Streamline Your Laravel Validation with Form Request Classes
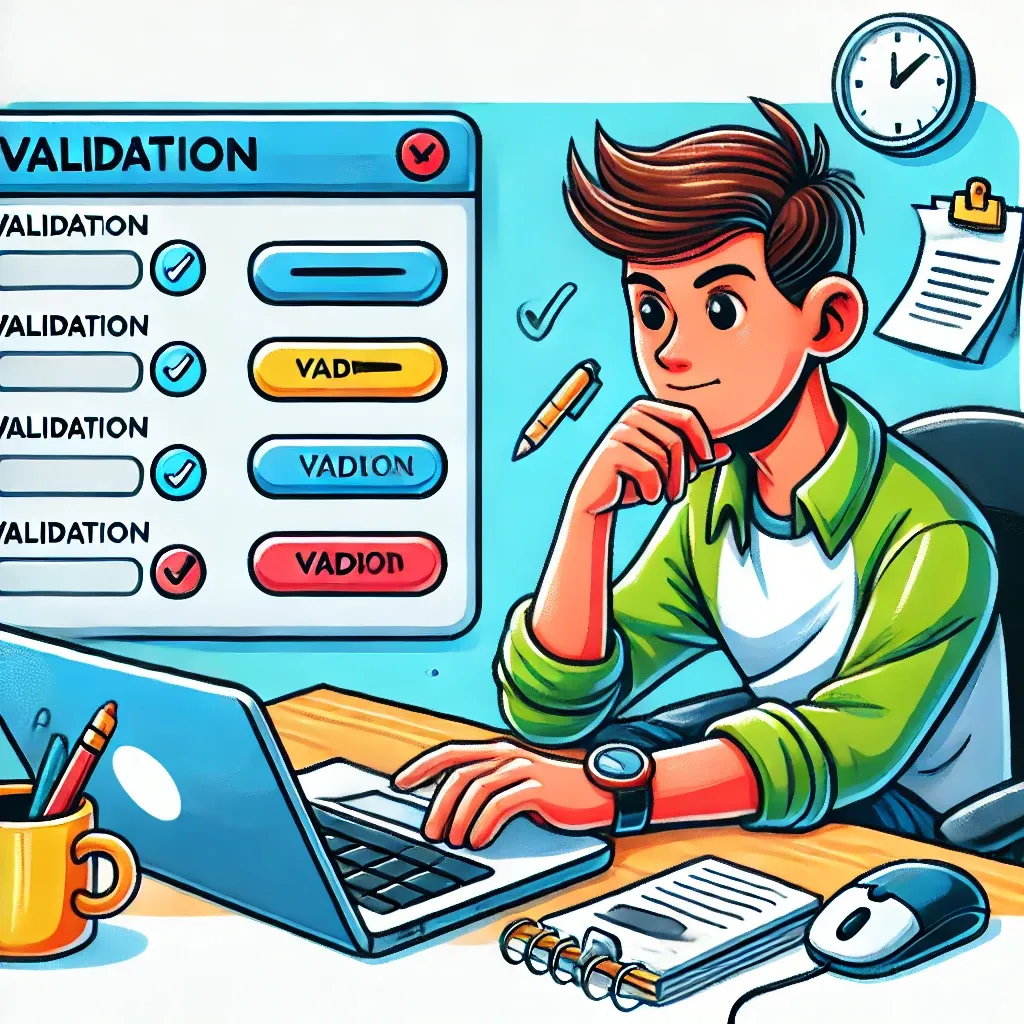
As Laravel developers, we're always looking for ways to keep our code clean, organized, and maintainable. One of the most powerful tools in our arsenal for achieving this is Form Request Validation. In this post, we'll explore how to leverage this feature to enhance your application's validation process.
What is Form Request Validation?
Form Request Validation is a Laravel feature that allows you to move your validation logic out of your controllers and into dedicated classes. This separation of concerns leads to cleaner, more maintainable code and promotes reusability across your application.
How to Use Form Request Validation
Let's walk through the process of implementing Form Request Validation:
- Create a Form Request Class:
// app/Http/Requests/StorePostRequest.php
use Illuminate\Foundation\Http\FormRequest;
class StorePostRequest extends FormRequest
{
public function authorize()
{
return true; // Or implement your authorization logic
}
public function rules()
{
return [
'title' => 'required|max:255',
'content' => 'required',
'publish_at' => 'nullable|date|after:today'
];
}
public function messages()
{
return [
'title.required' => 'A post title is required',
'content.required' => 'Post content cannot be empty',
'publish_at.after' => 'Publish date must be after today'
];
}
}
- Use the Form Request in Your Controller:
// app/Http/Controllers/PostController.php
use App\Http\Requests\StorePostRequest;
class PostController extends Controller
{
public function store(StorePostRequest $request)
{
// Validation will automatically occur
$validated = $request->validated();
Post::create($validated);
return redirect()->route('posts.index')->with('success', 'Post created successfully');
}
}
Benefits of Form Request Validation
- Clean Controllers: Your controller methods become more focused on their primary responsibility.
- Reusability: Validation rules can be reused across multiple controllers or actions.
- Improved Organization: Validation logic has a dedicated home, making it easier to manage and update.
- Advanced Validation: Form requests can handle complex validation scenarios, including conditional validation.
Advanced Usage: Custom Validation Rules
For more complex validations, you can create custom rules:
// app/Rules/Uppercase.php
use Illuminate\Contracts\Validation\Rule;
class Uppercase implements Rule
{
public function passes($attribute, $value)
{
return strtoupper($value) === $value;
}
public function message()
{
return 'The :attribute must be uppercase.';
}
}
// In your Form Request
use App\Rules\Uppercase;
public function rules()
{
return [
'title' => ['required', 'max:255', new Uppercase],
// other rules...
];
}
Pro Tips
- Use the
authorize
method in your form request to handle authorization logic. - Leverage the
prepareForValidation
method to modify data before validation occurs. - Use custom rules for complex validations that can't be expressed with built-in validation rules.
- Don't forget about the
sometimes
method for conditional validation.
Conclusion
Form Request Validation is a powerful feature that can significantly improve the structure and maintainability of your Laravel applications. By moving validation logic into dedicated classes, you create a more organized, reusable, and testable codebase.
Remember, clean code is not just about making things work—it's about making them work elegantly and efficiently. Form Request Validation is a step towards that goal, helping you build more robust and maintainable Laravel applications.
Happy coding!
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!