Streamlining Laravel Middleware: The Power of Aliases
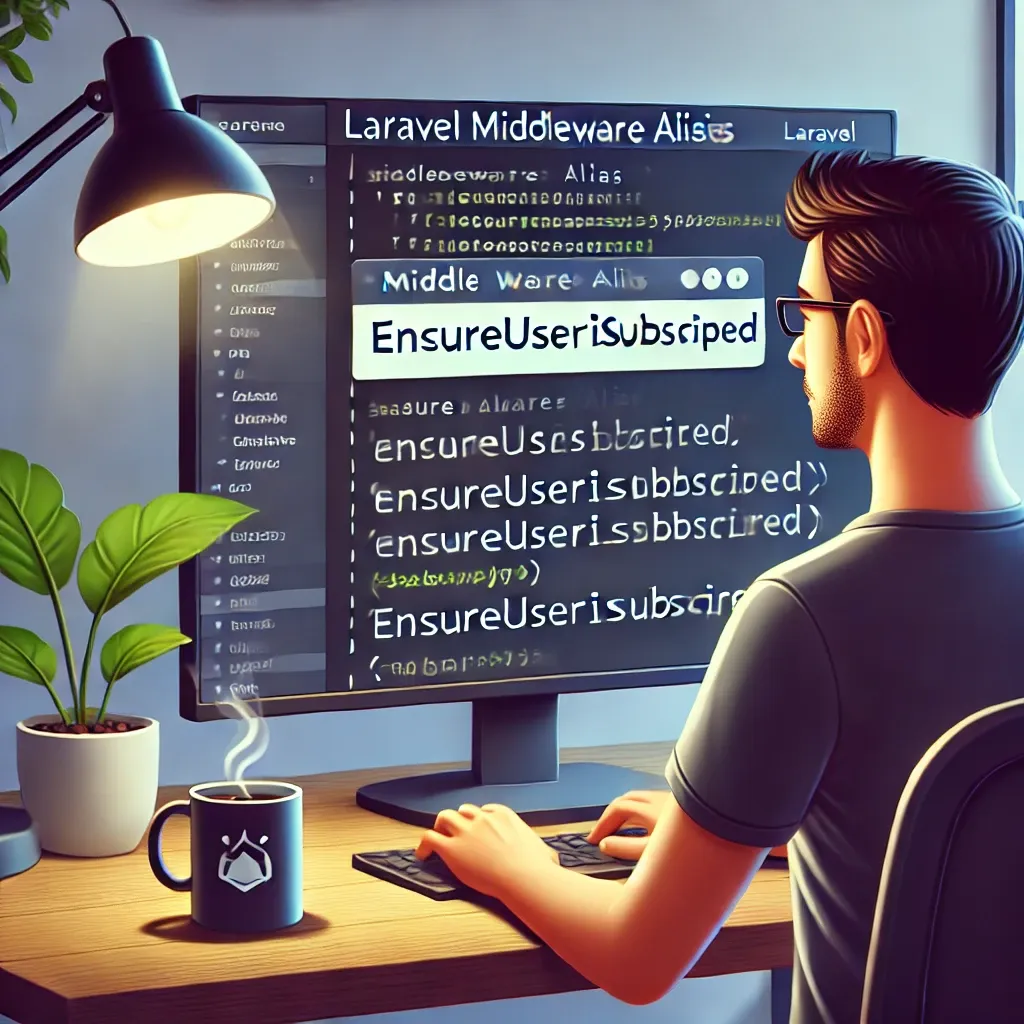
When building complex Laravel applications, you often find yourself using middleware with long, descriptive class names. While these names are great for understanding what the middleware does, they can clutter your route definitions. Enter middleware aliases - a powerful feature that allows you to assign short, memorable names to your middleware. Let's dive into how to use this feature effectively.
Understanding Middleware Aliases
Middleware aliases in Laravel allow you to define short aliases for your middleware classes. This is especially useful for middleware with long class names, improving the readability of your route definitions.
Setting Up Middleware Aliases
To set up middleware aliases, you'll need to modify your application's bootstrap/app.php
file. Here's how you can do it:
use App\Http\Middleware\EnsureUserIsSubscribed;
->withMiddleware(function (Middleware $middleware) {
$middleware->alias([
'subscribed' => EnsureUserIsSubscribed::class
]);
})
In this example, we're creating an alias 'subscribed' for the EnsureUserIsSubscribed
middleware.
Using Middleware Aliases
Once you've defined your aliases, you can use them when assigning middleware to routes:
Route::get('/profile', function () {
// ...
})->middleware('subscribed');
This is much cleaner and more readable than using the full class name.
Real-Life Example
Let's consider a scenario where we're building a SaaS application with various middleware for different subscription tiers and authentication methods. We'll set up aliases for these middleware and use them in our routes.
First, let's define our aliases in bootstrap/app.php
:
use App\Http\Middleware\EnsureUserIsSubscribed;
use App\Http\Middleware\EnsureUserHasTeamAccess;
use App\Http\Middleware\AuthenticateWithApiKey;
->withMiddleware(function (Middleware $middleware) {
$middleware->alias([
'subscribed' => EnsureUserIsSubscribed::class,
'team.access' => EnsureUserHasTeamAccess::class,
'api.auth' => AuthenticateWithApiKey::class,
]);
})
Now, let's use these aliases in our routes:
// In routes/web.php
Route::middleware(['subscribed', 'team.access'])->group(function () {
Route::get('/dashboard', [DashboardController::class, 'index']);
Route::get('/team', [TeamController::class, 'index']);
});
// In routes/api.php
Route::middleware(['api.auth'])->group(function () {
Route::get('/user', [UserController::class, 'show']);
Route::post('/data', [DataController::class, 'store']);
});
Here's how this might look in action:
// GET /dashboard
// If user is not subscribed:
{
"error": "Subscription required",
"code": 403
}
// If user is subscribed but doesn't have team access:
{
"error": "Team access required",
"code": 403
}
// If all checks pass:
{
"view": "dashboard",
"data": {
"user": {
"name": "John Doe",
"subscription": "pro"
},
"teamCount": 3
}
}
// GET /api/user with invalid API key
{
"error": "Invalid API key",
"code": 401
}
// GET /api/user with valid API key
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
By using middleware aliases, we've made our route definitions much cleaner and more readable. This becomes especially valuable as your application grows and you have more complex middleware stacks.
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!