Streamline HTML Attribute Handling with Laravel's New AsHtmlString Cast
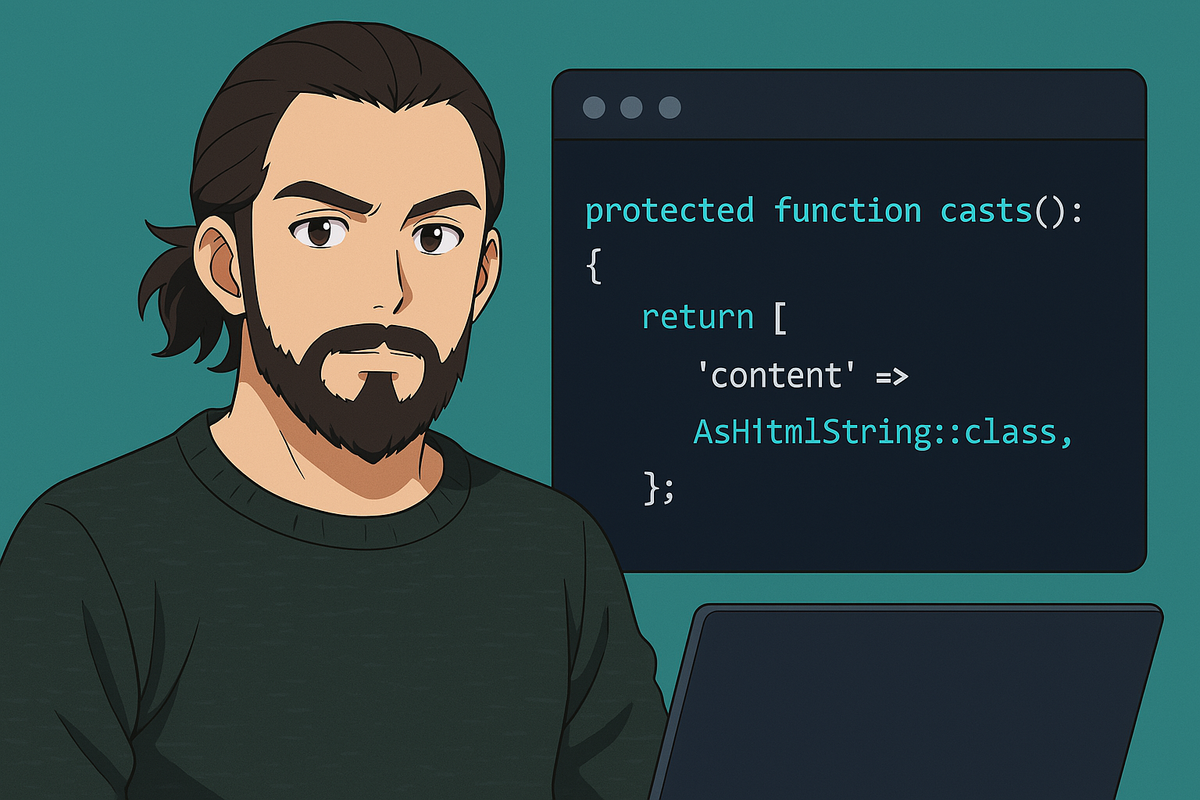
Working with HTML content stored in your database? Laravel v12.4 introduces the AsHtmlString cast, providing an elegant way to handle HTML-formatted attributes in your Eloquent models.
When dealing with rich content or formatted text in your database, you often need to display it without HTML escaping. Laravel has long had the HtmlString class for this purpose, but using it with Eloquent attributes required custom accessors or manual conversion. The new AsHtmlString cast simplifies this workflow by automatically converting string attributes to HtmlString instances.
Let's see how it works:
use Illuminate\Database\Eloquent\Casts\AsHtmlString;
use Illuminate\Database\Eloquent\Model;
class Article extends Model
{
protected function casts(): array
{
return [
'content' => AsHtmlString::class,
];
}
}
With this cast in place, whenever you access the content attribute, it will automatically be an HtmlString instance:
$article = Article::find(1);
$article->content; // Instance of \Illuminate\Support\HtmlString
Previous Approaches
Before this cast was available, you would typically handle HTML content in one of these ways:
// Using an accessor
protected function content(): Attribute
{
return Attribute::make(
get: fn (string $value) => str($value)->toHtmlString(),
);
}
// Or manually converting when needed
$htmlContent = new HtmlString($article->content);
$htmlContent = str($article->content)->toHtmlString();
Real-World Example
This cast is particularly useful for content management systems or applications that store formatted text:
class BlogPost extends Model
{
protected function casts(): array
{
return [
'title' => 'string',
'body' => AsHtmlString::class,
'excerpt' => AsHtmlString::class,
'meta_description' => 'string', // Regular string, will be escaped
];
}
}
In your Blade templates, you can now output the HTML content directly:
<article>
<h1>{{ $post->title }}</h1> <!-- Will be escaped -->
<div class="content">
{{ $post->body }} <!-- Will NOT be escaped -->
</div>
<div class="excerpt">
{{ $post->excerpt }} <!-- Will NOT be escaped -->
</div>
</article>
While this may seem like a narrow use case, it addresses a common need in many web applications, eliminating boilerplate code and making your models more expressive and type-safe.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!