Streamlining Laravel Middleware: Mastering Middleware Groups
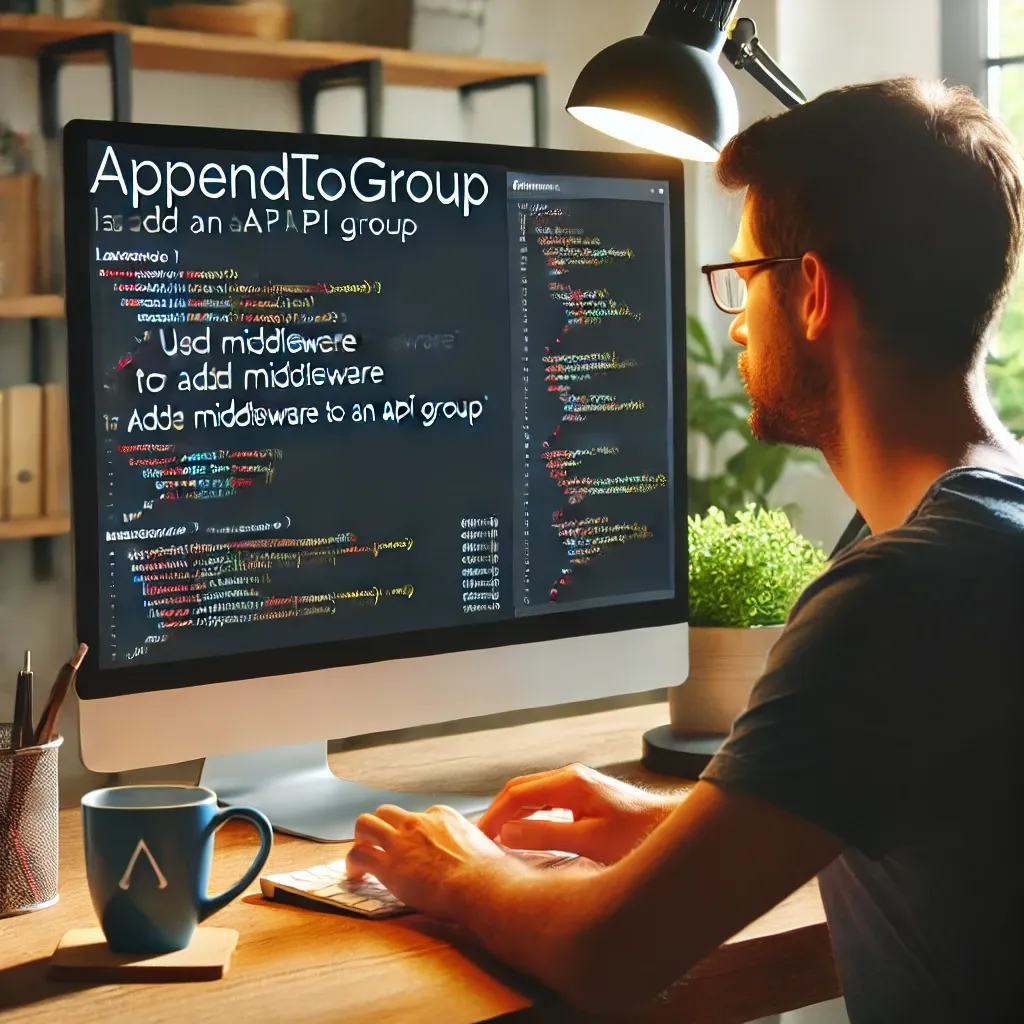
When building complex applications with Laravel, managing multiple middleware can become challenging. Laravel's middleware groups feature provides an elegant solution to organize and apply multiple middleware efficiently. In this post, we'll explore how to leverage middleware groups to keep your route definitions clean and your application structure organized.
Understanding Middleware Groups
Middleware groups in Laravel allow you to bundle several middleware under a single key. This makes it easier to assign multiple middleware to routes or route groups without cluttering your route definitions.
Setting Up Middleware Groups
To create a middleware group, you'll use the appendToGroup
or prependToGroup
method in your application's bootstrap/app.php
file. Here's how you can set it up:
use App\Http\Middleware\First;
use App\Http\Middleware\Second;
->withMiddleware(function (Middleware $middleware) {
$middleware->appendToGroup('api', [
First::class,
Second::class,
]);
$middleware->prependToGroup('web', [
First::class,
Second::class,
]);
})
In this example, we're creating two middleware groups: 'api' and 'web'. The appendToGroup
method adds middleware to the end of the group, while prependToGroup
adds them to the beginning.
Using Middleware Groups
Once you've defined your middleware groups, you can assign them to routes or route groups using the same syntax as individual middleware:
Route::get('/', function () {
// ...
})->middleware('api');
Route::middleware(['web'])->group(function () {
// ...
});
Real-Life Example
Let's consider a scenario where we have an API that requires authentication, rate limiting, and CORS handling. We can group these middleware for easier management:
use App\Http\Middleware\Authenticate;
use App\Http\Middleware\ThrottleRequests;
use Fruitcake\Cors\HandleCors;
->withMiddleware(function (Middleware $middleware) {
$middleware->appendToGroup('api', [
Authenticate::class,
ThrottleRequests::class.':api',
HandleCors::class,
]);
})
Now, we can apply this group to our API routes:
Route::prefix('api')->middleware(['api'])->group(function () {
Route::get('/user', function (Request $request) {
return $request->user();
});
Route::get('/posts', [PostController::class, 'index']);
});
When a request is made to any of these API routes, it will go through the authentication, rate limiting, and CORS middleware in order. Here's what the process looks like:
// Request to /api/user
// 1. Authenticate middleware
// If not authenticated:
{
"message": "Unauthenticated."
}
// 2. ThrottleRequests middleware
// If too many requests:
{
"message": "Too Many Attempts."
}
// 3. HandleCors middleware
// Adds appropriate CORS headers to response
// If all middleware pass:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
If you found this guide helpful, don't forget to subscribe to my daily newsletter and follow me on X/Twitter for more Laravel tips and tricks!