Streamlining Laravel API Responses: Disabling Resource Wrapping
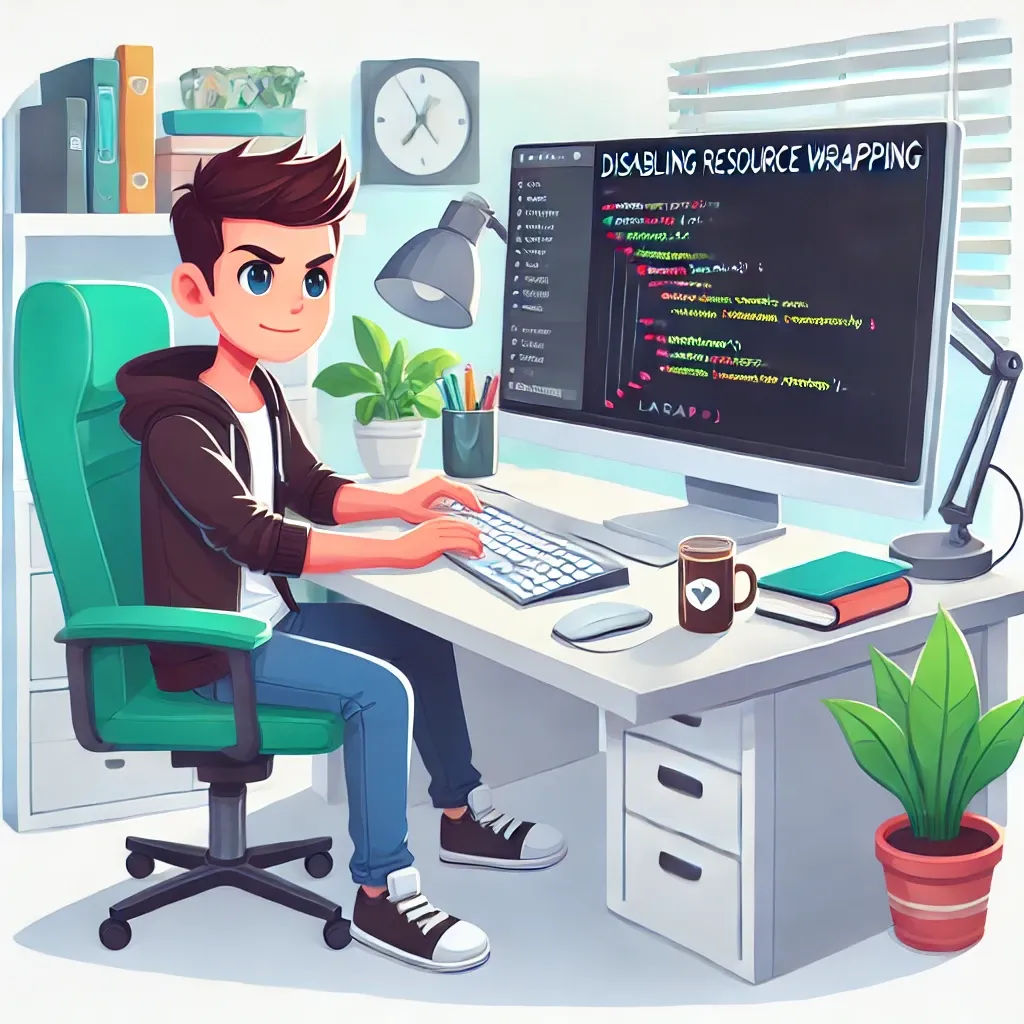
When building APIs with Laravel, you often use API resources to transform your models into JSON responses. By default, Laravel wraps your outermost resource in a data
key. While this is useful in many scenarios, there are times when you might want a flatter response structure. Let's explore how to disable this wrapping and customize your API responses.
Default Behavior
Typically, when you return a resource or resource collection from a Laravel route, it's wrapped in a data
key. For example:
Route::get('/users', function () {
return UserResource::collection(User::all());
});
This might result in a response like:
{
"data": [
{ "id": 1, "name": "Alice" },
{ "id": 2, "name": "Bob" }
]
}
Disabling the Wrapping
Laravel provides a straightforward way to disable this wrapping globally. You can do this by calling the withoutWrapping
method on the base JsonResource
class. This is typically done in a service provider.
Here's how you can implement this in your AppServiceProvider
:
<?php
namespace App\Providers;
use Illuminate\Http\Resources\Json\JsonResource;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function boot(): void
{
JsonResource::withoutWrapping();
}
}
Now, your API responses will no longer include the data
wrapper:
[
{ "id": 1, "name": "Alice" },
{ "id": 2, "name": "Bob" }
]
Selective Wrapping
It's worth noting that the withoutWrapping
method only affects the outermost resource. If you've manually added data
keys to your own resource collections, those will remain intact.
Real-World Scenario
Consider a scenario where you're building an API for a blog platform. You might have resources for posts, comments, and authors. Here's how you could structure these without the default wrapping:
class PostResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'title' => $this->title,
'content' => $this->content,
'author' => new AuthorResource($this->author),
'comments' => CommentResource::collection($this->comments),
];
}
}
class AuthorResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'name' => $this->name,
];
}
}
class CommentResource extends JsonResource
{
public function toArray($request)
{
return [
'id' => $this->id,
'content' => $this->content,
'user' => $this->user->name,
];
}
}
With wrapping disabled, a request to fetch a post might return:
{
"id": 1,
"title": "First Post",
"content": "This is the content of the first post.",
"author": {
"id": 1,
"name": "John Doe"
},
"comments": [
{
"id": 1,
"content": "Great post!",
"user": "Jane Smith"
},
{
"id": 2,
"content": "Thanks for sharing.",
"user": "Bob Johnson"
}
]
}
This flatter structure can be more intuitive and easier to work with for API consumers.
Considerations
While disabling wrapping can lead to cleaner, flatter API responses, it's important to consider consistency across your API. If you're building a public API or working with external clients, make sure to document this behavior clearly.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!