Streamlining Request Handling in Laravel with whenHas()
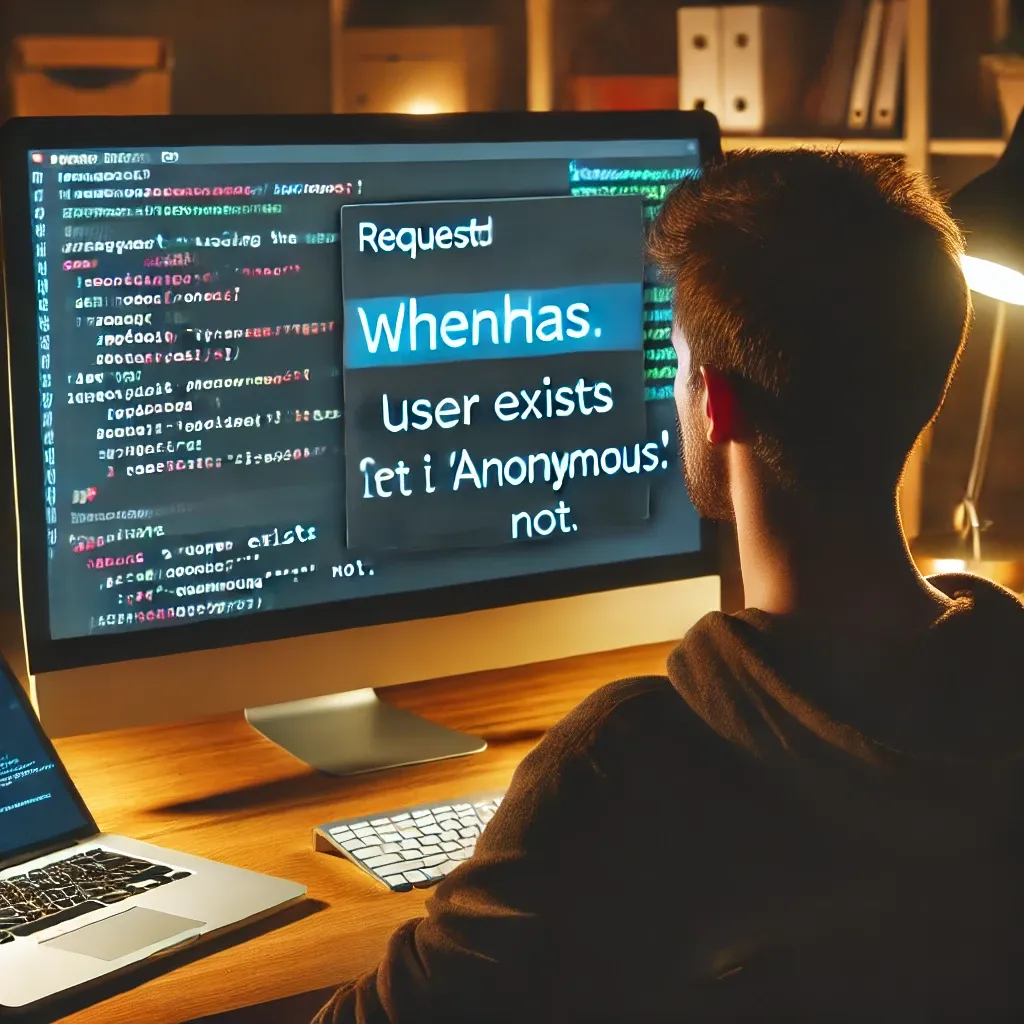
In the world of web development, handling optional input fields efficiently can sometimes be a challenge. Laravel, always at the forefront of developer experience, offers a elegant solution with the whenHas
method on the request object. This method allows you to execute code conditionally based on the presence of an input value. Let's dive into how this feature can enhance your Laravel applications.
Understanding $request->whenHas()
The whenHas
method on the request object allows you to execute a closure if a specified value is present in the request. It can also execute an alternative closure if the value is not present. Here's the basic syntax:
$request->whenHas('input_name', function ($value) {
// This runs when the input is present
}, function () {
// This runs when the input is not present (optional)
});
Real-Life Example
Let's consider a scenario where we're updating a user's profile. We want to update the user's name if it's provided in the request, otherwise set it to a default value.
Here's how we might implement this using whenHas
:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function updateProfile(Request $request, User $user)
{
$request->whenHas('name',
function ($name) use ($user) {
$user->update(['name' => $name]);
},
function () use ($user) {
$user->update(['name' => 'Anonymous User']);
}
);
$request->whenHas('email',
function ($email) use ($user) {
$user->update(['email' => $email]);
}
);
return response()->json($user);
}
}
In this example, we're using whenHas
to:
- Update the user's name if it's provided, otherwise set it to 'Anonymous User'
- Update the user's email only if it's provided in the request
Here's what the input and output might look like:
// PATCH /api/users/1
// Input
{
"name": "John Doe"
}
// Output
{
"id": 1,
"name": "John Doe",
"email": "john@example.com",
"updated_at": "2023-06-15T16:30:00.000000Z"
}
// Input (no name provided)
{
"email": "newemail@example.com"
}
// Output
{
"id": 1,
"name": "Anonymous User",
"email": "newemail@example.com",
"updated_at": "2023-06-15T16:35:00.000000Z"
}
Remember, while whenHas
is powerful for simple presence checks, for more complex conditional logic or when you need to perform operations based on the actual value of the input (not just its presence), you might still need to use traditional if statements or switch to other Laravel features like form requests or custom request classes.
If you found this tip helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel gems!