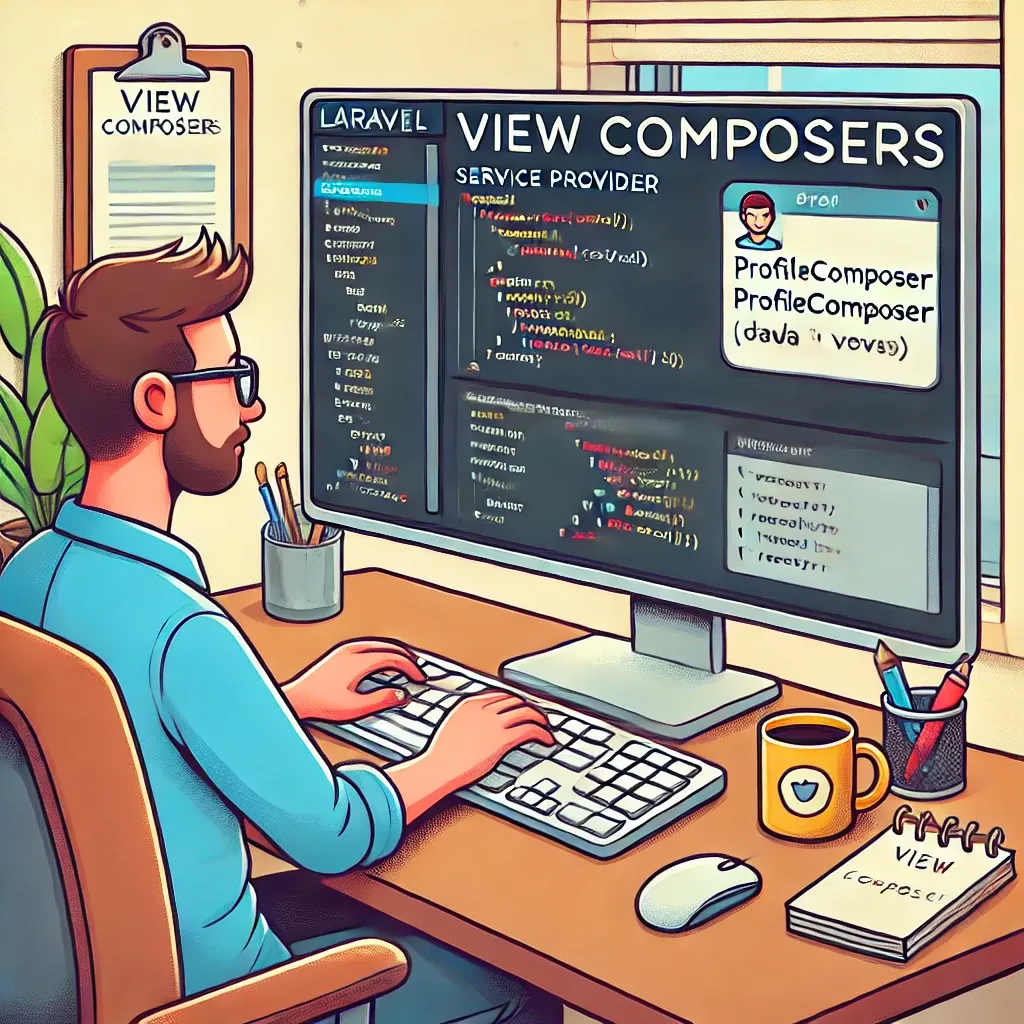
In Laravel applications, you often find yourself passing the same data to multiple views. This can lead to repetitive controller code and potential inconsistencies. Laravel's View Composers offer an elegant solution to this problem, allowing you to organize and manage view-specific logic more efficiently.
Understanding View Composers
View composers are callbacks or class methods that are called when a specific view is rendered. They allow you to bind data to a view every time it's rendered, reducing repetition and centralizing view-specific logic.
Basic Usage of View Composers
To create a view composer, you typically register it in one of your service providers. Let's look at an example in the AppServiceProvider
:
use Illuminate\Support\Facades\View;
use App\View\Composers\ProfileComposer;
public function boot()
{
View::composer('profile', ProfileComposer::class);
}
Now, let's create the ProfileComposer
class:
namespace App\View\Composers;
use App\Repositories\UserRepository;
use Illuminate\View\View;
class ProfileComposer
{
protected $users;
public function __construct(UserRepository $users)
{
$this->users = $users;
}
public function compose(View $view)
{
$view->with('count', $this->users->count());
}
}
Every time the 'profile' view is rendered, the compose
method will be called, adding the user count to the view data.
Using Closures for Simple Composers
For simpler cases, you can use closures directly:
View::composer('dashboard', function ($view) {
$view->with('stats', $this->getDashboardStats());
});
Wildcard Composers
You can use wildcards to attach a composer to multiple views:
View::composer('admin.*', AdminComposer::class);
This will attach the AdminComposer
to all views within the 'admin' directory.
Real-World Example: Sidebar with Dynamic Content
Let's consider a more complex example where we want to populate a sidebar with dynamic content across multiple pages:
// app/View/Composers/SidebarComposer.php
namespace App\View\Composers;
use Illuminate\View\View;
use App\Repositories\CategoryRepository;
use App\Repositories\TagRepository;
class SidebarComposer
{
protected $categories;
protected $tags;
public function __construct(CategoryRepository $categories, TagRepository $tags)
{
$this->categories = $categories;
$this->tags = $tags;
}
public function compose(View $view)
{
$view->with([
'popularCategories' => $this->categories->popular(5),
'recentTags' => $this->tags->recent(10),
'archivedMonths' => $this->categories->archivedMonths(),
]);
}
}
// app/Providers/AppServiceProvider.php
public function boot()
{
View::composer('layouts.sidebar', SidebarComposer::class);
}
// resources/views/layouts/sidebar.blade.php
<div class="sidebar">
<h3>Popular Categories</h3>
<ul>
@foreach($popularCategories as $category)
<li><a href="{{ route('category.show', $category) }}">{{ $category->name }}</a></li>
@endforeach
</ul>
<h3>Recent Tags</h3>
<div class="tag-cloud">
@foreach($recentTags as $tag)
<a href="{{ route('tag.show', $tag) }}">{{ $tag->name }}</a>
@endforeach
</div>
<h3>Archives</h3>
<ul>
@foreach($archivedMonths as $month => $count)
<li><a href="{{ route('archives', $month) }}">{{ $month }} ({{ $count }})</a></li>
@endforeach
</ul>
</div>
In this example, the SidebarComposer
populates the sidebar with popular categories, recent tags, and archive links. This data is automatically added to the sidebar view whenever it's included in a page, keeping your controllers clean and your sidebar content consistent across your application.
View composers in Laravel provide a powerful way to organize and reuse view logic across your application. By centralizing view-specific data preparation, you can create more maintainable, DRY code and ensure consistency across your views. Whether you're building complex dashboards, dynamic sidebars, or any feature requiring shared view data, view composers offer an elegant solution to streamline your Laravel application's view logic.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!