Sorting Collection Keys in Laravel Using sortKeysUsing
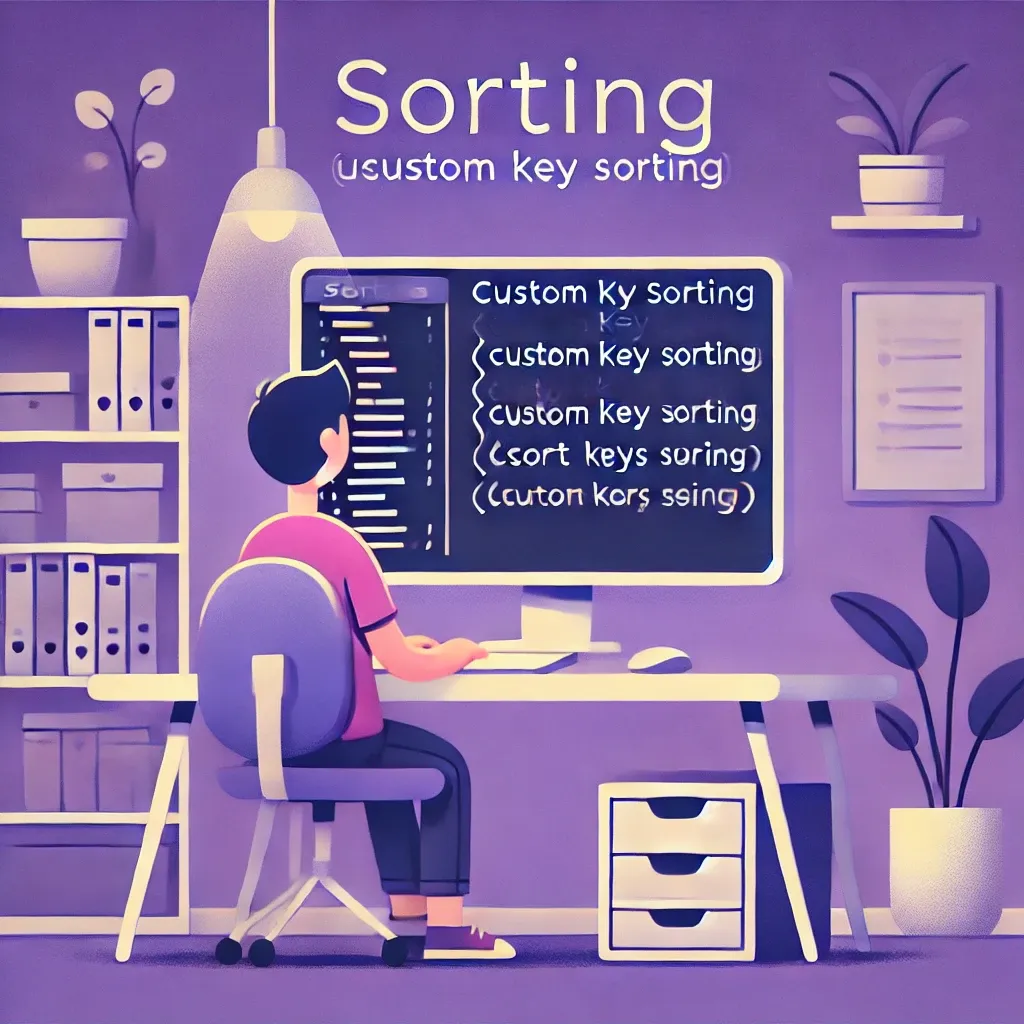
This powerful feature lets you customize key sorting with any comparison function, perfect for natural sorting and custom ordering logic.
Basic Usage
Here's how to sort collection keys using a callback:
$collection = collect([
'ID' => 22345,
'first' => 'John',
'last' => 'Doe',
]);
$sorted = $collection->sortKeysUsing('strnatcasecmp');
Real-World Example
Here's a practical implementation for sorting configuration settings:
class ConfigurationManager
{
public function getOrderedSettings(array $settings)
{
return collect($settings)
->sortKeysUsing(function ($a, $b) {
// Sort priority prefixes first
$isPriorityA = str_starts_with($a, 'priority_');
$isPriorityB = str_starts_with($b, 'priority_');
if ($isPriorityA && !$isPriorityB) return -1;
if (!$isPriorityA && $isPriorityB) return 1;
// Then sort by category
$categoryA = explode('_', $a)[0];
$categoryB = explode('_', $b)[0];
if ($categoryA !== $categoryB) {
return $categoryA <=> $categoryB;
}
// Finally, natural case-insensitive sort
return strnatcasecmp($a, $b);
});
}
}
// Usage
$settings = [
'email_host' => 'smtp.example.com',
'priority_notifications' => true,
'app_name' => 'My App',
'priority_maintenance' => false,
'email_port' => 587,
];
$manager = new ConfigurationManager();
$ordered = $manager->getOrderedSettings($settings);
The sortKeysUsing
method is perfect for situations where you need precise control over how collection keys are ordered, especially when dealing with configuration settings, form fields, or any associative data.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!