Streamlining View Logic with Blade Authorization Directives in Laravel
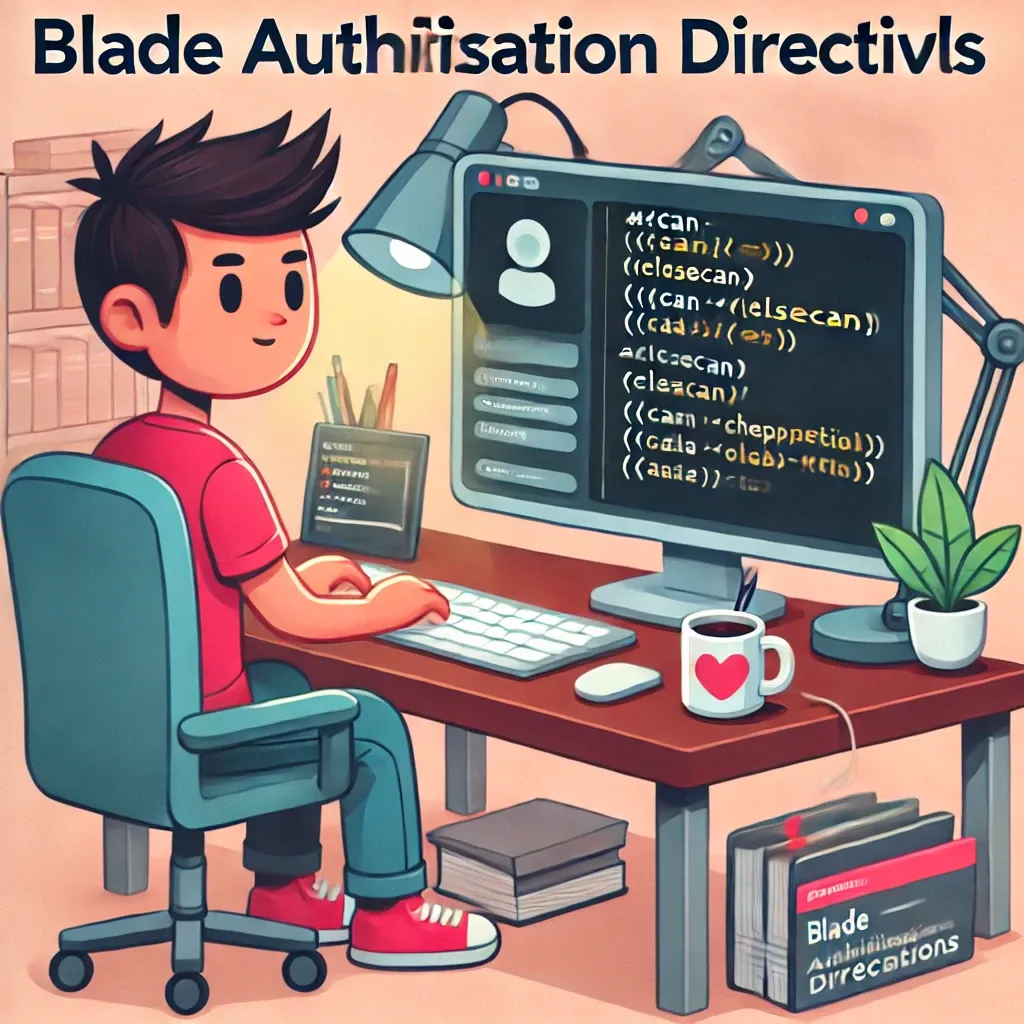
Laravel's Blade templating engine offers powerful directives for handling authorization directly in your views. These directives, namely @can
, @cannot
, and @canany
, allow you to conditionally display content based on user permissions, leading to cleaner and more secure templates.
Understanding Blade Authorization Directives
Blade authorization directives provide a clean syntax for performing authorization checks in your views. They work seamlessly with Laravel's authorization system, including policies and gates.
The @can and @cannot Directives
These directives allow you to check if a user is authorized to perform a specific action:
@can('update', $post)
<a href="{{ route('posts.edit', $post) }}">Edit Post</a>
@elsecan('create', App\Models\Post::class)
<a href="{{ route('posts.create') }}">Create New Post</a>
@else
<p>No permissions to edit or create posts.</p>
@endcan
@cannot('delete', $post)
<p>You can't delete this post.</p>
@endcannot
The @canany Directive
@canany
allows you to check if the user is authorized to perform any of a given set of actions:
@canany(['update', 'view', 'delete'], $post)
<div class="post-actions">
<!-- Post action buttons -->
</div>
@elsecanany(['create'], \App\Models\Post::class)
<a href="{{ route('posts.create') }}">Create New Post</a>
@endcanany
Practical Examples
• Displaying an admin panel link:
@can('access-admin-panel')
<li><a href="{{ route('admin.dashboard') }}">Admin Panel</a></li>
@endcan
• Conditionally showing edit buttons:
<h1>{{ $post->title }}</h1>
@can('update', $post)
<a href="{{ route('posts.edit', $post) }}" class="btn btn-primary">Edit</a>
@endcan
• Managing multiple permissions:
@canany(['moderate', 'admin'])
<div class="moderation-tools">
<!-- Moderation tools here -->
</div>
@endcanany
Using with Policies
Blade authorization directives work seamlessly with Laravel policies:
@can('update', $project)
<form action="{{ route('projects.update', $project) }}" method="POST">
@method('PUT')
@csrf
<!-- Form fields -->
<button type="submit">Update Project</button>
</form>
@endcan
Handling Guest Users
For guest users, you can use the @guest
directive in combination with authorization checks:
@guest
<a href="{{ route('login') }}">Login to edit posts</a>
@else
@can('edit', $post)
<a href="{{ route('posts.edit', $post) }}">Edit Post</a>
@endcan
@endguest
By using Blade authorization directives, you can:
• Keep your views clean and readable
• Ensure that sensitive UI elements are only shown to authorized users
• Maintain consistency between your backend authorization logic and frontend display
• Reduce the risk of exposing actions to unauthorized users
These directives provide a powerful tool for implementing view-level authorization in your Laravel applications, enhancing both security and user experience.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!