Streamline HTTP Client Configuration with Laravel's globalOptions Method
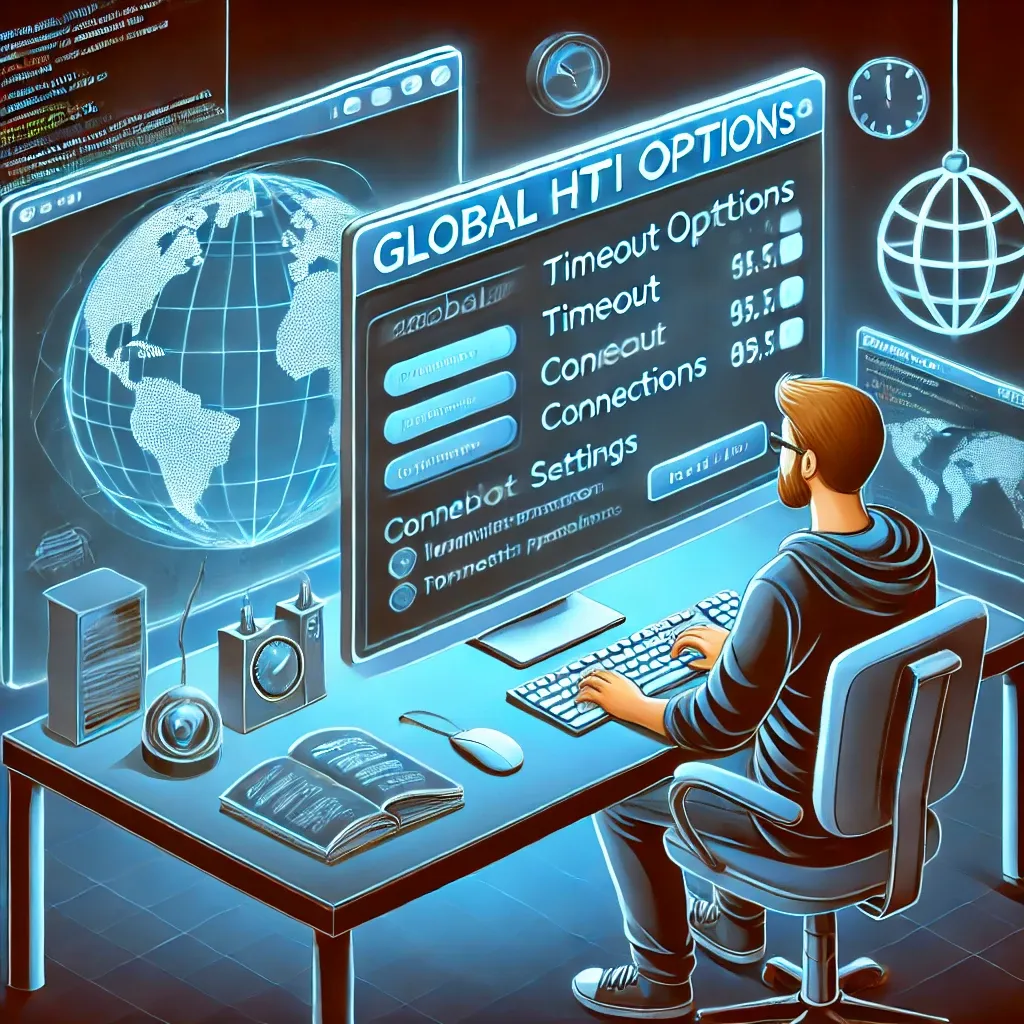
Managing HTTP client configuration across your entire Laravel application can be challenging. The new globalOptions method provides a centralized approach to setting default HTTP client behavior.
When your application interacts with multiple external APIs, configuring the HTTP client with consistent timeouts, headers, or other options often leads to repetitive code. Laravel's HTTP client now allows you to define these settings globally, ensuring consistent behavior while reducing duplication.
Let's see how the new globalOptions method works:
use Illuminate\Support\Facades\Http;
// Set global options in a service provider
Http::globalOptions([
'timeout' => 5,
'connect_timeout' => 2,
]);
Once configured, these options apply to all HTTP requests made through Laravel's HTTP client, unless explicitly overridden for specific requests.
Real-World Example
A common use case is setting up consistent timeouts, retry logic, or default headers across your application. Here's how you might configure this in a service provider:
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Http;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Bootstrap any application services.
*/
public function boot(): void
{
Http::globalOptions([
// Set reasonable timeouts
'timeout' => 10,
'connect_timeout' => 3,
// Add default headers
'headers' => [
'User-Agent' => 'MyApp/1.0',
'Accept' => 'application/json',
],
// Configure default retry behavior
'retry' => 3,
'retry_delay' => 100,
]);
}
}
These global settings provide a consistent foundation for all HTTP requests, but you can still override specific options when needed:
// Uses global options plus specific ones for this request
Http::withToken('api-token')
->post('https://api.example.com/endpoint', [
'data' => 'value'
]);
// Override timeout just for this request
Http::timeout(30)
->get('https://slow-api.example.com/endpoint');
It's worth noting that if you call globalOptions multiple times, subsequent calls will completely override the previous configuration, not merge with it. Plan your service provider setup accordingly to ensure all your global options are configured in a single call.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!