Streaming Large JSON Datasets in Laravel with streamJson()
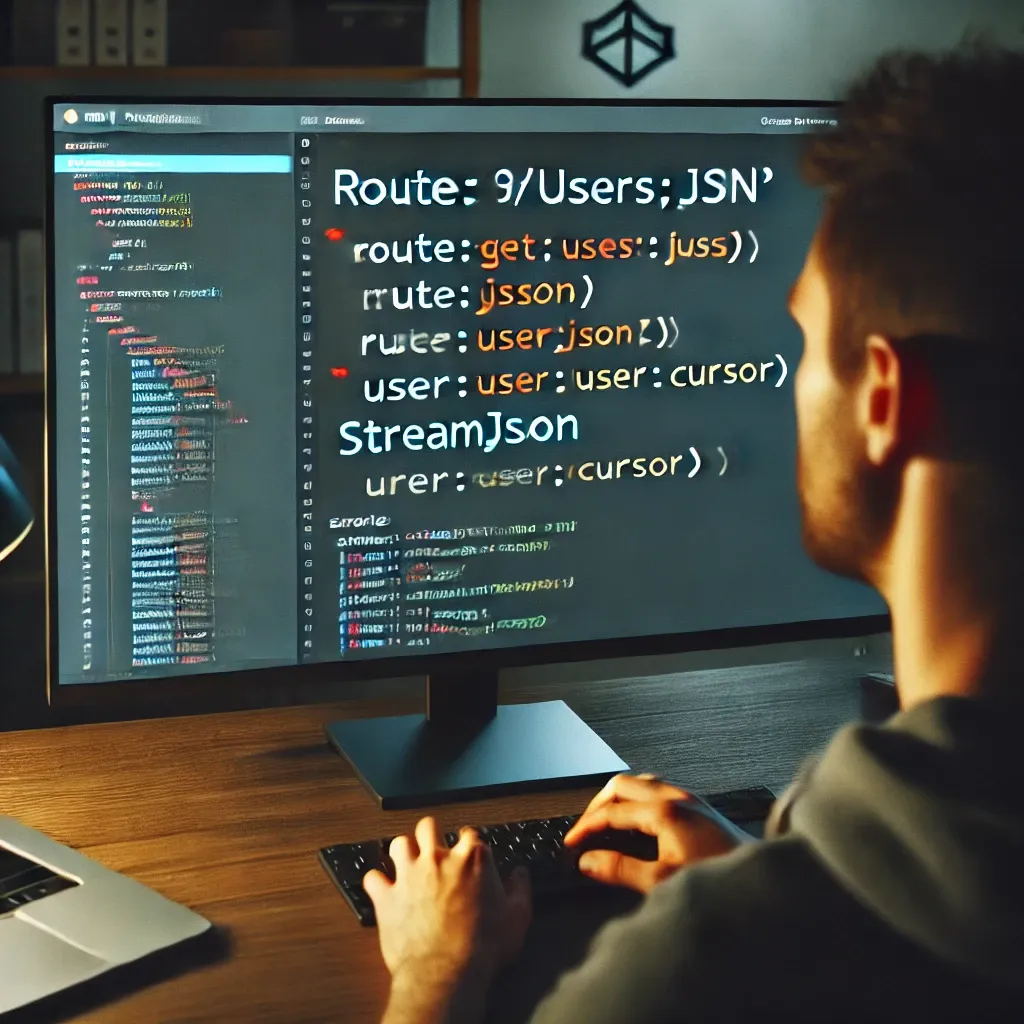
When dealing with large datasets in web applications, sending all the data at once can lead to long load times and high memory usage. Laravel provides a solution to this problem with the streamJson
method, which allows you to stream JSON data incrementally. This approach is particularly useful for large datasets that need to be sent progressively to the browser in a format that can be easily parsed by JavaScript.
Understanding streamJson()
The streamJson
method is available on the response object in Laravel. It allows you to stream JSON data incrementally, which can significantly improve performance and reduce memory usage for large datasets.
Basic Usage
Here's a simple example of how to use streamJson
:
use App\Models\User;
Route::get('/users.json', function () {
return response()->streamJson([
'users' => User::cursor(),
]);
});
In this example, we're using Eloquent's cursor()
method to efficiently iterate over the users table. The streamJson
method will then stream this data as JSON to the client.
Real-Life Example
Let's consider a scenario where we need to stream a large dataset of products, including their categories and tags. Here's how we might implement this:
<?php
namespace App\Http\Controllers;
use App\Models\Product;
use Illuminate\Http\Request;
class ProductController extends Controller
{
public function index()
{
return response()->streamJson([
'products' => Product::with('category', 'tags')->cursor()->map(function ($product) {
return [
'id' => $product->id,
'name' => $product->name,
'price' => $product->price,
'category' => $product->category->name,
'tags' => $product->tags->pluck('name'),
];
}),
]);
}
}
In this example, we're eagerly loading the category
and tags
relationships to avoid N+1 query issues. We're then using cursor()
to efficiently iterate over the products, and map()
to format each product as we stream it.
Here's what the output might start to look like:
{
"products": [
{
"id": 1,
"name": "Product 1",
"price": 19.99,
"category": "Electronics",
"tags": ["new", "featured"]
},
{
"id": 2,
"name": "Product 2",
"price": 29.99,
"category": "Clothing",
"tags": ["sale"]
},
// ... more products will be streamed as they're processed
]
}
The browser will receive this data incrementally, allowing it to start processing and displaying results before the entire dataset has been transferred.
By using streamJson
, you can handle large datasets more efficiently, providing a better user experience with faster initial load times and progressive updates to the UI. This approach is particularly valuable when dealing with datasets that are too large to comfortably load all at once.