Simplify Real-Time Notifications with Laravel's Anonymous Broadcasts
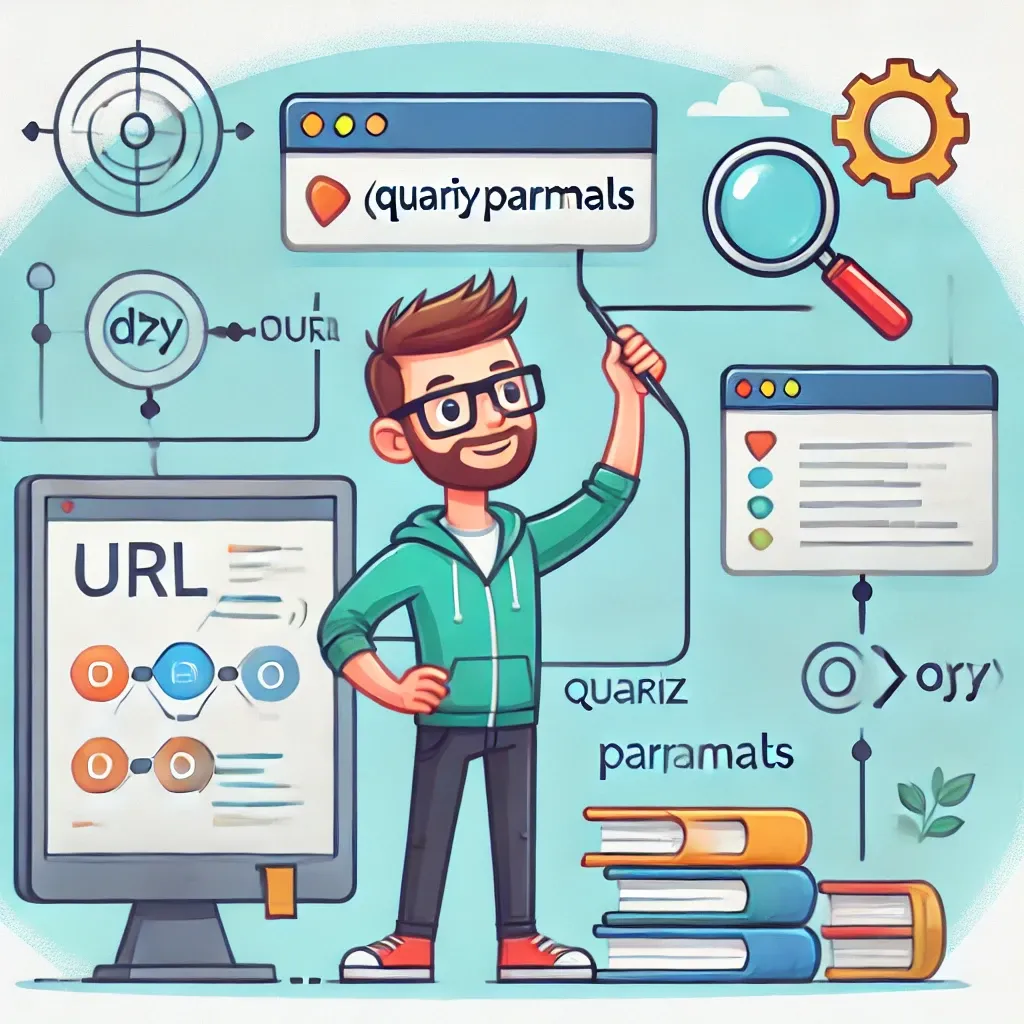
Laravel's real-time capabilities just got even more accessible with anonymous broadcasts - a lightweight solution for sending ad-hoc notifications to your frontend.
Sometimes you need to notify your frontend about an event without creating a dedicated event class. Anonymous broadcasts solve this problem by providing a simple API to send real-time updates directly.
Let's see how it works:
// Send a basic anonymous broadcast
Broadcast::on('my-channel')->send();
Using Anonymous Broadcasts
By default, this broadcasts an "AnonymousEvent" with empty data. You can customize both using the as
and with
methods:
// Custom event name and data
Broadcast::on('orders.'.$order->id)
->as('OrderPlaced')
->with(['id' => $order->id, 'total' => $order->total])
->send();
For private or presence channels, use the dedicated methods:
// Private channel broadcast
Broadcast::private('user.'.$userId)->send();
// Presence channel broadcast
Broadcast::presence('team-chat')->send();
If you need immediate broadcasting instead of queuing, use sendNow
:
Broadcast::on('notifications')->sendNow();
To exclude the current user from receiving the broadcast:
Broadcast::on('chat')->toOthers()->send();
On the frontend, listen for these events with Laravel Echo:
Echo.channel('orders.' + orderId)
.listen('.OrderPlaced', (data) => {
showNotification('Order placed!', data);
});
When to Use Anonymous Broadcasts
Anonymous broadcasts are perfect for:
- Simple notifications without complex data
- Quick status updates
- UI refresh triggers
- Temporary notifications that don't need permanent storage
- Chat features like "user is typing" indicators
By eliminating the need to create formal event classes, anonymous broadcasts make adding real-time features to your application faster and more straightforward.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter (https://x.com/harrisrafto), Bluesky (https://bsky.app/profile/harrisrafto.eu), and YouTube (https://www.youtube.com/@harrisrafto). It helps a lot!