Streamline Your Laravel Models with Stringable Attributes
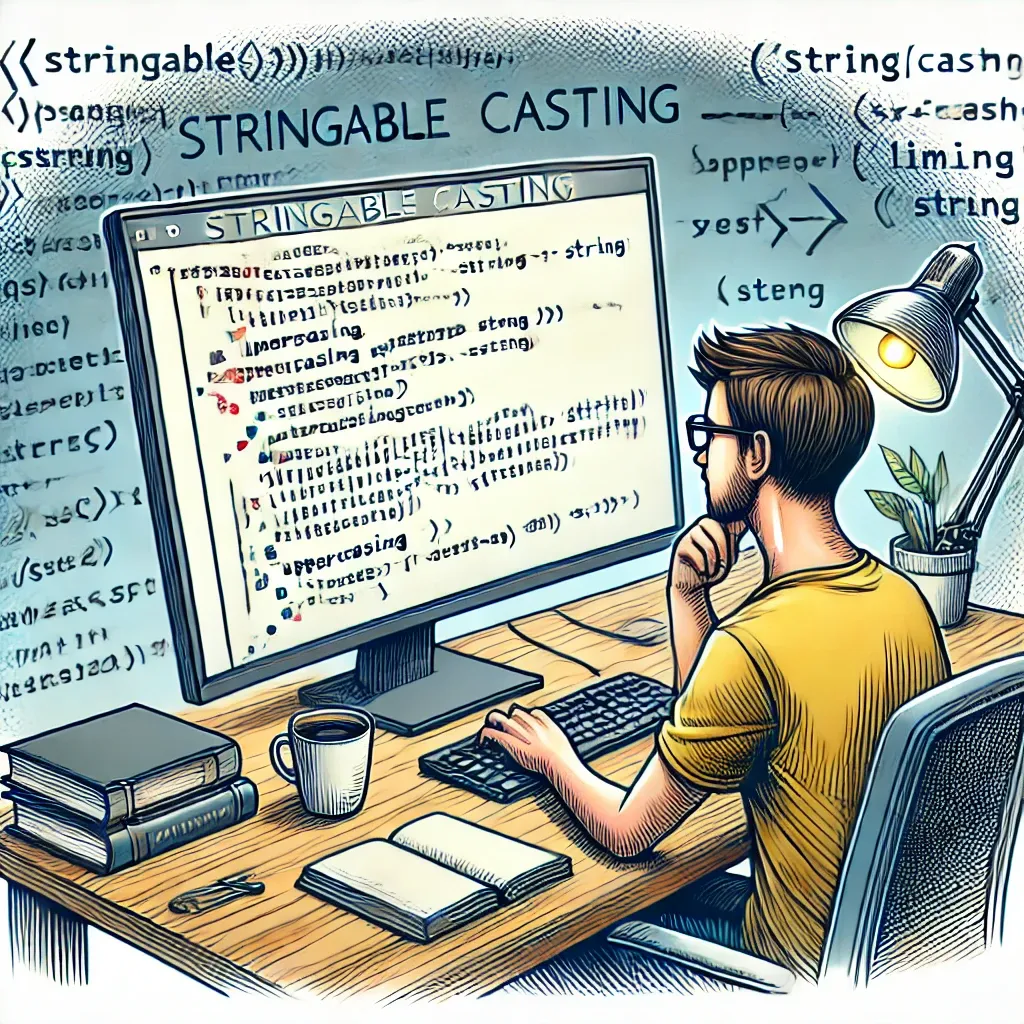
Laravel's AsStringable
cast is a powerful tool that can significantly enhance how you work with string attributes in your Eloquent models. By transforming your string attributes into Stringable objects, you gain access to a wide array of string manipulation methods, leading to cleaner and more expressive code.
Implementing AsStringable in Your Models
To use the AsStringable
cast, you simply need to specify it in your model's $casts
property:
use Illuminate\Database\Eloquent\Casts\AsStringable;
class Post extends Model
{
protected $casts = [
'title' => AsStringable::class,
'content' => AsStringable::class,
];
}
Real-World Example: Blog Post Management
Let's consider a blog application where we need to manipulate post titles and content for various purposes. Here's how we can leverage Stringable attributes:
class Post extends Model
{
protected $casts = [
'title' => AsStringable::class,
'content' => AsStringable::class,
];
public function getSnippetAttribute()
{
return $this->content->words(20)->append('...');
}
public function getSlugAttribute()
{
return $this->title->slug();
}
public function getFormattedContentAttribute()
{
return $this->content
->replaceMatches('/\*\*(.*?)\*\*/', '<strong>$1</strong>')
->replaceMatches('/\*(.*?)\*/', '<em>$1</em>')
->replaceMatches('/\n/', '<br>');
}
}
In this example:
• We cast both title
and content
as Stringable.
• getSnippetAttribute()
creates a 20-word preview of the post content.
• getSlugAttribute()
generates a URL-friendly slug from the post title.
• getFormattedContentAttribute()
applies some basic Markdown-like formatting to the content.
Usage in a controller:
class PostController extends Controller
{
public function show(Post $post)
{
return view('posts.show', [
'post' => $post,
'snippet' => $post->snippet,
'formattedContent' => $post->formatted_content,
]);
}
public function index()
{
$posts = Post::all()->map(function ($post) {
return [
'id' => $post->id,
'title' => $post->title->title(),
'snippet' => $post->snippet,
'slug' => $post->slug,
];
});
return view('posts.index', compact('posts'));
}
}
In your Blade templates:
<!-- posts/show.blade.php -->
<h1>{{ $post->title->title() }}</h1>
<p class="snippet">{{ $snippet }}</p>
<div class="content">{!! $formattedContent !!}</div>
<!-- posts/index.blade.php -->
@foreach ($posts as $post)
<article>
<h2><a href="{{ route('posts.show', $post['slug']) }}">{{ $post['title'] }}</a></h2>
<p>{{ $post['snippet'] }}</p>
</article>
@endforeach
This approach is particularly useful for content-heavy applications like blogs, wikis, or documentation systems. It allows you to encapsulate common string operations within your models, keeping your controllers and views clean and focused on their primary responsibilities.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!