Streamlining Redirects in Laravel Livewire with redirectRoute()
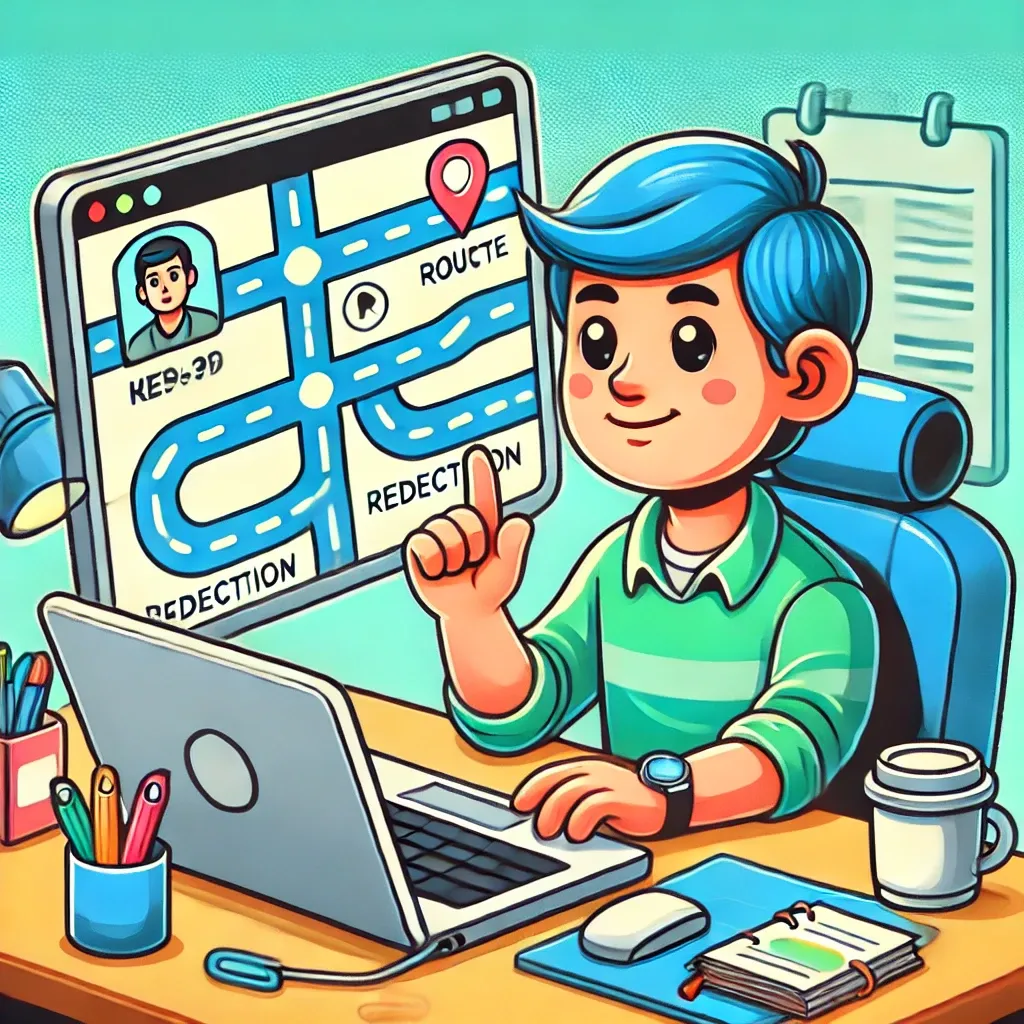
As Livewire developers, we often need to redirect users after completing an action. While Livewire provides several ways to handle redirects, the redirectRoute() method stands out for its simplicity and type safety. In this post, we'll explore how to use redirectRoute() to create cleaner, more maintainable code in your Livewire components.
Understanding redirectRoute()
The redirectRoute() method is a Livewire helper that allows you to redirect to a named route after an action. It provides a cleaner syntax compared to traditional redirect methods and offers the advantage of early error detection for invalid route names or parameters.
Basic Usage of redirectRoute()
Let's start with a basic example:
class CreatePost extends Component
{
public $title;
public $content;
public function save()
{
$post = Post::create($this->validate([
'title' => 'required|string|max:255',
'content' => 'required|string',
]));
return $this->redirectRoute('posts.show', ['post' => $post->id]);
}
}
In this example:
- After creating a new post, we redirect to the 'posts.show' route.
- The post's ID is passed as a parameter to the route.
Advantages of redirectRoute()
- Clean Syntax: It's more readable than constructing redirect responses manually.
- Type Safety: Invalid route names will throw exceptions early, helping catch errors during development.
- Consistency: Encourages the use of named routes throughout your application.
Advanced Usage
Passing Multiple Parameters
You can easily pass multiple parameters to your routes:
return $this->redirectRoute('admin.users.posts', ['userId' => $user->id, 'postId' => $post->id]);
Using with Query Parameters
You can include query parameters in your redirect:
return $this->redirectRoute('search', ['query' => $searchTerm], ['page' => 1, 'sort' => 'relevance']);
Combining with Flash Messages
redirectRoute() works seamlessly with Laravel's flash messaging:
public function update()
{
// Update logic here
session()->flash('message', 'Profile updated successfully!');
return $this->redirectRoute('profile.show');
}
Real-World Example: E-commerce Order Process
Let's consider a more complex scenario of an e-commerce order process:
class CheckoutComponent extends Component
{
public $cart;
public $shippingAddress;
public $paymentMethod;
public function processOrder()
{
$this->validate([
'shippingAddress' => 'required|string',
'paymentMethod' => 'required|in:credit_card,paypal',
]);
try {
$order = Order::create([
'user_id' => auth()->id(),
'total' => $this->cart->total(),
'shipping_address' => $this->shippingAddress,
'payment_method' => $this->paymentMethod,
]);
$this->cart->clear();
session()->flash('order_completed', true);
return $this->redirectRoute('orders.confirmation', ['order' => $order->id]);
} catch (\Exception $e) {
session()->flash('error', 'There was an error processing your order.');
return $this->redirectRoute('checkout.error');
}
}
}
In this example:
- After successfully creating an order, we redirect to the order confirmation page.
- If there's an error, we redirect to an error page.
- We're using session flashing to pass messages along with the redirects.
Best Practices
- Use Named Routes: Always use named routes in your application to take full advantage of redirectRoute().
- Group Related Routes: Use route groups and prefixes to organize your routes, making them easier to manage in redirects.
- Handle Errors Gracefully: Use try-catch blocks to handle potential errors and redirect users appropriately.
- Combine with Authorization: Use redirectRoute() in combination with Laravel's authorization features to redirect unauthorized users.
- Keep Controllers Thin: Use redirectRoute() in your Livewire components to handle redirects, keeping your controllers focused on HTTP-related tasks.
Conclusion
Livewire's redirectRoute() method offers a clean, type-safe way to handle redirects in your components. By leveraging this feature, you can create more readable and maintainable code, catch routing-related errors early, and streamline your redirect logic. Whether you're building a simple CRUD application or a complex e-commerce platform, mastering redirectRoute() will help you create more robust and user-friendly Livewire applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!