Streamlining Testing and Seeding with Laravel Model Factories
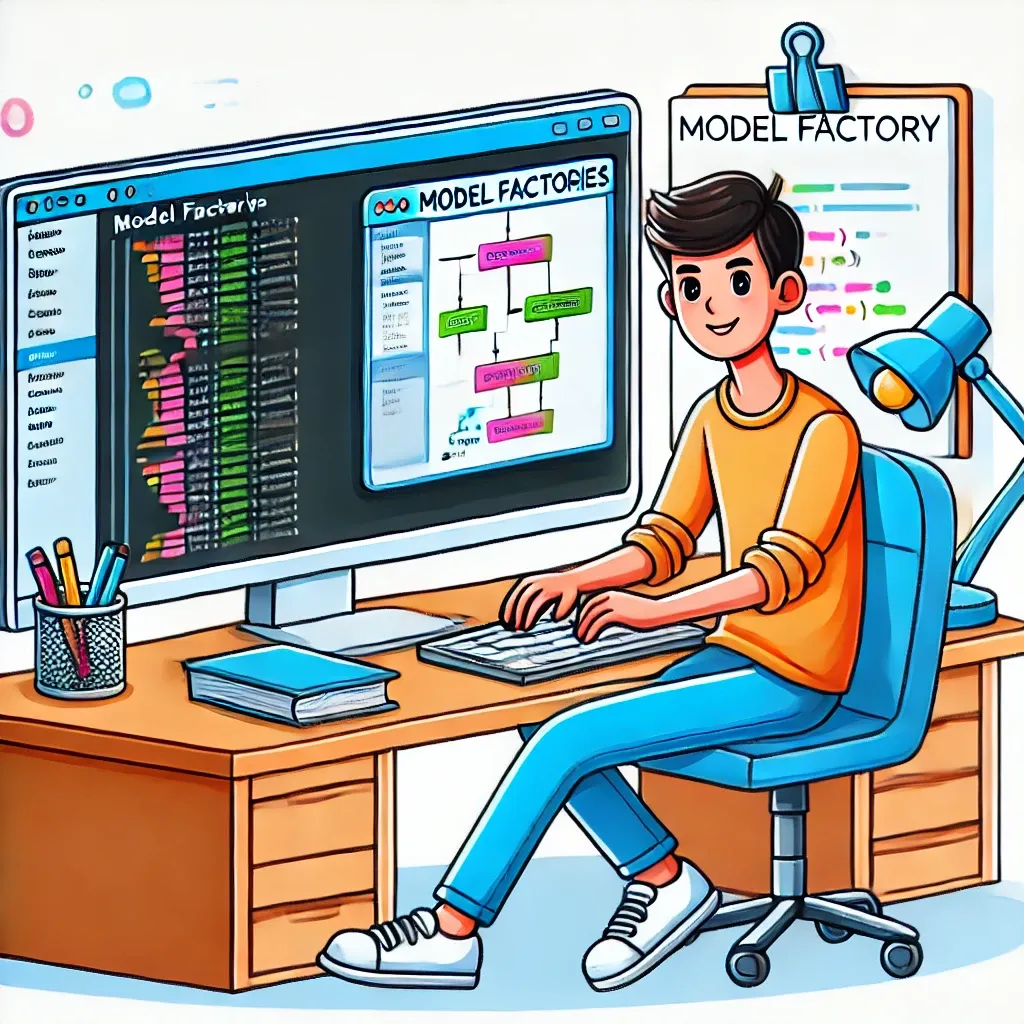
Laravel's model factories are a powerful tool for generating fake data, making testing and database seeding both efficient and consistent. Let's dive into how you can leverage model factories in your Laravel applications.
Basic Factory Definition
First, let's define a basic factory. In Laravel 8+, factories are defined as classes:
namespace Database\Factories;
use App\Models\User;
use Illuminate\Database\Eloquent\Factories\Factory;
use Illuminate\Support\Str;
class UserFactory extends Factory
{
protected $model = User::class;
public function definition()
{
return [
'name' => $this->faker->name,
'email' => $this->faker->unique()->safeEmail,
'email_verified_at' => now(),
'password' => bcrypt('password'),
'remember_token' => Str::random(10),
];
}
}
Using Factories in Tests
In your tests, you can create model instances easily:
public function test_user_can_create_post()
{
$user = User::factory()->create();
$response = $this->actingAs($user)->post('/posts', [
'title' => 'My First Post',
'content' => 'This is the content of my first post.',
]);
$response->assertStatus(201);
}
Creating Multiple Models
Need multiple instances? No problem:
$users = User::factory()->count(3)->create();
Customizing Attribute Values
You can override specific attributes:
$user = User::factory()->create([
'name' => 'John Doe',
]);
Factory States
States allow you to define discrete modifications to your factories:
class UserFactory extends Factory
{
// ...
public function admin()
{
return $this->state(function (array $attributes) {
return [
'role' => 'admin',
];
});
}
}
// Usage
$admin = User::factory()->admin()->create();
Relationships
Factories make it easy to create related models:
class PostFactory extends Factory
{
public function definition()
{
return [
'user_id' => User::factory(),
'title' => $this->faker->sentence,
'content' => $this->faker->paragraph,
];
}
}
// Create a user with three posts
$user = User::factory()
->has(Post::factory()->count(3))
->create();
Seeding the Database
Factories are great for seeding your database:
class DatabaseSeeder extends Seeder
{
public function run()
{
User::factory()->count(50)->create();
User::factory()
->count(10)
->has(Post::factory()->count(3))
->create();
}
}
Sequences
Use sequences when you need to cycle through a series of values:
$users = User::factory()
->count(10)
->sequence(fn ($sequence) => ['role' => $sequence->index % 2 ? 'user' : 'admin'])
->create();
After Making/Creating Callbacks
You can perform actions after a model is made or created:
User::factory()
->afterMaking(function (User $user) {
// ...
})
->afterCreating(function (User $user) {
$user->profile()->create([...]);
})
->create();
Using Factories with Real Data
Factories can be useful for populating fields with real data while filling in the gaps:
$realUser = [
'name' => 'Jane Doe',
'email' => 'jane@example.com',
];
$user = User::factory()->make($realUser);
Laravel's model factories provide a flexible, powerful way to generate test data and seed your database. By leveraging factories, you can write more robust tests, easily set up complex data scenarios, and ensure consistency across your testing and development environments.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!