Spice Up Your Laravel Queries with inRandomOrder
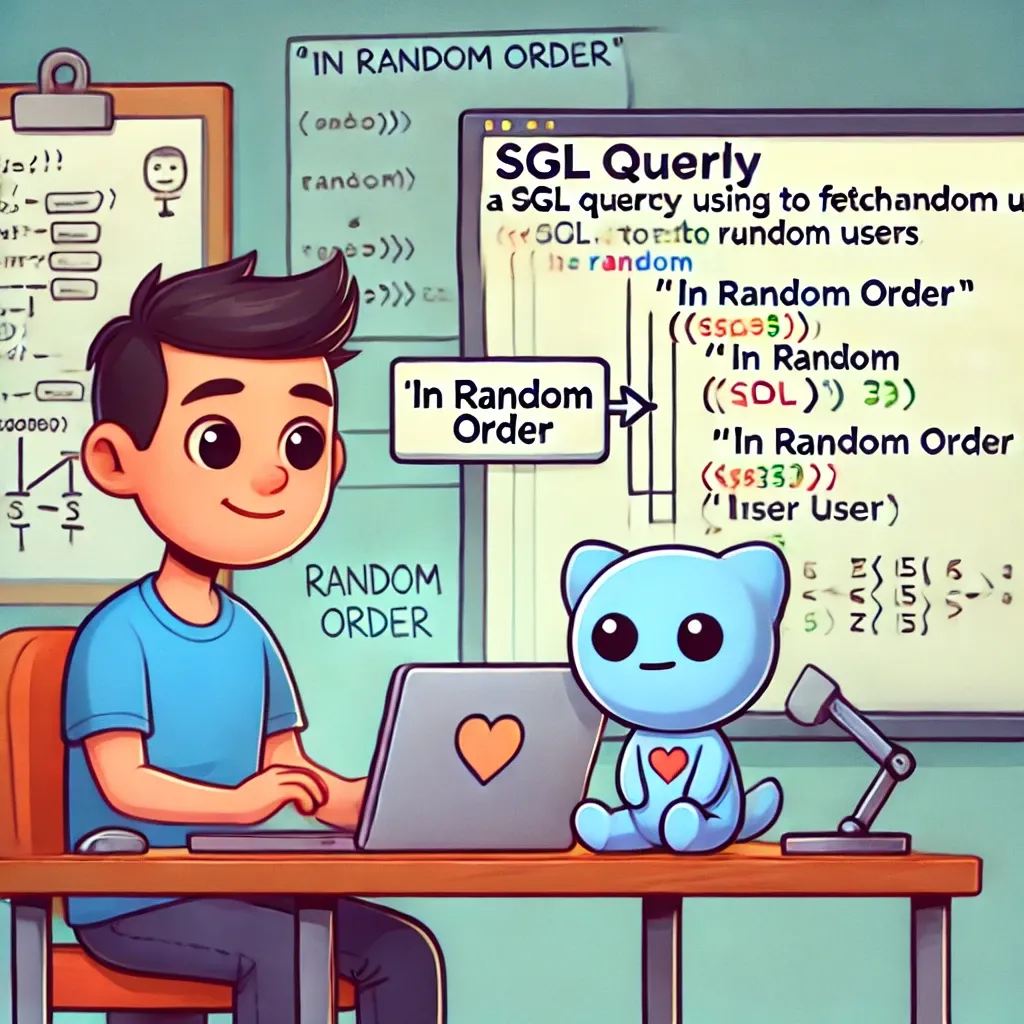
Ever needed to add a dash of randomness to your database queries? Laravel's inRandomOrder
method is here to help! Let's explore how this nifty tool can bring some unpredictability to your data retrieval.
What's inRandomOrder All About?
The inRandomOrder
method in Laravel lets you grab random records from your database. It's like shuffling a deck of cards before picking one.
How to Use It
Here's a quick example:
use Illuminate\Support\Facades\DB;
$randomUser = DB::table('users')
->inRandomOrder()
->first();
This query picks a random user from the 'users' table. Simple, right?
Behind the Scenes
When you use inRandomOrder
, Laravel adds an ORDER BY RAND()
(or something similar, depending on your database) to your query. It's like telling your database to mix things up before serving the results.
Real-World Example: Quote of the Day
Imagine you're building an app that shows a new inspirational quote each day. Here's how you might do it:
use App\Models\Quote;
use Illuminate\Support\Facades\Cache;
class QuoteController extends Controller
{
public function getDailyQuote()
{
return Cache::remember('daily_quote', now()->endOfDay(), function () {
return Quote::inRandomOrder()->first();
});
}
}
What's happening here:
- We're using the
Quote
model to talk to our quotes table. inRandomOrder()
picks a random quote.- We're using caching to keep the same quote all day long.
- The cache resets at midnight, so we get a fresh quote each day.
This way, your app serves up a new dose of inspiration every day, without hammering your database.
Using inRandomOrder
in Laravel is a great way to add some surprise to your app. Whether it's picking random content or making fair selections, this method has got you covered.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!