Simplifying API Interactions with Laravel's HTTP Client
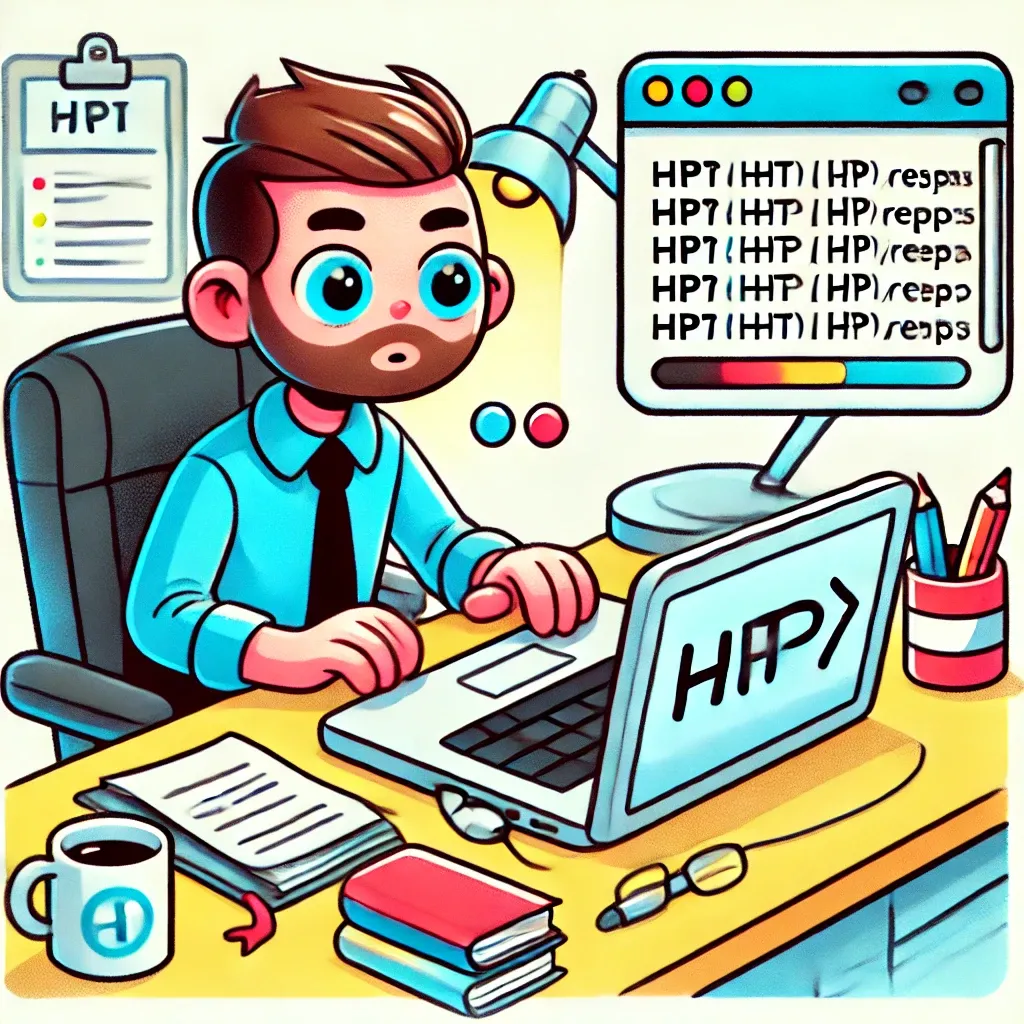
Laravel's HTTP client provides a clean, expressive interface for making HTTP requests. Whether you're consuming external APIs or testing your own, this powerful tool can significantly streamline your code. Let's dive into how to leverage Laravel's HTTP client effectively.
Basic Usage
Making a GET request is straightforward:
use Illuminate\Support\Facades\Http;
$response = Http::get('https://api.github.com/users/laravel');
$statusCode = $response->status();
$body = $response->body();
$jsonData = $response->json();
For POST requests with JSON data:
$response = Http::post('https://api.example.com/users', [
'name' => 'Steve',
'role' => 'Developer',
]);
Request Headers
You can easily add headers to your requests:
$response = Http::withHeaders([
'X-API-Key' => 'your-api-key',
'Accept' => 'application/json',
])->get('https://api.example.com/data');
Handling Authentication
For APIs requiring authentication:
$response = Http::withBasicAuth('username', 'password')
->get('https://api.example.com/users');
// Or for bearer token authentication
$response = Http::withToken('your-token')
->get('https://api.example.com/users');
Timeout and Retries
Set timeouts and automatic retries:
$response = Http::timeout(3)
->retry(3, 100)
->get('https://api.example.com/users');
This will retry the request up to 3 times, with a 100ms delay between attempts.
Error Handling
Laravel's HTTP client provides methods to handle different response scenarios:
$response = Http::get('https://api.example.com/users');
if ($response->successful()) {
// Status code in the range 200-299
}
if ($response->failed()) {
// Status code not in the range 200-299
}
$response->throw(); // Throw an exception if a client or server error occurred
Concurrent Requests
For multiple concurrent requests:
$responses = Http::pool(fn ($pool) => [
$pool->get('https://api.example.com/users'),
$pool->get('https://api.example.com/posts'),
$pool->get('https://api.example.com/comments'),
]);
Using Macros
Macros allow you to define reusable request patterns:
Http::macro('github', function () {
return Http::withHeaders([
'Authorization' => 'token ' . config('services.github.token'),
])->baseUrl('https://api.github.com');
});
$response = Http::github()->get('/users/laravel');
Testing
Laravel's HTTP client integrates seamlessly with testing:
Http::fake([
'github.com/*' => Http::response(['name' => 'Taylor'], 200),
]);
$response = Http::get('https://github.com/users/taylor');
$response->assertStatus(200);
$response->assertJson(['name' => 'Taylor']);
Middleware for Requests
You can even add middleware to your HTTP requests:
use Illuminate\Http\Client\PendingRequest;
Http::middleware(function (PendingRequest $request, Closure $next) {
$request->withHeaders([
'X-Example' => 'Value',
]);
return $next($request);
})->get('https://example.com');
Guzzle Options
For advanced scenarios, you can pass Guzzle options:
$response = Http::withOptions([
'debug' => true,
])->get('https://api.example.com/users');
Laravel's HTTP client provides a powerful, expressive way to interact with APIs. By leveraging its features, you can write cleaner, more maintainable code for your API interactions. Whether you're building a complex API integration or simply need to make a few HTTP requests, the HTTP client offers the flexibility and ease of use to meet your needs.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter. It helps a lot!