Streamline HTTP Response Handling with Laravel's resource Method
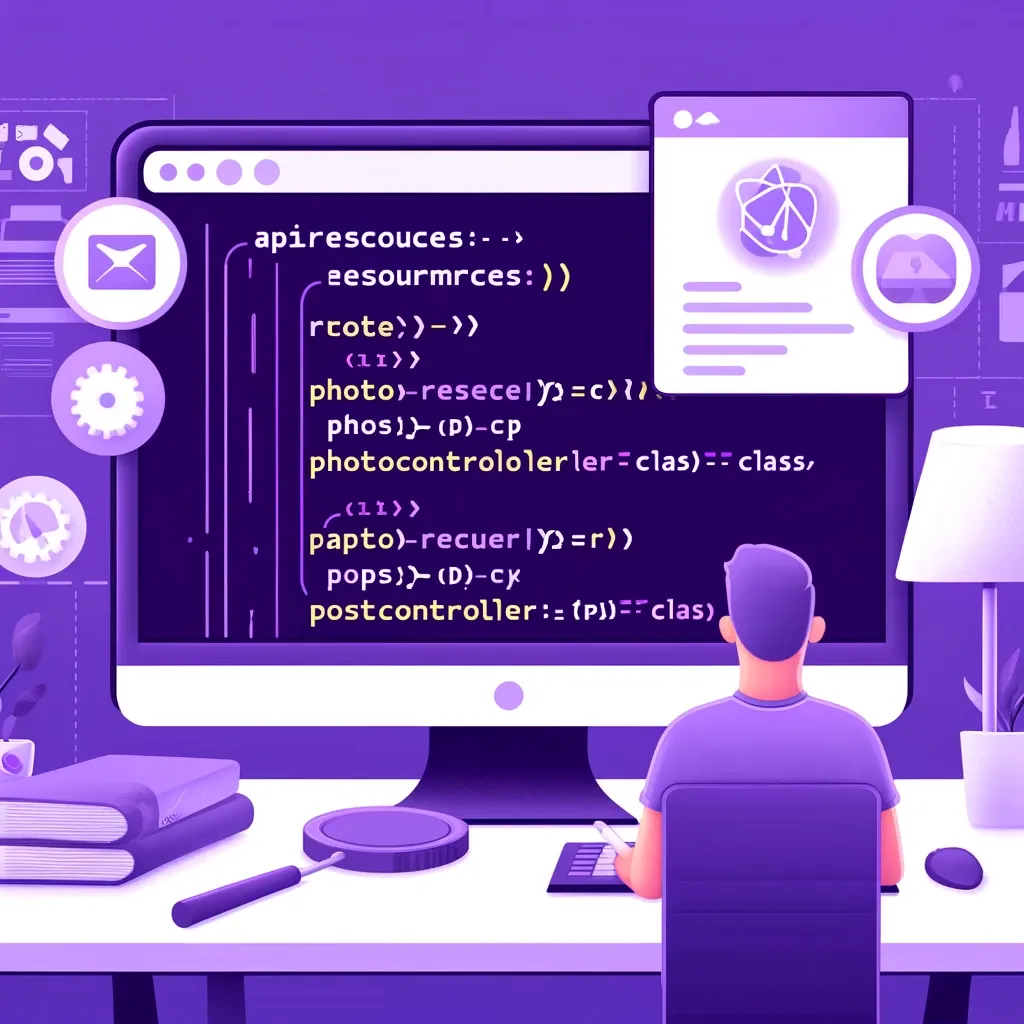
Ever wrestled with streams in Laravel's HTTP Client? The new resource()
method makes handling stream responses incredibly simple! Let's explore this handy improvement.
The Old vs New Way
The traditional approach was quite verbose:
// Old way
use GuzzleHttp\Psr7\StreamWrapper;
$response = Http::get($imageUrl);
Storage::disk('s3')->writeStream(
'thumbnail.png',
StreamWrapper::getResource($response->toPsrResponse()->getBody())
);
// New way
$response = Http::get($imageUrl);
Storage::disk('s3')->writeStream('thumbnail.png', $response->resource());
Real-World Example
Here's how you might use it in an image processing service:
class ImageService
{
public function downloadAndStore(string $imageUrl, string $filename)
{
try {
$response = Http::get($imageUrl);
if ($response->successful()) {
Storage::disk('s3')->writeStream(
"images/{$filename}",
$response->resource()
);
return true;
}
Log::error("Failed to download image: {$imageUrl}");
return false;
} catch (Exception $e) {
Log::error("Error processing image: {$e->getMessage()}");
throw $e;
}
}
}
The new resource()
method simplifies stream handling in your HTTP client responses, making file operations cleaner and more intuitive.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!