Simplifying Route Parameters with Laravel's URL Defaults
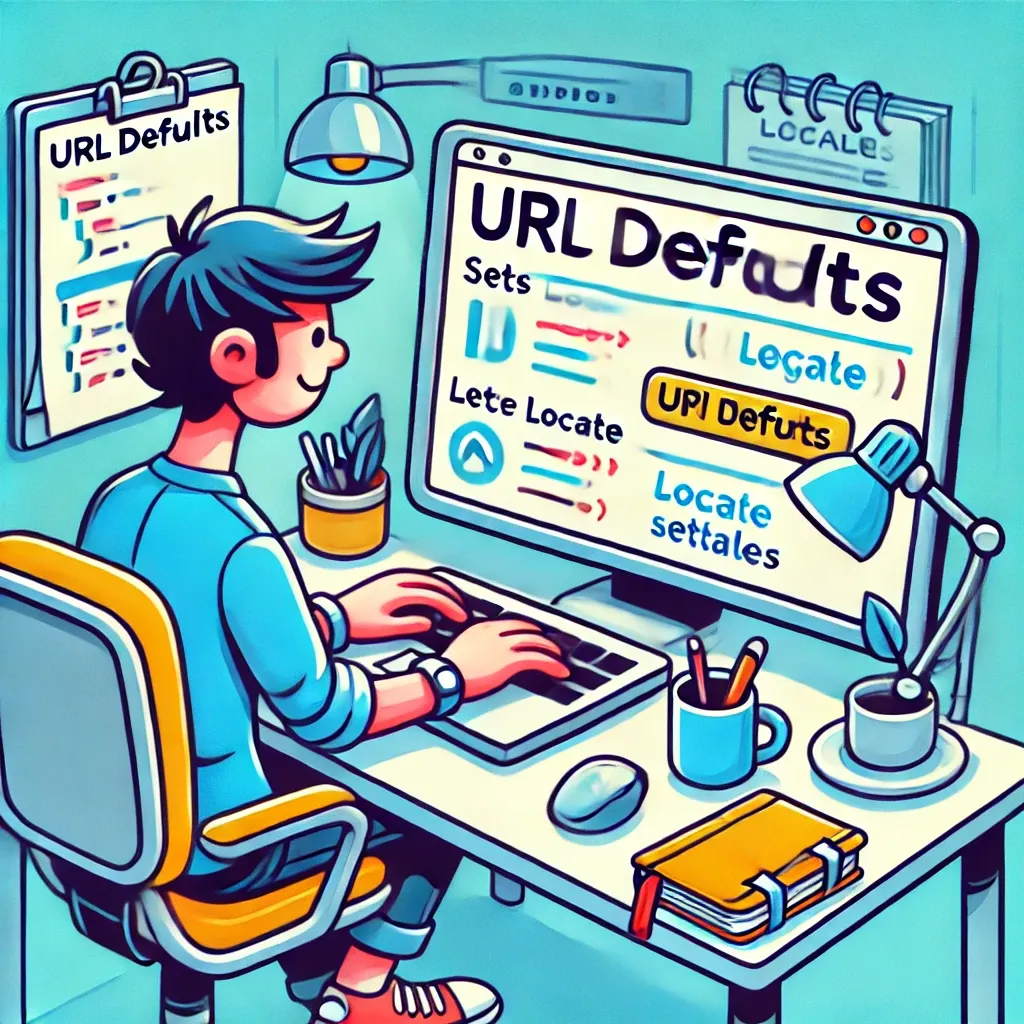
When building Laravel applications, especially those supporting multiple languages or complex routing structures, you often find yourself dealing with repetitive URL parameters. Laravel's URL defaults feature offers an elegant solution to this problem, allowing you to set default values for URL parameters across your entire application. Let's explore how to leverage this powerful feature in your Laravel projects.
Understanding URL Defaults
URL defaults in Laravel allow you to specify request-wide default values for certain URL parameters. This is particularly useful for parameters that are common across many routes, such as locale or currency.
Setting Up URL Defaults
The best place to set up URL defaults is in a middleware. This ensures that the defaults are applied early in the request lifecycle. Here's an example of how to create and implement such a middleware:
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\URL;
use Symfony\Component\HttpFoundation\Response;
class SetDefaultLocaleForUrls
{
public function handle(Request $request, Closure $next): Response
{
URL::defaults(['locale' => $request->user()->locale ?? config('app.locale')]);
return $next($request);
}
}
In this middleware, we're setting a default 'locale' parameter based on the authenticated user's preference or falling back to the application's default locale.
Registering the Middleware
To use this middleware, you need to register it in your app/Http/Kernel.php
file:
protected $middleware = [
// Other middleware...
\App\Http\Middleware\SetDefaultLocaleForUrls::class,
];
Using URL Defaults in Routes
Once you've set up URL defaults, you can define routes that use these default values:
Route::get('{locale}/posts', function () {
// ...
})->name('posts.index');
Now, when generating URLs for this route, you don't need to specify the locale unless you want to override the default:
// Uses the default locale
$url = route('posts.index');
// Overrides the default locale
$url = route('posts.index', ['locale' => 'fr']);
Real-World Example: Multi-language Blog
Let's consider a more comprehensive example of a multi-language blog:
// In App\Http\Middleware\SetDefaultLocaleForUrls
public function handle(Request $request, Closure $next): Response
{
$locale = $request->session()->get('locale', config('app.locale'));
URL::defaults(['locale' => $locale]);
app()->setLocale($locale);
return $next($request);
}
// In routes/web.php
Route::prefix('{locale}')->group(function () {
Route::get('/', [HomeController::class, 'index'])->name('home');
Route::get('posts', [PostController::class, 'index'])->name('posts.index');
Route::get('posts/{post}', [PostController::class, 'show'])->name('posts.show');
});
// In a view file
<a href="{{ route('posts.index') }}">{{ __('All Posts') }}</a>
<a href="{{ route('posts.show', $post) }}">{{ $post->title }}</a>
// In a controller
public function changeLocale(Request $request, $locale)
{
$request->session()->put('locale', $locale);
return redirect()->back();
}
In this example:
- The middleware sets the default locale based on the user's session.
- All routes are prefixed with the locale parameter.
- In views, we don't need to specify the locale when generating URLs.
- A
changeLocale
method allows users to switch languages, updating the session and thus the URL defaults.
Laravel's URL defaults feature provides a powerful way to simplify route handling in complex applications, especially those dealing with internationalization or other global parameters. By leveraging this feature, you can create more maintainable, DRY code while providing a better experience for your users and fellow developers alike.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!