Streamlining Tasks with Custom Artisan Commands in Laravel
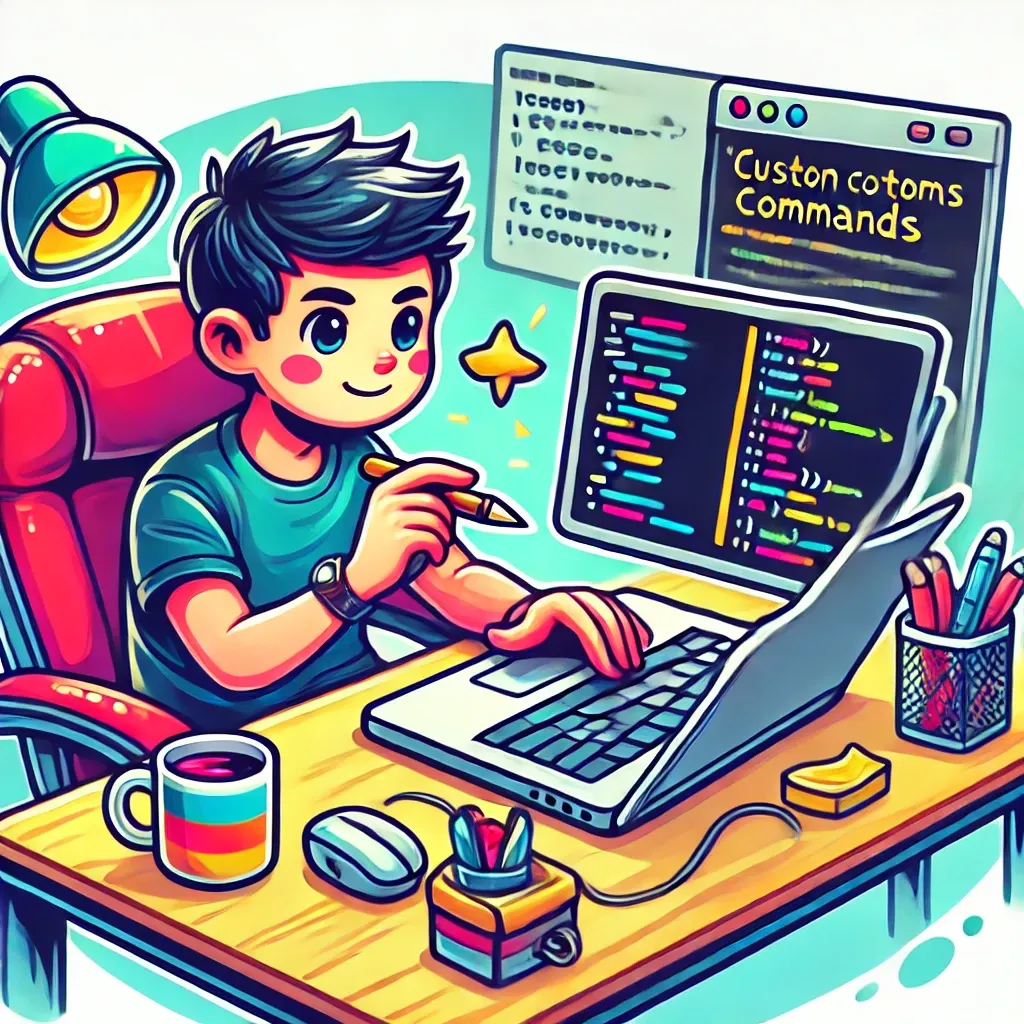
Laravel's Artisan console provides a powerful way to create custom commands for automating tasks in your application. Whether you need to perform data imports, run cleanups, or execute any application-specific operations, custom Artisan commands can significantly simplify these processes. Let's explore how to create and leverage custom commands effectively.
Creating a Custom Command
To create a new command, use the Artisan command:
php artisan make:command SendWeeklyNewsletter
This generates a new command class:
namespace App\Console\Commands;
use Illuminate\Console\Command;
class SendWeeklyNewsletter extends Command
{
protected $signature = 'newsletter:send {--queue}';
protected $description = 'Send the weekly newsletter to subscribers';
public function handle()
{
$job = new SendNewsletterJob();
$this->option('queue')
? dispatch($job)
: $job->handle();
$this->info('Newsletter dispatched successfully!');
}
}
Defining the Command Signature
The $signature
property defines your command's name and any arguments or options:
protected $signature = 'newsletter:send
{type : The type of newsletter to send}
{--queue : Whether the job should be queued}';
Handling Command Logic
The handle
method contains the command's logic:
public function handle()
{
$type = $this->argument('type');
$shouldQueue = $this->option('queue');
$job = new SendNewsletterJob($type);
$shouldQueue ? dispatch($job) : $job->handle();
$this->info("$type newsletter dispatched successfully!");
}
Prompting for Input
You can interact with the user for additional input:
public function handle()
{
$name = $this->ask('What is your name?');
$password = $this->secret('What is the password?');
if ($this->confirm('Do you wish to continue?')) {
// Proceed with operation
}
}
Displaying Output
Use various methods to display output:
$this->info('Operation successful!');
$this->error('Something went wrong.');
$this->line('Processing...');
$this->table(
['Name', 'Email'],
[
['John Doe', 'john@example.com'],
['Jane Doe', 'jane@example.com'],
]
);
$this->progressBar(100);
Registering the Command
Register your command in app/Console/Kernel.php
:
protected $commands = [
\App\Console\Commands\SendWeeklyNewsletter::class,
];
Scheduling Commands
To automate command execution, use Laravel's scheduler in app/Console/Kernel.php
:
protected function schedule(Schedule $schedule)
{
$schedule->command('newsletter:send --queue')
->weekly()
->mondays()
->at('8:00');
}
Calling Other Commands
You can call other Artisan commands from within your command:
public function handle()
{
$this->call('cache:clear');
$this->callSilent('email:send', [
'user' => 1, '--queue' => 'default'
]);
}
Testing Commands
Laravel provides tools to test your custom commands:
public function test_newsletter_command()
{
$this->artisan('newsletter:send', ['type' => 'weekly'])
->expectsOutput('weekly newsletter dispatched successfully!')
->assertExitCode(0);
}
Dependency Injection
You can use Laravel's service container to inject dependencies:
public function handle(NewsletterService $newsletter)
{
$newsletter->send();
}
Custom Artisan commands in Laravel offer a powerful way to automate tasks, improve developer workflows, and manage application-specific operations. By leveraging these commands, you can create more maintainable, efficient, and automated Laravel applications. Whether you're running complex data processing tasks or simple maintenance operations, custom Artisan commands provide the flexibility and power to streamline your development process.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!