Streamlining API Responses: Conditional Attribute Merging in Laravel Resources
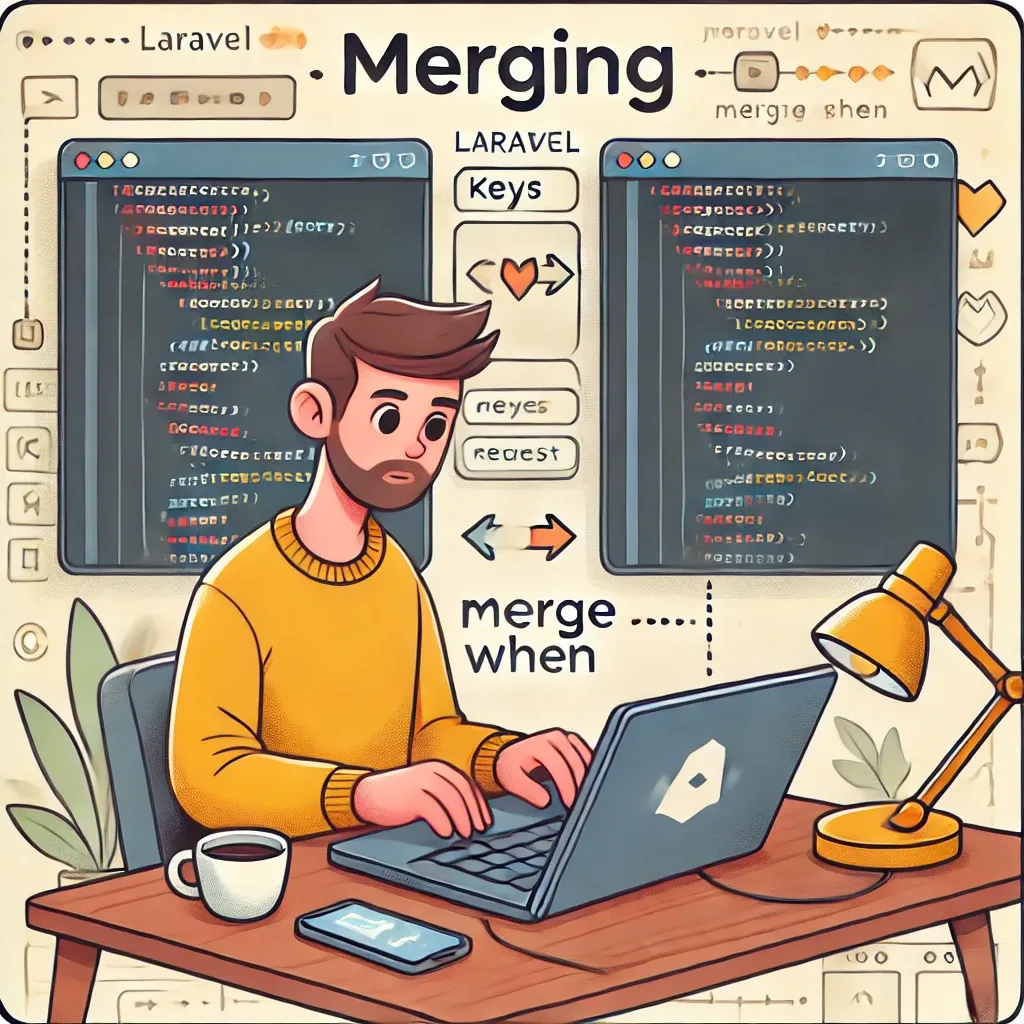
When building APIs with Laravel, you often need to include multiple attributes based on a single condition. Laravel's API Resources provide a powerful mergeWhen
method to achieve this efficiently. Let's explore how to use this feature to create cleaner, more conditional API responses.
Understanding mergeWhen
The mergeWhen
method allows you to include multiple attributes in your resource response based on a single condition. This is particularly useful when you have several related attributes that should be included or excluded together.
Basic Usage
Here's a simple example of how to use mergeWhen
:
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\JsonResource;
class UserResource extends JsonResource
{
public function toArray(Request $request): array
{
return [
'id' => $this->id,
'name' => $this->name,
'email' => $this->email,
$this->mergeWhen($request->user()->isAdmin(), [
'last_login' => $this->last_login,
'ip_address' => $this->ip_address,
'is_banned' => $this->is_banned,
]),
];
}
}
In this example, the additional user information is only included if the authenticated user is an admin.
Practical Applications
The mergeWhen
method is particularly useful in several scenarios:
• Role-based data exposure
• Feature-flag dependent attributes
• Conditional inclusion of computed properties
• Version-specific API responses
Advanced Example
Let's consider a more complex ProductResource
that uses mergeWhen
in various ways:
use Illuminate\Http\Request;
use Illuminate\Http\Resources\Json\JsonResource;
class ProductResource extends JsonResource
{
public function toArray(Request $request): array
{
return [
'id' => $this->id,
'name' => $this->name,
'price' => $this->price,
$this->mergeWhen($request->user()->isAdmin(), [
'cost' => $this->cost,
'profit_margin' => $this->profit_margin,
]),
$this->mergeWhen($request->include_stock_info, [
'stock_count' => $this->stock_count,
'reorder_level' => $this->reorder_level,
'is_in_stock' => $this->stock_count > 0,
]),
$this->mergeWhen($this->isOnSale(), [
'sale_price' => $this->sale_price,
'discount_percentage' => $this->discount_percentage,
'sale_ends_at' => $this->sale_ends_at,
]),
'category' => new CategoryResource($this->whenLoaded('category')),
];
}
}
By leveraging the mergeWhen
method in Laravel API Resources, you can create more flexible and efficient API responses. This approach allows you to conditionally include groups of related attributes, leading to cleaner, more maintainable code and more adaptable API endpoints.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!