Simplifying View Logic with Laravel Blade's Service Injection
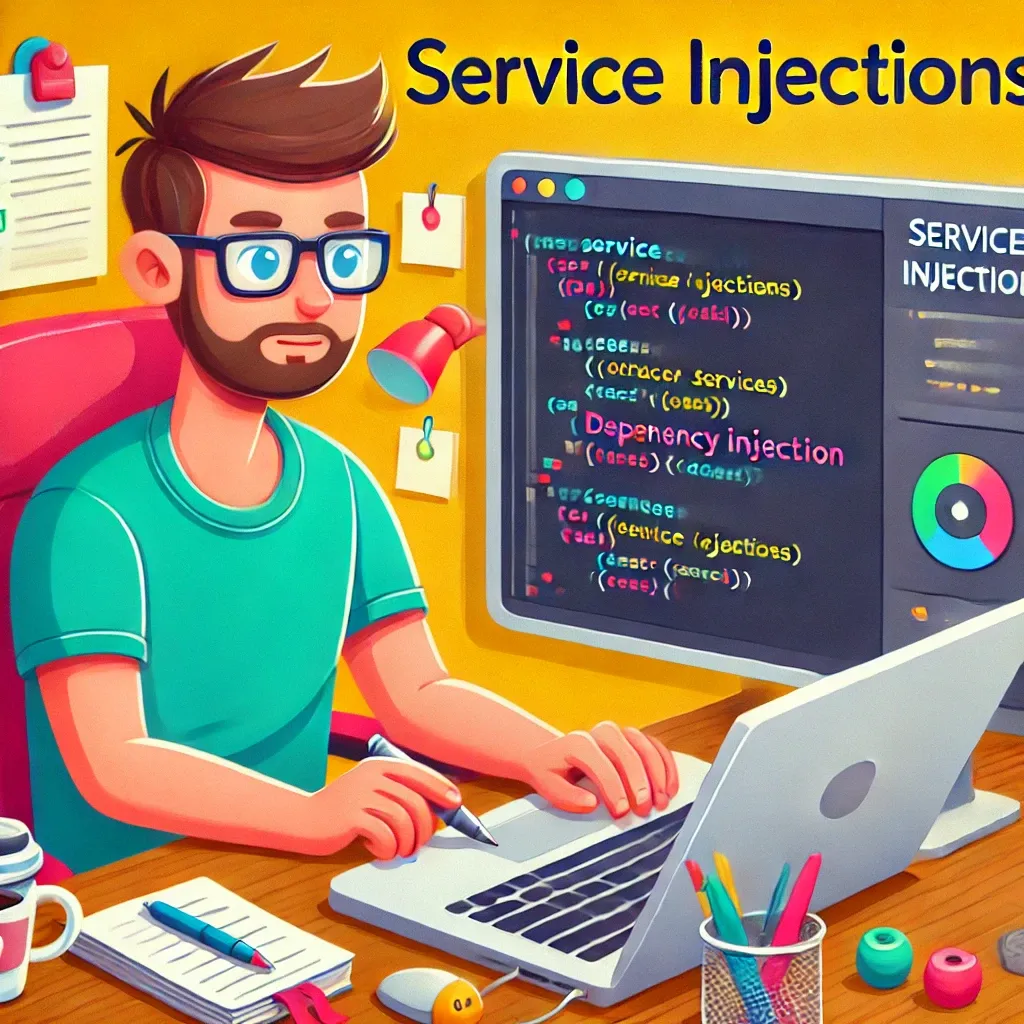
Laravel's Blade templating engine is known for its simplicity and power. One of its lesser-known but incredibly useful features is service injection. This feature allows you to directly inject services into your Blade views, reducing the need to pass data through controllers. Let's explore how you can leverage this feature to write cleaner, more maintainable code.
Understanding Blade Service Injection
Blade service injection allows you to use the @inject
directive to retrieve a service from the Laravel service container directly in your view. This can be particularly useful for accessing utility classes or services that provide view-specific functionality.
Basic Usage
The basic syntax for service injection in Blade is as follows:
@inject('metrics', 'App\Services\MetricsService')
<div>
Monthly Revenue: {{ $metrics->monthlyRevenue() }}
</div>
In this example:
'metrics'
is the variable name you'll use in the view to access the service.'App\Services\MetricsService'
is the class or interface name of the service you want to inject.
Real-World Example: Dashboard with Metrics
Let's consider a more comprehensive example of a dashboard that displays various metrics:
// app/Services/DashboardMetricsService.php
namespace App\Services;
class DashboardMetricsService
{
public function totalUsers()
{
return \App\Models\User::count();
}
public function monthlyRevenue()
{
return \App\Models\Order::whereMonth('created_at', now()->month)->sum('total');
}
public function averageOrderValue()
{
return \App\Models\Order::avg('total');
}
}
// resources/views/dashboard.blade.php
@inject('metrics', 'App\Services\DashboardMetricsService')
<div class="dashboard">
<div class="metric">
<h3>Total Users</h3>
<p>{{ number_format($metrics->totalUsers()) }}</p>
</div>
<div class="metric">
<h3>Monthly Revenue</h3>
<p>${{ number_format($metrics->monthlyRevenue(), 2) }}</p>
</div>
<div class="metric">
<h3>Average Order Value</h3>
<p>${{ number_format($metrics->averageOrderValue(), 2) }}</p>
</div>
</div>
In this example, we've injected a DashboardMetricsService
into our view, allowing us to easily display various metrics without cluttering our controller with this logic.
Combining with View Composers
For more complex scenarios, you can combine service injection with view composers:
// app/Providers/AppServiceProvider.php
use Illuminate\Support\Facades\View;
use App\Services\DashboardMetricsService;
public function boot()
{
View::composer('dashboard', function ($view) {
$view->with('metrics', new DashboardMetricsService());
});
}
// resources/views/dashboard.blade.php
<div class="dashboard">
<div class="metric">
<h3>Total Users</h3>
<p>{{ number_format($metrics->totalUsers()) }}</p>
</div>
<!-- ... other metrics ... -->
</div>
This approach allows you to inject the service once for the entire view, which can be more efficient than using @inject
multiple times.
Blade service injection is a powerful feature that can help you write cleaner, more maintainable views in Laravel. By allowing you to inject services directly into your templates, it provides a way to keep your controllers lean while still leveraging complex business logic in your views. When used judiciously, it can significantly improve the organization and readability of your Laravel applications.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!