Streamlining File Downloads with Laravel's Storage::download Method
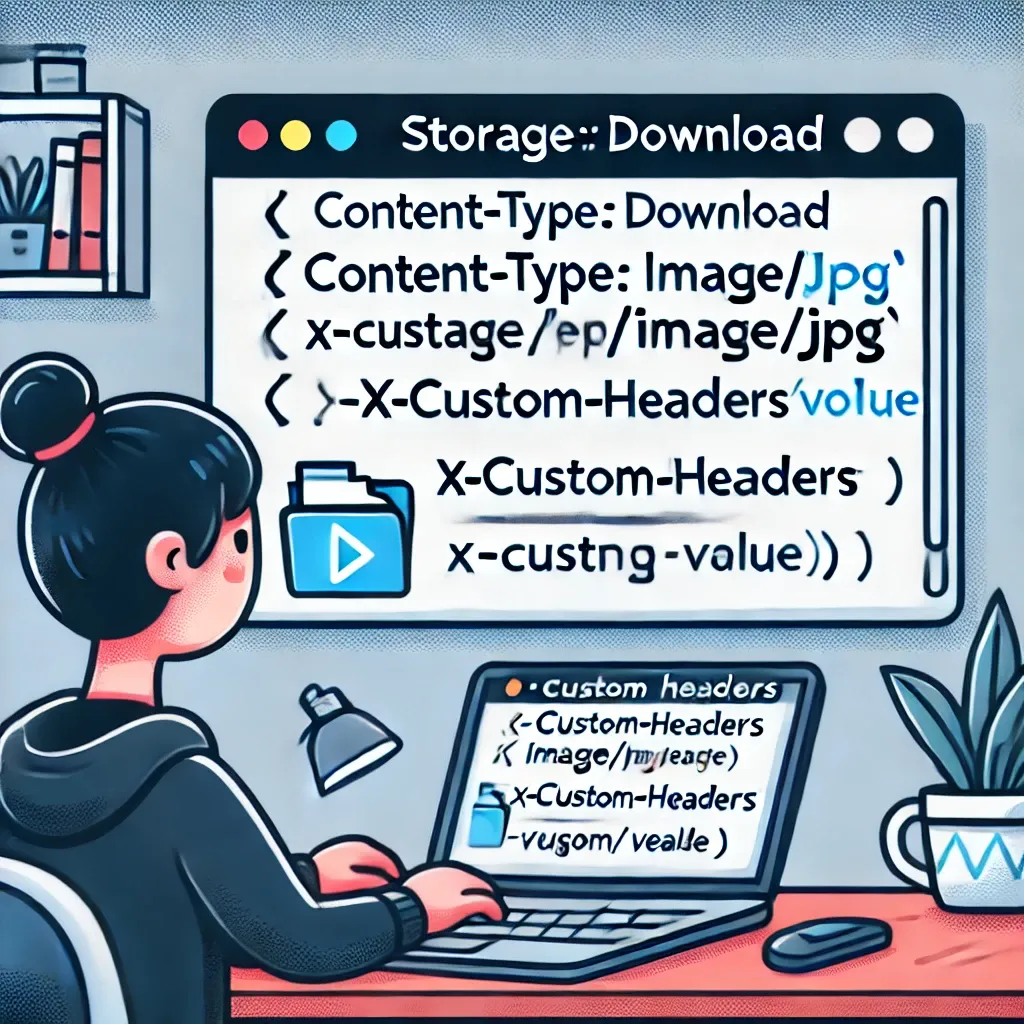
In web applications, handling file downloads is a common requirement. Laravel simplifies this process with its Storage::download
method, providing a clean and secure way to serve files to users. Let's explore how to leverage this powerful feature in your Laravel applications.
Understanding Storage::download
The Storage::download
method is part of Laravel's filesystem abstraction. It allows you to generate a response that will force the user's browser to download the file at a given path. This method is versatile, allowing you to customize the download filename and even add custom headers.
Basic Usage
Here's a simple example of how to use Storage::download
:
use Illuminate\Support\Facades\Storage;
public function downloadFile($filename)
{
return Storage::download("files/{$filename}");
}
This will prompt the user to download the file with its original filename.
Advanced Usage
You can customize the download by specifying a name and adding headers:
return Storage::download('files/report.pdf', 'annual_report_2023.pdf', [
'Content-Type' => 'application/pdf',
'Cache-Control' => 'no-cache, no-store, must-revalidate',
]);
This example changes the download name and adds custom headers for content type and caching behavior.
Practical Applications
Secure File Downloads
For files that require authorization:
public function downloadSecureFile(Request $request, $fileId)
{
$file = SecureFile::findOrFail($fileId);
if ($request->user()->cannot('download', $file)) {
abort(403);
}
return Storage::download($file->path, $file->original_name);
}
Dynamic File Generation
You can generate files on-the-fly and offer them for download:
use Illuminate\Support\Facades\Storage;
use PDF;
public function downloadInvoice(Order $order)
{
$pdf = PDF::loadView('invoices.template', ['order' => $order]);
Storage::put("invoices/{$order->id}.pdf", $pdf->output());
return Storage::download("invoices/{$order->id}.pdf", "Invoice-{$order->number}.pdf");
}
Temporary URL Downloads
For large files or when you want to provide a temporary download link:
use Illuminate\Support\Facades\Storage;
public function getDownloadLink($fileId)
{
$file = File::findOrFail($fileId);
$url = Storage::temporaryUrl(
$file->path,
now()->addMinutes(5),
[
'ResponseContentType' => 'application/octet-stream',
'ResponseContentDisposition' => 'attachment; filename="' . $file->original_name . '"',
]
);
return response()->json(['download_url' => $url]);
}
This generates a temporary URL that expires after 5 minutes.
Error Handling
It's important to handle cases where the file might not exist:
public function downloadFile($filename)
{
$path = "files/{$filename}";
if (!Storage::exists($path)) {
abort(404, 'File not found');
}
return Storage::download($path);
}
Laravel's Storage::download
method provides a powerful and flexible way to handle file downloads in your applications. Whether you're serving user-uploaded files, dynamically generated documents, or large data sets, this method offers a clean and secure solution. By leveraging Laravel's filesystem abstraction, you can easily manage downloads across different storage drivers, making your code more portable and maintainable.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!