Simplifying Data Transformation with Laravel's transform() Helper
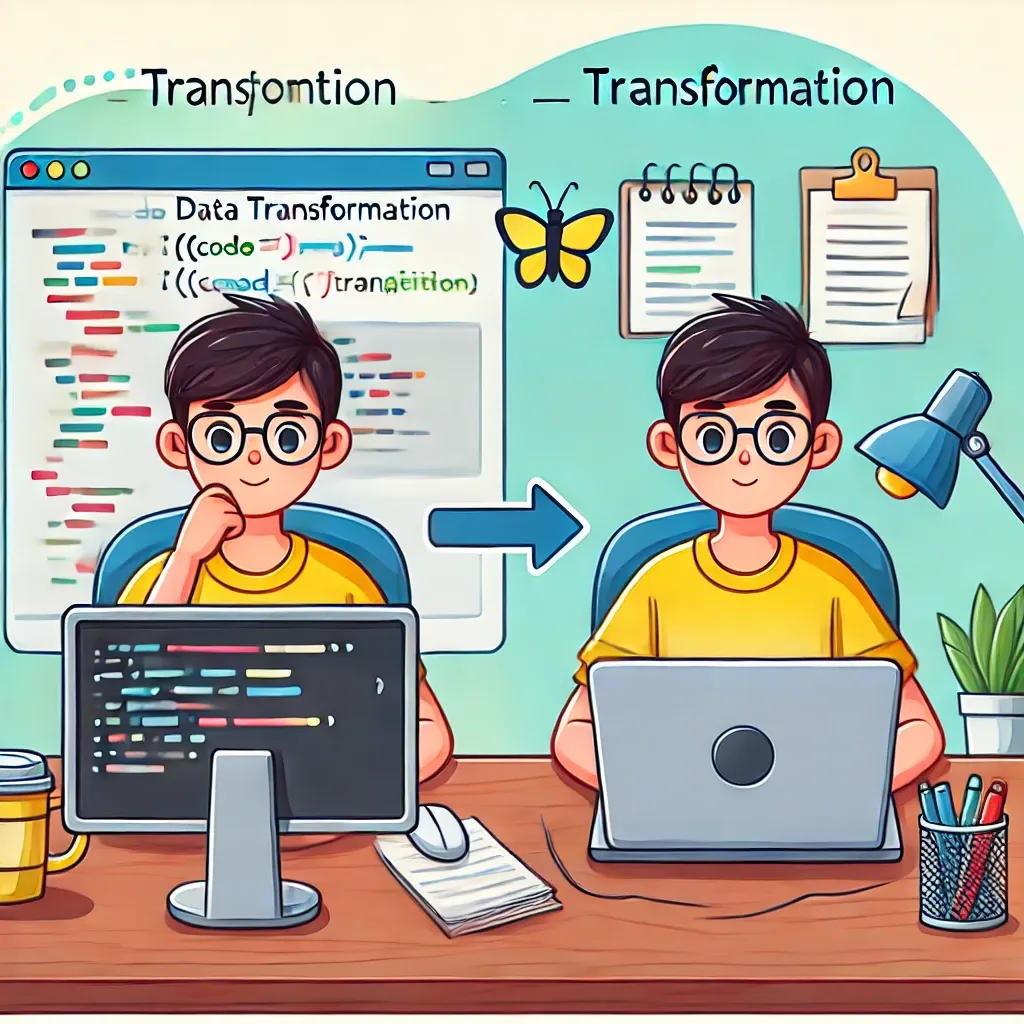
Laravel's transform()
helper is a powerful yet often overlooked tool that can significantly clean up your code when dealing with conditional data modifications. This versatile function allows you to transform data only when it meets certain conditions, making it perfect for handling user input, formatting API responses, or providing default values. Let's explore how to effectively use the transform()
helper in your Laravel projects.
Basic Usage
The transform()
helper takes up to three arguments:
- The value to transform
- A callback function to apply if the value is not null
- An optional default value to return if the original value is null
Here's a simple example:
$upper = transform('hello', fn ($value) => strtoupper($value));
// Output: HELLO
In this case, 'hello' is transformed to uppercase because it's not null.
Handling Null Values
One of the most powerful features of transform()
is its ability to handle null values gracefully:
$result = transform(null, fn ($value) => $value * 2, 'No value');
// Output: 'No value'
Here, since the input is null, the callback is not executed, and the default value 'No value' is returned instead.
Real-World Examples
User Name Formatting
Let's say you want to display a user's name, but if it's not set, you want to show 'Guest' instead:
$formatted = transform($user->name, fn ($name) => ucfirst($name), 'Guest');
// Output: 'John' (if $user->name is 'john') or 'Guest' (if $user->name is null)
API Response Formatting
When building APIs, you often need to format data before sending it back to the client:
$response = [
'user' => transform($user, function ($user) {
return [
'id' => $user->id,
'name' => $user->name,
'email' => $user->email,
'joined_at' => $user->created_at->format('Y-m-d'),
];
}, null),
];
This will return formatted user data if a user exists, or null if there's no user.
Default Values in Configurations
The transform()
helper can be useful when working with configuration values that might not be set:
$timeout = transform(config('app.timeout'), fn ($timeout) => $timeout * 60, 30);
This multiplies the configured timeout by 60 (converting minutes to seconds) if it's set, or defaults to 30 seconds if it's not.
Chaining Transformations
You can chain transform()
calls for more complex transformations:
$result = transform(
transform($input, fn ($value) => trim($value)),
fn ($value) => strtolower($value),
'default'
);
This trims the input, converts it to lowercase, and provides a default value if the input was null or an empty string.
Comparison with Ternary Operator
While you could use the ternary operator for similar operations, transform()
often leads to cleaner, more readable code:
// Using ternary operator
$name = $user->name ? ucfirst($user->name) : 'Guest';
// Using transform()
$name = transform($user->name, fn ($name) => ucfirst($name), 'Guest');
The transform()
version is more expressive and easier to extend if more complex logic is needed.
Performance Considerations
The transform()
helper is implemented efficiently in Laravel, but for extremely performance-critical sections of your code, you might want to benchmark it against direct conditionals to ensure it meets your needs.
Laravel's transform()
helper is a versatile tool that can significantly improve the readability and maintainability of your code. By providing a clean way to handle conditional data transformations and null values, it helps you write more expressive and error-resistant code. Whether you're cleaning up user input, formatting API responses, or providing default values, the transform()
helper is a valuable addition to your Laravel toolkit.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!