Streamlining Authorization with Inline Checks in Laravel
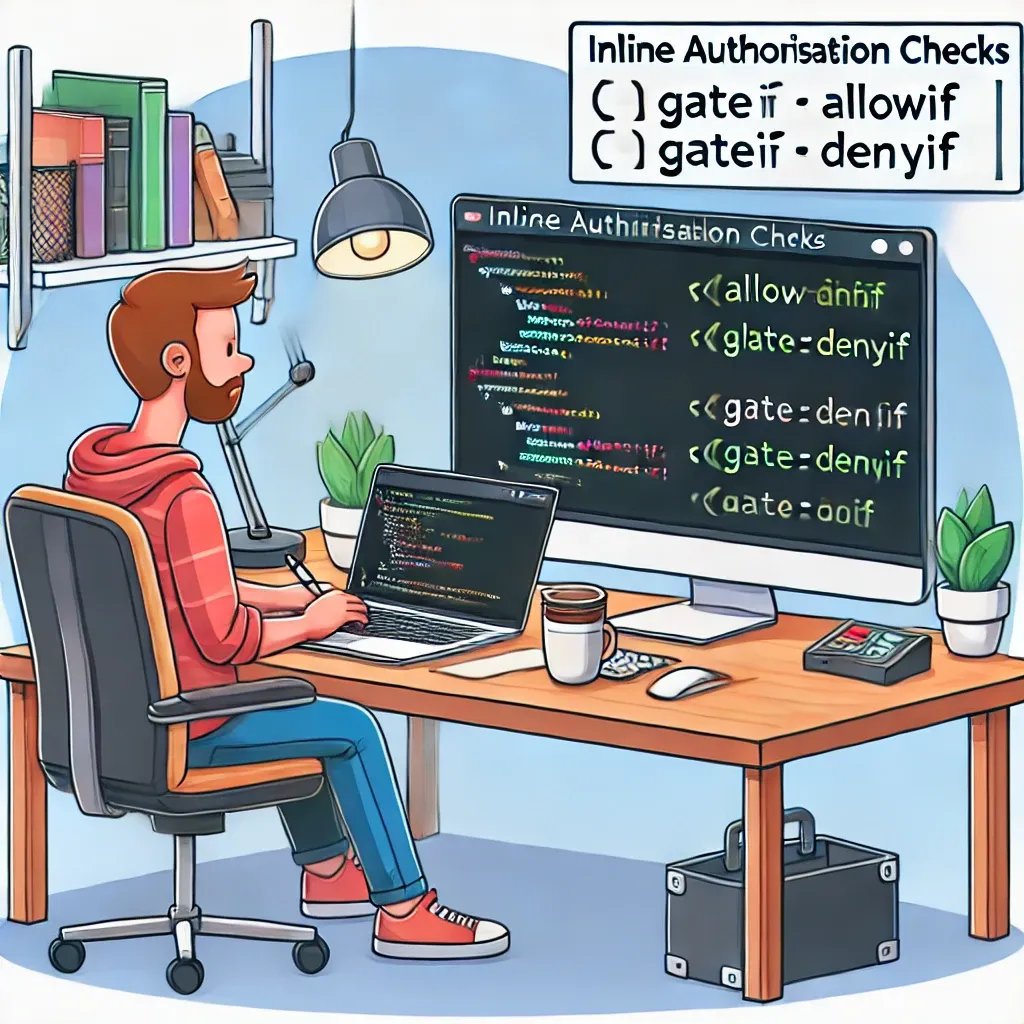
Laravel's authorization system is powerful and flexible, but sometimes you need a quick, simple way to perform authorization checks without defining a full gate. This is where inline authorization checks come in handy. Let's explore how to use Gate::allowIf()
and Gate::denyIf()
to streamline your authorization logic.
Understanding Inline Authorization
Inline authorization allows you to perform quick authorization checks without the need to define dedicated gates. This can be particularly useful for one-off checks or when you want to keep your authorization logic close to where it's used.
Using Gate::allowIf()
Gate::allowIf()
allows you to define a condition that, if met, will authorize the action. Here's a basic example:
use Illuminate\Support\Facades\Gate;
Gate::allowIf(fn ($user) => $user->isAdministrator());
In this case, if the user is an administrator, they will be authorized. If not, Laravel will throw an AuthorizationException
.
Using Gate::denyIf()
Conversely, Gate::denyIf()
allows you to define a condition that, if met, will deny the action:
Gate::denyIf(fn ($user) => $user->isBanned());
Here, if the user is banned, access will be denied and an AuthorizationException
will be thrown.
Practical Examples
Let's look at some practical ways to use these methods:
• Authorizing based on user roles:
Gate::allowIf(fn ($user) => $user->role === 'editor' || $user->role === 'admin');
• Denying access based on account status:
Gate::denyIf(fn ($user) => $user->account_status === 'suspended');
• Allowing access based on ownership:
Gate::allowIf(fn ($user, $post) => $user->id === $post->user_id);
Handling Exceptions
When using these methods, it's important to remember that they will throw an AuthorizationException
if the check fails. You can handle this exception in your app/Exceptions/Handler.php
file or catch it directly:
try {
Gate::allowIf(fn ($user) => $user->isVerified());
// Perform action for verified users
} catch (AuthorizationException $e) {
return response()->json(['error' => 'You must verify your account first.'], 403);
}
Combining with Other Authorization Methods
Inline authorization checks can be combined with Laravel's other authorization methods for more complex scenarios:
if (Gate::allows('edit-settings') && Gate::allowIf(fn ($user) => $user->isActiveSubscriber())) {
// User can edit settings and is an active subscriber
}
Use Cases
• Quick permission checks in controllers:
public function update(Request $request, Post $post)
{
Gate::allowIf(fn ($user) => $user->can('update', $post));
// Update post...
}
• Conditional access in blade templates:
@php
Gate::allowIf(fn ($user) => $user->hasPermission('view-dashboard'))
@endphp
<div>
<!-- Dashboard content -->
</div>
• API endpoint authorization:
Route::get('/api/sensitive-data', function () {
Gate::allowIf(fn ($user) => $user->tokenCan('read:sensitive-data'));
return SensitiveData::all();
});
Inline authorization checks with Gate::allowIf()
and Gate::denyIf()
provide a clean, expressive way to handle simple authorization logic in your Laravel applications. They're particularly useful for quick checks that don't warrant a full gate definition, helping you keep your code concise and your authorization logic close to where it's needed.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!