Streamlining Object Creation and Modification with Laravel's with() Helper
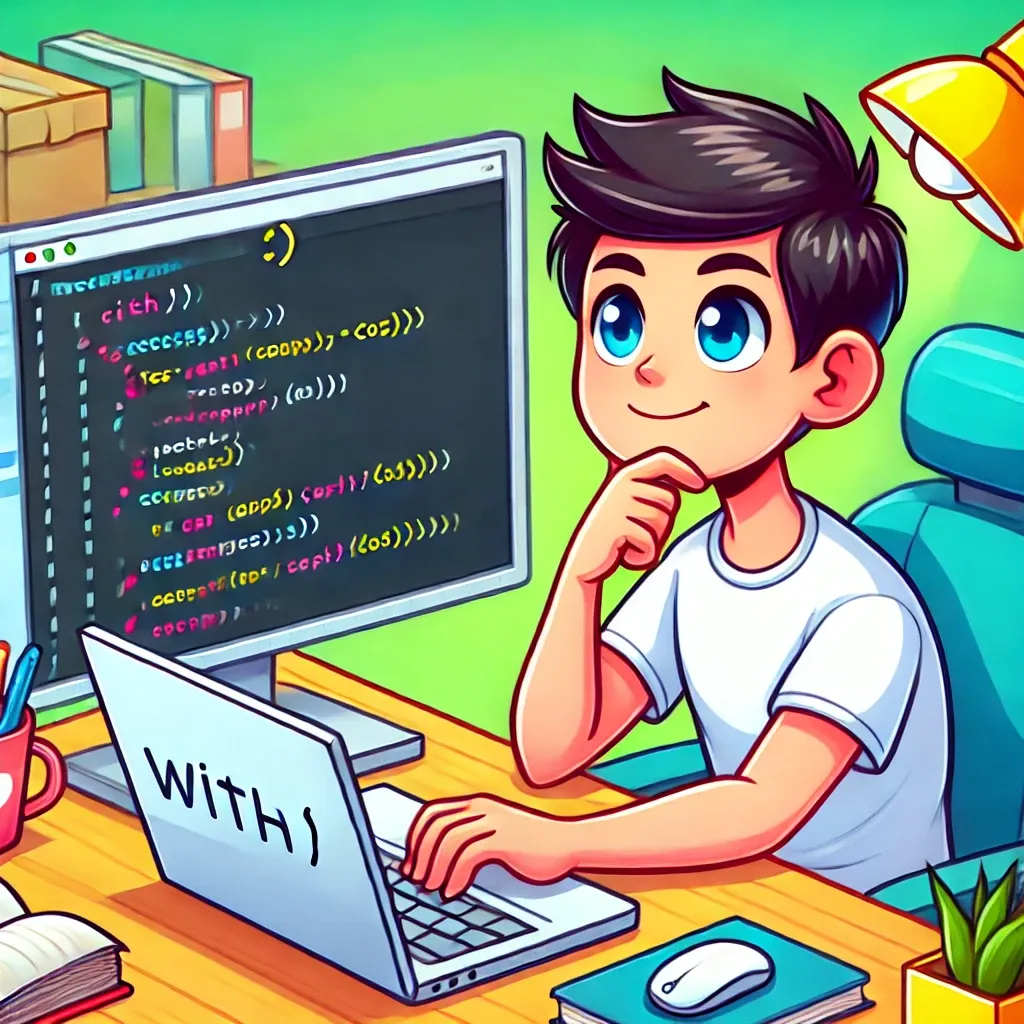
Laravel's with()
helper is a simple yet powerful function that allows developers to create and modify objects in a single, fluent operation. This helper can significantly improve code readability and reduce the number of lines needed for object setup. Let's explore how to leverage this useful helper in your Laravel projects.
Basic Usage of with()
The with()
helper takes two arguments: an object (or value) and a closure. It applies the closure to the object and then returns the resulting object. Here's a simple example:
$user = with(new User, function ($user) {
$user->name = 'John Doe';
$user->email = 'john@example.com';
return $user;
});
This creates a new User
object, sets its name and email properties, and returns the modified object.
Simplifying Complex Object Setup
The with()
helper shines when setting up complex objects:
$response = with(new Response('Hello'), function ($response) {
return $response->header('X-Custom', 'Value')
->cookie('name', 'value', 60);
});
This creates a new Response
object, adds a custom header and a cookie, all in one fluent operation.
Using with() in Model Factories
The with()
helper can be particularly useful in model factories:
use App\Models\Post;
class PostFactory extends Factory
{
public function definition()
{
return [
'title' => $this->faker->sentence,
'content' => $this->faker->paragraphs(3, true),
'published_at' => $this->faker->dateTimeBetween('-1 year', 'now'),
];
}
public function published()
{
return $this->state(function (array $attributes) {
return with($attributes, function (&$attrs) {
$attrs['published_at'] = now();
$attrs['is_published'] = true;
return $attrs;
});
});
}
}
Here, with()
is used to modify the attributes array in a published post state.
Conditional Object Modification
You can use with()
for conditional object modification:
$user = with(new User, function ($user) use ($request) {
$user->name = $request->name;
$user->email = $request->email;
if ($request->has('role')) {
$user->role = $request->role;
}
return $user;
});
This setup allows for flexible object creation based on input data.
Creating Test Data
The with()
helper is excellent for creating test data:
public function testUserRegistration()
{
$userData = with([], function ($data) {
$data['name'] = 'Test User';
$data['email'] = 'test@example.com';
$data['password'] = 'password';
$data['password_confirmation'] = 'password';
return $data;
});
$response = $this->post('/register', $userData);
$response->assertRedirect('/home');
$this->assertDatabaseHas('users', ['email' => 'test@example.com']);
}
This approach keeps your test setup clean and readable.
Real-World Example: Configuring a Third-Party API Client
Here's how you might use with()
to set up a complex API client:
use ThirdParty\ApiClient;
$client = with(new ApiClient, function ($client) use ($config) {
$client->setApiKey($config['api_key'])
->setBaseUrl($config['base_url'])
->setVersion($config['version'])
->setRetryPolicy([
'times' => 3,
'sleep' => 1000,
])
->setLogger(app('log'))
->setCache(app('cache')->store('redis'));
if ($config['debug']) {
$client->enableDebugging();
}
return $client;
});
This example demonstrates how with()
can make complex object setup more readable and maintainable.
Performance Considerations
While with()
is convenient, it's important to note that it doesn't provide any performance benefits over traditional object creation and modification. Its primary advantage is in code readability and organization.
The with()
helper in Laravel is a versatile tool that can significantly improve the readability and organization of your code, especially when dealing with complex object setup or modification. By allowing you to create and configure objects in a single, fluent operation, it promotes cleaner, more expressive code. Whether you're setting up test data, configuring complex objects, or simplifying your factories, the with()
helper is a valuable addition to your Laravel toolkit.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!