Streaming Responses in Laravel: A Guide to Efficient Data Delivery
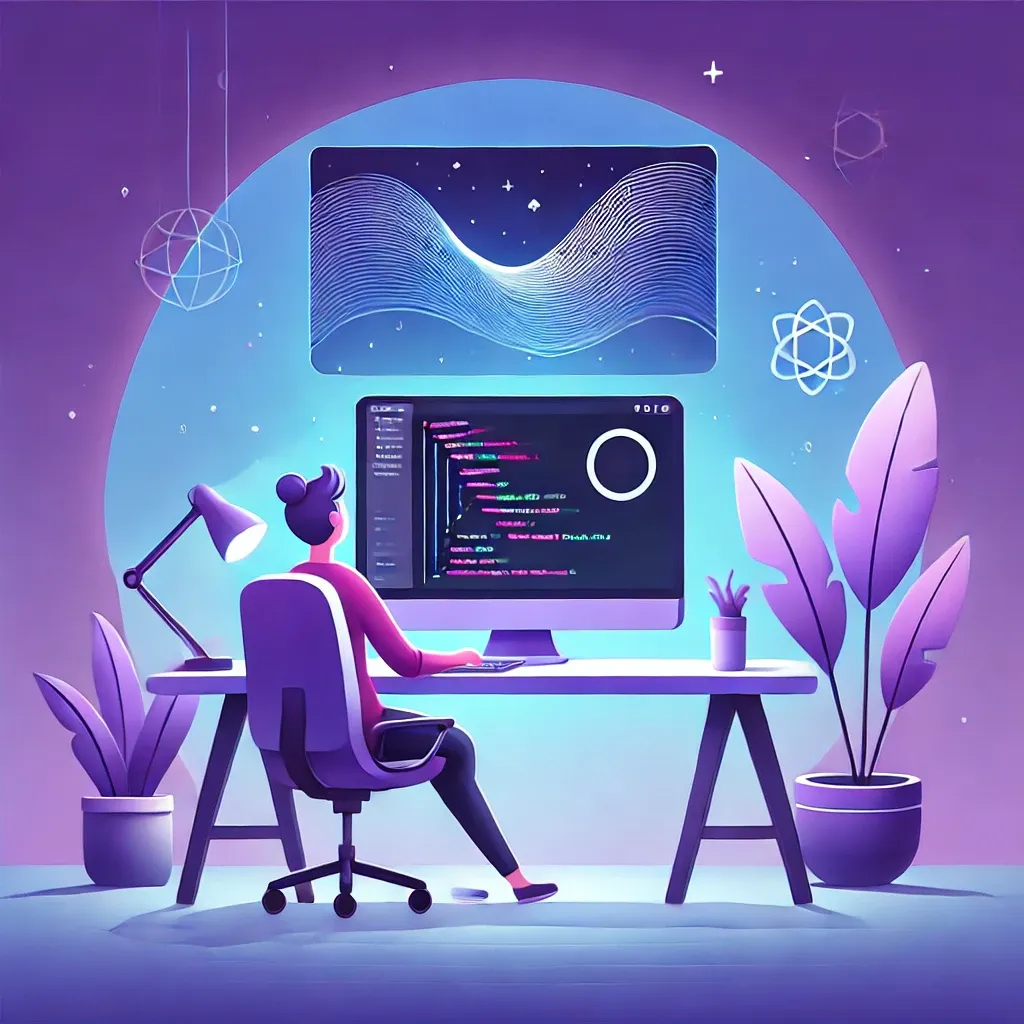
Need to handle large responses efficiently? Laravel's streaming response feature lets you send data chunks as they're generated! Let's explore this powerful performance optimization technique.
Basic Streaming
Here's the simplest way to create a streaming response:
return response()->stream(function () {
foreach (streamedContent() as $chunk) {
echo $chunk;
ob_flush();
flush();
}
}, 200, ['X-Accel-Buffering' => 'no']);
Real-World Example
Here's how you might use streaming for a large report generation:
class ReportController extends Controller
{
public function downloadLargeReport()
{
return response()->stream(function () {
// CSV Headers
echo "ID,Name,Email,Created At\n";
ob_flush();
flush();
// Process users in chunks
User::query()
->chunk(1000, function ($users) {
foreach ($users as $user) {
echo sprintf(
"%d,%s,%s,%s\n",
$user->id,
$user->name,
$user->email,
$user->created_at
);
ob_flush();
flush();
}
});
}, 200, [
'Content-Type' => 'text/csv',
'Content-Disposition' => 'attachment; filename="users.csv"',
'X-Accel-Buffering' => 'no'
]);
}
public function streamActivityLog()
{
return response()->stream(function () {
echo "<pre>\n";
ob_flush();
flush();
Activity::query()
->orderBy('id', 'desc')
->chunk(100, function ($activities) {
foreach ($activities as $activity) {
echo sprintf(
"[%s] %s: %s\n",
$activity->created_at->format('Y-m-d H:i:s'),
$activity->user->name,
$activity->description
);
ob_flush();
flush();
}
});
}, 200, [
'Content-Type' => 'text/plain',
'X-Accel-Buffering' => 'no'
]);
}
}
Streaming responses help reduce memory usage and improve user experience by sending data as it's generated rather than waiting for the entire response to be ready.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!