Smart Date Navigation with Carbon in Laravel
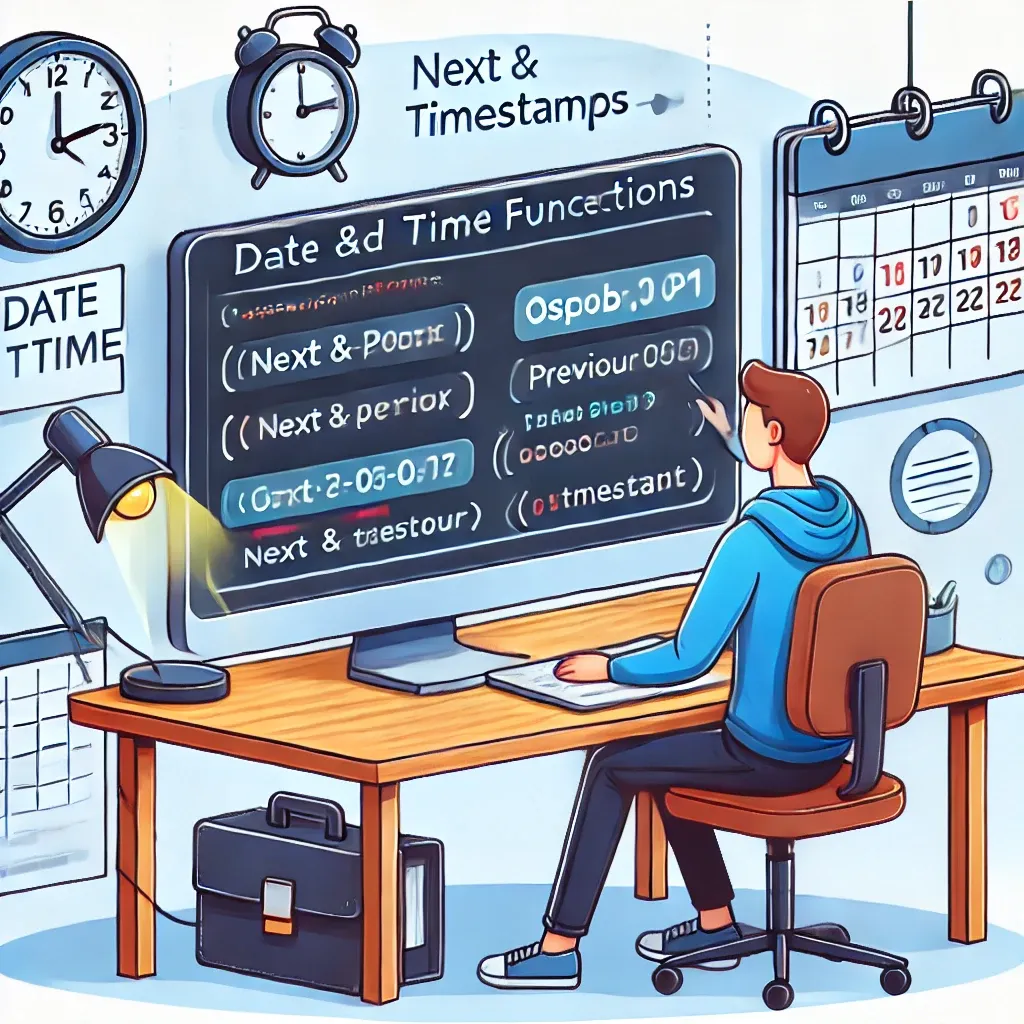
Laravel's Carbon library enhances date manipulation with intuitive navigation methods, making it easy to find specific times and days in your code.
Whether you need to find the next Monday, the previous Friday, or specific times like afternoon meetings, Carbon provides a fluent interface for navigating dates. These methods make complex date calculations simple and readable.
Let's see how it works:
$now = now(); // 2024-02-15 14:30:00
$now->next('15:30'); // 2024-02-16 15:30:00
$now->next('Monday'); // 2024-02-19 00:00:00
$now->previous('Friday'); // 2024-02-09 00:00:00
Real-World Example
Here's how you might use these methods in a scheduling system:
class AppointmentScheduler
{
public function findNextAvailable(Carbon $from)
{
// Skip to next weekday if weekend
if ($from->isWeekend()) {
$from = $from->nextWeekday();
}
// Set to next business hours
return $from->next('15:30');
}
public function scheduleWeeklyMeeting(Carbon $start)
{
return [
'this_week' => $start->next('Tuesday')->setTime(10, 30),
'next_week' => $start->next('Tuesday')->addWeek()->setTime(10, 30),
'backup_day' => $start->next('Thursday')->setTime(14, 30)
];
}
public function getWeekendAvailability(Carbon $date)
{
return [
'next_weekend' => [
'start' => $date->nextWeekendDay()->setTime(9, 30),
'end' => $date->nextWeekendDay()->setTime(17, 30)
],
'following_weekend' => [
'start' => $date->addWeek()->nextWeekendDay()->setTime(9, 30),
'end' => $date->addWeek()->nextWeekendDay()->setTime(17, 30)
]
];
}
}
These navigation methods simplify complex date calculations, making your code more readable and maintainable. Think of them as a GPS for dates - tell them where you want to go, and they'll find the right path there.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!